CakePHP Error Tracking and Monitoring: Tools and Techniques
CakePHP is a popular and powerful PHP framework known for its simplicity, speed, and flexibility. Developing web applications with CakePHP can be a breeze, but like any software project, it’s not immune to errors and bugs. To ensure your CakePHP application runs smoothly and stays error-free, you need robust error tracking and monitoring tools and techniques. In this blog post, we’ll explore some essential tools and techniques for effective CakePHP error tracking and monitoring, helping you maintain the reliability and performance of your CakePHP applications.
Table of Contents
1. Why Error Tracking and Monitoring Are Crucial
Before diving into the tools and techniques, let’s understand why error tracking and monitoring are crucial for CakePHP applications:
1.1. Ensuring Application Reliability
CakePHP applications often serve critical functions, such as e-commerce platforms or content management systems. An error or bug can disrupt these functions, leading to a poor user experience or financial losses. Error tracking helps you catch and fix issues before they impact your users.
1.2. Identifying Performance Bottlenecks
Monitoring tools not only help find errors but also uncover performance bottlenecks. Slow database queries, high CPU usage, or memory leaks can severely affect your CakePHP application’s performance. Monitoring allows you to pinpoint these issues and optimize your code accordingly.
1.3. Maintaining Data Security
Security vulnerabilities in web applications are a serious concern. An unnoticed error might expose sensitive user data or make your application susceptible to attacks. Proper error tracking helps you detect and patch security vulnerabilities promptly.
2. Essential Error Tracking Tools and Techniques
Let’s explore some of the essential tools and techniques for effective CakePHP error tracking:
2.1. CakePHP’s Built-in Error Handling
CakePHP provides robust error handling capabilities out of the box. You can configure how errors are logged, displayed, or even sent as email notifications. Here’s how you can configure error handling in CakePHP:
php // In config/app.php 'Error' => [ 'errorLevel' => E_ALL, 'exceptionRenderer' => 'Cake\Error\ExceptionRenderer', 'skipLog' => [], 'log' => true, 'trace' => true, ]
By customizing these settings, you can control how CakePHP handles errors and exceptions.
2.2. Logging Errors with Monolog
Monolog is a popular PHP logging library that can be integrated with CakePHP to enhance error tracking. It allows you to log errors, warnings, and other messages to various outputs, such as files, databases, or external services. To use Monolog in CakePHP, first, install it via Composer:
bash composer require monolog/monolog
Next, configure Monolog in your CakePHP application:
php // In config/bootstrap.php use Monolog\Logger; use Monolog\Handler\StreamHandler; $logger = new Logger('my_logger'); $logger->pushHandler(new StreamHandler(LOGS . 'error.log', Logger::ERROR)); Log::setConfig('error', [ 'className' => 'Cake\Log\Engine\FileLog', 'path' => LOGS, 'file' => 'error', 'logLevels' => ['error', 'warning'], ]);
Now, CakePHP will use Monolog to log errors to a file named error.log.
2.3. Exception Tracking with Sentry
Sentry is a powerful error and exception tracking tool that provides real-time error alerts and detailed error reports. Integrating Sentry with CakePHP can help you proactively identify and resolve issues. To get started, sign up for a Sentry account and install the Sentry SDK:
bash composer require sentry/sentry
Configure Sentry in your CakePHP application:
php // In config/bootstrap.php use Sentry\SentrySdk; SentrySdk::init([ 'dsn' => 'YOUR_DSN_HERE', // Other configuration options ]);
Replace ‘YOUR_DSN_HERE’ with your actual Sentry DSN (Data Source Name).
With Sentry, you can monitor your application’s errors in real-time, receive notifications, and access detailed error reports to expedite debugging.
3. Effective Monitoring Techniques
In addition to error tracking, effective monitoring techniques can help you identify performance issues and maintain the health of your CakePHP application:
3.1. Application Performance Monitoring (APM)
APM tools like New Relic or AppDynamics offer comprehensive insights into your application’s performance. They can monitor database queries, HTTP requests, and code execution times. Integrating APM tools with CakePHP can help you identify bottlenecks and optimize your code for better performance.
3.2. Database Query Profiling
CakePHP provides built-in tools for profiling database queries. You can enable query logging and analyze the generated SQL queries to identify slow or inefficient queries. Here’s how to enable query logging:
php // In your controller's action $this->loadModel('YourModel'); $query = $this->YourModel->find(); $query->enableHydration(false)->toArray(); debug($query->sql());
This code will display the generated SQL query, allowing you to optimize it if necessary.
3.3. Server and Infrastructure Monitoring
Monitoring your server and infrastructure is crucial for identifying hardware-related issues. Tools like Nagios, Zabbix, or Prometheus can help you monitor server metrics like CPU usage, memory usage, and disk space. By keeping an eye on these metrics, you can prevent server failures and downtime.
4. Best Practices for Error Handling
To make the most of error tracking and monitoring, follow these best practices:
4.1. Use Descriptive Error Messages
When an error occurs, ensure that the error message provides enough information to understand the issue. Include details like the error type, the location in the code, and any relevant variables or inputs.
4.2. Implement Error Codes
Consider using error codes to standardize error reporting in your CakePHP application. This makes it easier to categorize and prioritize errors and helps developers quickly identify the root causes.
4.3. Regularly Review Error Logs
Set up a routine to review error logs and reports. Regularly check your monitoring dashboard to catch and address issues promptly. Establishing this practice can prevent errors from piling up and becoming overwhelming.
4.4. Automate Error Notifications
Configure your error tracking and monitoring tools to send automated notifications when critical errors occur. This ensures that your development team is alerted in real-time and can take immediate action.
Conclusion
Error tracking and monitoring are indispensable for maintaining the reliability, performance, and security of your CakePHP applications. By leveraging built-in features, third-party tools, and best practices, you can ensure that your CakePHP projects run smoothly and deliver a seamless user experience. Don’t wait for errors to pile up; start implementing these tools and techniques today to keep your CakePHP applications error-free and performant.
Table of Contents
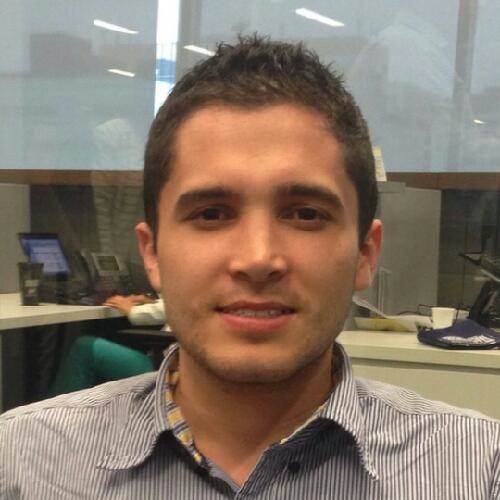
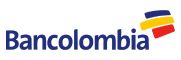