CakePHP Error Handling and Logging: Debugging Made Easy
CakePHP, a powerful PHP framework, is known for its developer-friendly approach. One of the areas where CakePHP shines is error handling and logging, making debugging a seamless process. As a developer, you know how crucial it is to have effective tools to identify and resolve issues quickly. In this blog, we’ll dive into the world of CakePHP error handling and logging, exploring its essential features, best practices, and how it simplifies debugging.
1. Understanding CakePHP Error Handling
Error handling in CakePHP is based on exceptions, which are a way of handling error conditions. When an error occurs, an exception is thrown, and if it is not caught, it will halt the application and display an error page or message. Let’s take a look at some key aspects of CakePHP error handling:
1.1. Exception Types
CakePHP provides various built-in exception classes that cover different types of errors:
- Cake\Core\Exception\Exception: The base class for all exceptions within CakePHP.
- Cake\Datasource\Exception\RecordNotFoundException: Thrown when a database record is not found.
- Cake\Network\Exception\NotFoundException: Used for 404 Not Found errors.
- Cake\Network\Exception\BadRequestException: Represents a 400 Bad Request error.
- Cake\Network\Exception\ForbiddenException: Thrown when a user does not have the required permissions.
- Cake\Network\Exception\MethodNotAllowedException: Represents a 405 Method Not Allowed error.
- Cake\Network\Exception\InternalErrorException: Used for 500 Internal Server errors.
Using specific exception classes allows you to handle different types of errors differently.
1.2. Error Configuration
The error configuration in CakePHP is handled in the config/app.php file. Here you can set the level of error reporting and customize error handling. The default error level is E_ALL & ~E_DEPRECATED & ~E_USER_DEPRECATED, but you can modify it based on your development environment.
php // config/app.php 'Error' => [ 'errorLevel' => E_ALL & ~E_DEPRECATED & ~E_USER_DEPRECATED, // Other error configurations ]
1.3. Custom Exception Handling
CakePHP allows you to define custom exception handlers to handle exceptions based on your application’s requirements. You can create custom exception classes or modify the existing ones to include additional information or error handling logic.
To set up custom exception handling, you need to modify the bootstrap.php file and use the Cake\Error\ExceptionRenderer class.
php // config/bootstrap.php use App\Error\AppExceptionRenderer; Configure::write('Error.exceptionRenderer', AppExceptionRenderer::class);
2. Logging in CakePHP
Effective logging is essential for understanding application behavior and tracking down issues. CakePHP provides a built-in logging system that allows you to record information about various events. Let’s explore CakePHP logging and how it can help you with debugging:
2.1. Logging Configuration
Similar to error handling, the logging configuration is also managed in the config/app.php file. You can set the logging levels, loggers, and their configurations.
php // config/app.php 'Log' => [ 'error' => [ 'className' => 'Cake\Log\Engine\FileLog', 'path' => LOGS, 'levels' => ['error', 'warning'], 'file' => 'error', ], // Other loggers ]
In the above example, we have set up a file logger to log error and warning level messages. The log files will be stored in the logs/ directory.
2.2. Logging Levels
CakePHP supports several logging levels, each representing a different severity of messages:
- debug: Used for detailed debug information.
- info: General information about the application’s operation.
- notice: Used for less critical conditions.
- warning: Indicates potential issues that should be addressed.
- error: Represents errors that need attention.
- critical: Critical conditions that require immediate action.
- alert: Alerts that require immediate attention.
- emergency: Used for emergency situations.
You can choose the appropriate logging level based on the severity of the message you want to log.
2.3. Logging Messages
To log messages in CakePHP, you can use the Log class, which provides static methods for each logging level. Here’s an example:
php use Cake\Log\Log; Log::info('User login successful.', ['user_id' => 123]);
In this example, we log an informational message about a successful user login and include additional data such as the user ID.
2.4. Logging Context
Contextual information is crucial for understanding log messages fully. CakePHP allows you to include context data when logging messages, which provides additional insights into the logged events.
php use Cake\Log\Log; $orderId = 456; Log::warning('Order not processed.', ['order_id' => $orderId]);
By including the $orderId in the log context, you can easily trace the related log entries.
3. Error Handling and Logging Best Practices
To make the most out of CakePHP error handling and logging capabilities, follow these best practices:
3.1. Use Specific Exception Types
When throwing exceptions, use specific exception types whenever possible. This allows you to handle different errors separately and provide more informative error messages to users.
php use Cake\Network\Exception\NotFoundException; if (!$user) { throw new NotFoundException('User not found.'); }
3.2. Log Meaningful Messages
When logging messages, ensure that the log entries provide meaningful information. Include relevant data and context to help identify the root cause of issues.
php use Cake\Log\Log; Log::error('Database connection failed.', ['error' => $e->getMessage()]);
Including the error message in the log entry gives valuable insights into the problem.
3.3. Utilize Log Levels Wisely
Choose the appropriate logging level for each log message. Use higher log levels like emergency and alert sparingly, reserving them for critical situations.
php use Cake\Log\Log; Log::emergency('Server is down!');
Reserve the emergency log level for truly urgent issues that require immediate attention.
3.4. Regularly Monitor Logs
Make it a habit to monitor your application’s logs regularly. Logs can provide valuable information about application behavior, user activity, and potential issues.
3.5. Enable Debug Mode in Development
In the development environment, enable CakePHP’s debug mode to display detailed error messages and stack traces. However, remember to disable debug mode in production to avoid exposing sensitive information to users.
php // config/app.php 'debug' => env('DEBUG', true),
Conclusion
CakePHP’s error handling and logging features provide a robust foundation for smooth development and efficient debugging. By leveraging custom exception handling, setting appropriate logging levels, and incorporating meaningful log messages, you can streamline the debugging process. Following best practices and regularly monitoring logs will help you maintain a stable and error-free CakePHP application. So, next time you encounter an issue, dive into the logs and make debugging easy with CakePHP! Happy coding!
Table of Contents
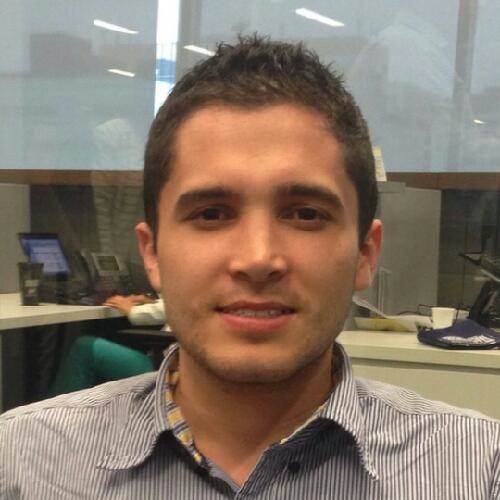
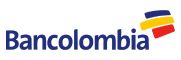