CakePHP and Angular: Building Dynamic Web Applications
In the realm of modern web development, creating dynamic and interactive web applications is no longer just a preference, but a necessity. Users expect seamless and engaging experiences that go beyond static content. This is where the combination of CakePHP and Angular comes into play. By leveraging the capabilities of CakePHP on the backend and Angular on the frontend, developers can create feature-rich, dynamic web applications that cater to user demands and expectations.
Table of Contents
1. Introduction to CakePHP and Angular
1.1. The Power of Backend-frontend Collaboration:
In the world of web development, separating the frontend and backend has become a common practice. This separation allows developers to focus on their respective strengths while collaborating seamlessly to build powerful web applications. CakePHP, a popular PHP framework known for its simplicity and elegance, serves as an excellent choice for creating the backend logic of web applications. Angular, on the other hand, is a widely used TypeScript-based frontend framework that enables the creation of dynamic and interactive user interfaces.
1.2. Brief Overview of CakePHP:
CakePHP follows the convention over configuration (CoC) and model-view-controller (MVC) principles, which streamline development by providing predefined structures and patterns. It offers features like scaffolding, data validation, and code generation, reducing the time needed to build complex applications. CakePHP’s ORM (Object-Relational Mapping) simplifies database interactions and data manipulation.
1.3. Brief Overview of Angular:
Angular, maintained by Google, is renowned for building dynamic single-page applications (SPAs). It employs a component-based architecture, allowing developers to create reusable UI components. Angular’s two-way data binding and dependency injection enhance productivity and enable real-time updates without manual DOM manipulation.
2. Setting Up the Development Environment
2.1. Installing CakePHP:
To begin, make sure you have PHP and Composer installed. Use Composer to install CakePHP by running the following command:
bash composer create-project --prefer-dist cakephp/app myapp
2.2. Creating a Basic CakePHP Project:
Once the installation is complete, navigate to the project directory and use CakePHP’s built-in server to start the development server:
bash cd myapp bin/cake server
2.3. Installing Angular CLI:
To work with Angular, you’ll need Node.js and npm installed. Install Angular CLI globally using npm:
bash npm install -g @angular/cli
2.4. Setting Up an Angular Project:
Create a new Angular project by running the following command:
bash ng new angular-app
2.5. Navigate to the project directory:
bash cd angular-app
2.6. Start the Angular development server:
bash ng serve
3. Building the Backend with CakePHP
3.1. Creating Models, Views, and Controllers:
CakePHP’s CLI tool helps generate MVC components efficiently. Use the following commands to generate a controller, model, and template:
bash bin/cake bake controller Articles bin/cake bake model Article
3.2. Implementing RESTful APIs:
CakePHP simplifies API creation. Define routes and actions in your controller to handle HTTP requests:
php // config/routes.php $routes->setRouteClass(DashedRoute::class); $routes->scope('/api', function (RouteBuilder $builder) { $builder->setExtensions(['json']); // Enable JSON responses $builder->resources('Articles'); });
3.3. Handle CRUD operations in the controller:
php // src/Controller/ArticlesController.php public function index() { $articles = $this->Articles->find('all'); $this->set([ 'articles' => $articles, '_serialize' => ['articles'], ]); }
3.4. Handling Data and Database Interaction:
CakePHP’s ORM simplifies database interactions. Define database credentials in config/app_local.php and use the ORM to query the database:
php // src/Controller/ArticlesController.php public function index() { $articles = $this->Articles->find('all'); $this->set([ 'articles' => $articles, '_serialize' => ['articles'], ]); }
4. Crafting the Frontend with Angular
4.1. Creating Components, Services, and Modules:
Angular’s component-based structure encourages the creation of reusable UI elements. Generate components using the Angular CLI:
bash ng generate component article-list
This generates the necessary files for an Angular component. Services can be generated in a similar manner:
bash ng generate service article
4.2. Implementing Reactive UI Components:
Angular leverages reactive programming with Observables. Fetch data from the backend using Angular’s HttpClient module and subscribe to the response:
typescript // src/app/article-list/article-list.component.ts import { Component, OnInit } from '@angular/core'; import { ArticleService } from '../article.service'; @Component({ selector: 'app-article-list', templateUrl: './article-list.component.html', styleUrls: ['./article-list.component.css'], }) export class ArticleListComponent implements OnInit { articles: any[] = []; constructor(private articleService: ArticleService) {} ngOnInit(): void { this.articleService.getArticles().subscribe((data) => { this.articles = data; }); } }
4.3. Managing HTTP Requests to Backend:
Create an Angular service to handle HTTP requests:
typescript // src/app/article.service.ts import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root', }) export class ArticleService { private baseUrl = 'http://localhost:8765/api/articles'; constructor(private http: HttpClient) {} getArticles(): Observable<any[]> { return this.http.get<any[]>(this.baseUrl); } }
5. Integration: Making CakePHP and Angular Work Together
5.1. CORS Configuration for Cross-Origin Requests:
To allow cross-origin requests between CakePHP and Angular, configure CORS settings in your CakePHP application. Install the cakephp/cakephp-cors plugin:
bash composer require cakephp/cakephp-cors
5.2. Configure the plugin in config/bootstrap.php:
php // config/bootstrap.php Plugin::load('Cors', ['bootstrap' => true, 'routes' => true]);
5.3. Enable CORS for your CakePHP application by modifying config/cors.php:
php // config/cors.php return [ 'Cors' => [ 'allowOrigin' => ['*'], 'allowMethods' => ['GET', 'POST', 'PUT', 'DELETE'], // ... ], ];
5.4. Consuming RESTful APIs from Angular:
Angular’s HttpClient automatically handles CORS. You can directly call the API endpoint from your Angular service as shown earlier.
5.5. Displaying Dynamic Data in Angular Components:
Integrate the Angular component created earlier with the data fetched from the CakePHP API:
html <!-- src/app/article-list/article-list.component.html --> <div *ngFor="let article of articles"> <h2>{{ article.title }}</h2> <p>{{ article.body }}</p> </div>
6. Creating an Interactive User Experience
6.1. Implementing User Authentication and Authorization:
For user authentication, you can use CakePHP’s built-in authentication component. Configure authentication in src/Application.php:
php // src/Application.php $this->addPlugin('Authentication');
6.2. Utilizing Angular Forms for User Input:
Angular’s reactive forms make capturing and validating user input easy. Create a form to add new articles:
typescript // src/app/article-form/article-form.component.ts import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { ArticleService } from '../article.service'; @Component({ selector: 'app-article-form', templateUrl: './article-form.component.html', styleUrls: ['./article-form.component.css'], }) export class ArticleFormComponent implements OnInit { articleForm: FormGroup; constructor(private fb: FormBuilder, private articleService: ArticleService) { this.articleForm = this.fb.group({ title: ['', Validators.required], body: ['', Validators.required], }); } onSubmit(): void { if (this.articleForm.valid) { const newArticle = this.articleForm.value; this.articleService.addArticle(newArticle).subscribe(() => { // Refresh the list of articles }); } } }
6.3. Real-time Updates with WebSockets:
To implement real-time updates, you can integrate WebSockets using libraries like socket.io. Angular can communicate with the backend to receive instant updates without continuous polling.
7. Optimizing Performance and Security
7.1. Caching Strategies for CakePHP:
CakePHP offers caching mechanisms to enhance performance. Implement caching for frequently accessed data to reduce database queries.
7.2. Security Best Practices in Angular:
Angular provides security features like content security policies, sanitization, and built-in protections against common web vulnerabilities. Ensure proper validation and sanitization of user input to prevent cross-site scripting (XSS) attacks.
8. Deployment and Beyond
8.1. Building and Deploying Angular Applications:
To build the Angular application for production, use the following command:
bash ng build --prod
This generates optimized files in the dist directory, which can be deployed to a web server.
8.2. Deploying CakePHP Applications:
For CakePHP, deploy your application using standard PHP hosting methods. Ensure the server meets the requirements of your CakePHP version.
9. Case Study: Building a Dynamic E-commerce Platform
9.1. Backend Setup for Product Management:
Utilize CakePHP to manage products, categories, and user accounts. Implement APIs for listing products and handling orders.
9.2. Frontend Implementation of Shopping Cart:
Create an Angular shopping cart component to manage product selections, quantities, and total prices. Integrate with the CakePHP backend to process orders.
9.3. User Authentication and Order Tracking:
Implement user registration, login, and authentication in CakePHP. Enable users to track their orders using Angular components that consume CakePHP APIs.
Conclusion
The Synergy of CakePHP and Angular:
Combining the strengths of CakePHP and Angular empowers developers to create dynamic, feature-rich web applications. CakePHP’s robust backend capabilities and Angular’s interactive frontend components complement each other, resulting in seamless user experiences.
Empowering the Next Generation of Web Applications:
CakePHP and Angular exemplify the potential of collaboration between backend and frontend technologies. As user expectations continue to evolve, harnessing the power of these frameworks will be pivotal in building the next generation of dynamic web applications that captivate and engage users like never before.
Table of Contents
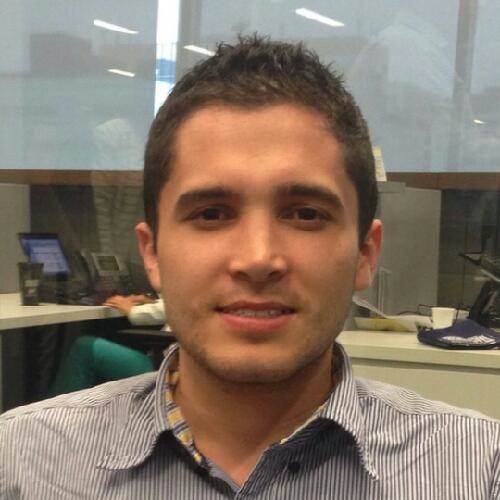
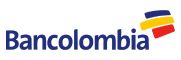