Working with CakePHP’s Email Component: Sending Emails
Sending emails is a fundamental aspect of modern web applications. Whether it’s for user registration, password resets, or sending newsletters, the ability to communicate with users via email is crucial. CakePHP, a popular PHP framework, simplifies this process with its Email Component. In this comprehensive guide, we’ll explore how to effectively use CakePHP’s Email Component to send emails in your web applications.
Table of Contents
1. Introduction to CakePHP’s Email Component
CakePHP is known for its rapid development capabilities and a wide range of built-in features that streamline web application development. The Email Component is one such feature that makes sending emails a breeze. It abstracts away many of the complexities involved in email delivery, allowing developers to focus on their application’s functionality.
1.1. Setting Up CakePHP
Before we dive into using the Email Component, make sure you have a CakePHP project set up. If you haven’t done this yet, follow these steps:
- Install CakePHP: You can use Composer to create a new CakePHP project.
bash composer create-project --prefer-dist cakephp/app myapp
2. Configure Your Database: Update your config/app.php file to configure your database connection settings.
3. Create a Controller and View: Create a controller and view that you want to use to trigger email sending.
1.2. Configuring Email Settings
To send emails using CakePHP, you need to configure your email settings in the config/app.php file. CakePHP supports various email transports, including SMTP, Sendmail, and more. Here’s an example configuration for using SMTP:
php // config/app.php 'EmailTransport' => [ 'default' => [ 'className' => 'Smtp', 'host' => 'smtp.example.com', 'port' => 587, 'timeout' => 30, 'username' => 'your_username', 'password' => 'your_password', 'tls' => true, ], ],
Make sure to replace ‘smtp.example.com’, ‘your_username’, and ‘your_password’ with your actual SMTP server details.
2. Sending Basic Emails
Now that you have your CakePHP project set up and email settings configured, let’s start sending some basic emails.
2.1. Using the Email Class
CakePHP provides an Email class for sending emails. In your controller, you can load this class using:
php // src/Controller/YourController.php use Cake\Mailer\Email;
Now, you can use the Email class to send emails within your controller’s actions:
php // src/Controller/YourController.php public function sendEmail() { $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Hello, CakePHP!') ->send('This is a basic email sent using CakePHP.'); }
In the code above, we create a new Email instance, set the recipient’s email address, subject, and body of the email, and then send it. The ‘default’ argument in new Email(‘default’) refers to the email transport configuration we defined earlier.
2.2. Sending HTML Emails
CakePHP makes it easy to send HTML emails by using the setEmailFormat(‘html’) method:
php // src/Controller/YourController.php public function sendHtmlEmail() { $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Hello, CakePHP HTML!') ->setEmailFormat('html') ->send('<p>This is an HTML email sent using CakePHP.</p>'); }
By setting the email format to ‘html’, CakePHP will send the email as HTML, allowing you to include rich content, such as images and links.
3. Email Templates
Sending basic emails as shown above is suitable for simple use cases. However, for more complex emails, it’s advisable to use email templates. CakePHP supports both plain text and HTML templates.
3.1. Creating Email Templates
To create an email template, navigate to the src/Template/Email directory in your CakePHP project. You can create a new folder for your email templates if needed. Inside this directory, create a new file for your email template with the .ctp file extension. For example, welcome.ctp for a welcome email template.
In your email template file, you can use plain HTML and CakePHP’s template variables to customize the content of the email:
html <!-- src/Template/Email/welcome.ctp --> <html> <head></head> <body> <p>Dear <?= $name ?>,</p> <p>Welcome to our website! We're excited to have you on board.</p> </body> </html>
3.2. Using Email Templates
To use an email template in your controller, you can load it using the setViewVars() method and then pass any necessary variables:
php // src/Controller/YourController.php public function sendWelcomeEmail() { $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Welcome to Our Website') ->setEmailFormat('html') ->setViewVars(['name' => 'John Doe']) // Pass template variables ->viewBuilder() ->setTemplate('welcome') // Use the 'welcome.ctp' template ->setLayout('default'); // Set the layout (default is 'default') $email->send(); }
In this example, we pass the variable ‘name’ to the email template and specify which template to use using ->setTemplate(‘welcome’). You can also specify the layout using ->setLayout(‘default’).
4. Adding Attachments
Sometimes, you may need to attach files, such as PDFs or images, to your emails. CakePHP makes this task straightforward.
4.1. Attaching Files
To attach a file to your email, use the attachments() method:
php // src/Controller/YourController.php public function sendEmailWithAttachment() { $email = new Email('default'); $email->setTo('recipient@example.com') ->setSubject('Email with Attachment') ->setEmailFormat('html') ->setAttachments([ 'file.pdf' => [ 'file' => 'path/to/file.pdf', 'mimetype' => 'application/pdf', ], ]) ->send('Check out this PDF file!'); }
In this example, we attach a PDF file named ‘file.pdf’ located at ‘path/to/file.pdf’. You can specify the file’s MIME type to ensure proper handling.
5. Sending Emails with Queues
For resource-intensive tasks like sending large batches of emails, it’s a good practice to use queues. CakePHP integrates seamlessly with popular queue systems like RabbitMQ, Beanstalk, and others.
5.1. Configuring Queues
First, make sure you have a queue system set up and configured in your CakePHP project. CakePHP uses the Queue plugin for this purpose. Install it via Composer:
bash composer require dereuromark/cakephp-queue
Next, configure the queue system settings in your config/app.php:
php // config/app.php 'Queue' => [ 'default' => [ 'className' => 'Queue', 'url' => env('QUEUE_URL', null), // Other queue-specific settings ], ],
5.2. Sending Emails with Queues
To send emails using a queue, you need to create a queue task. CakePHP provides a convenient command to generate a queue task:
bash bin/cake bake queue Email
This will generate a EmailQueueTask file in your src/Shell/Task directory. You can then modify this task to send emails using the Email class.
Here’s an example of how you can send an email with a queue task:
php // src/Shell/Task/EmailQueueTask.php use Cake\Mailer\Email; class EmailQueueTask extends QueueTask { public function run(array $data, $id) { $email = new Email('default'); $email->setTo($data['recipient']) ->setSubject($data['subject']) ->setEmailFormat('html') ->send($data['body']); } }
Now, you can enqueue email sending tasks using the Queue plugin, ensuring efficient email delivery.
Conclusion
CakePHP’s Email Component simplifies the process of sending emails in your web applications. From basic text emails to complex HTML templates and attachments, CakePHP provides the tools you need to create engaging email communication with your users.
By configuring email settings, using the Email class, leveraging templates, and even queuing emails for efficient delivery, you can ensure that your application’s email functionality is both robust and user-friendly.
Whether you’re building a user registration system, a notification system, or a newsletter feature, CakePHP’s Email Component will empower you to create compelling email experiences for your users with ease. Start harnessing the power of CakePHP’s Email Component today to enhance your web application’s communication capabilities.
In this comprehensive guide, we’ve covered the essential aspects of working with CakePHP’s Email Component. From setting up CakePHP, configuring email settings, sending basic and HTML emails, using email templates, adding attachments, to sending emails with queues, you now have a solid foundation to incorporate email functionality seamlessly into your CakePHP projects. Happy coding!
Table of Contents
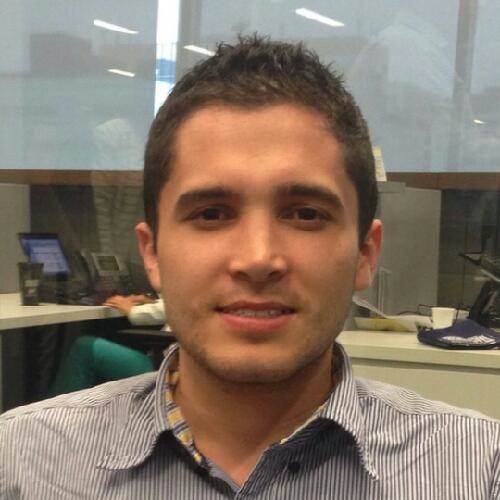
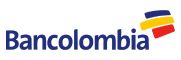