Understanding CakePHP Events and Event Listeners
Modern web applications are becoming increasingly complex, with numerous components interacting to deliver seamless user experiences. In such intricate systems, maintaining clear communication and coordination between different parts of your application is essential. This is where the concept of events and event listeners comes into play. In the context of CakePHP, these tools provide an elegant and efficient way to manage interactions between different components of your application, making your code more organized, modular, and easier to maintain.
Table of Contents
1. The Essence of CakePHP Events
In the world of software development, events are occurrences that signify something of importance within an application’s lifecycle. These occurrences can range from a user registering on a website to a critical error being encountered. CakePHP recognizes the significance of such events and provides a built-in mechanism to handle them effectively.
2. Benefits of Using Events
Using events and event listeners in CakePHP offers a plethora of advantages:
- Decoupling of Components: One of the primary benefits is decoupling. Components of your application can communicate without knowing about each other’s implementation details. This promotes modularity, making your codebase more maintainable and adaptable.
- Flexibility: Events provide a flexible way to extend and modify the behavior of your application without altering existing code. You can introduce new functionalities or modify existing ones by simply adding or changing event listeners.
- Organized Workflow: By segregating various functionalities into events and listeners, you create a well-organized workflow. This not only enhances readability but also facilitates collaboration among developers working on different parts of the application.
- Reusability: Event listeners are reusable components. Once defined, they can be used across different parts of your application, reducing redundancy and promoting a DRY (Don’t Repeat Yourself) approach.
3. Anatomy of CakePHP Events and Event Listeners
To grasp the concept of CakePHP events and event listeners, let’s break down their individual components:
3.1. Events
Events, in CakePHP, are representations of notable occurrences within your application. They are identified by a unique name and can carry data that needs to be communicated to event listeners. Think of events as signals that something has happened and needs attention.
3.2. Event Listeners
Event listeners are the observers of events. They are responsible for performing specific actions when an event they are associated with is triggered. Event listeners encapsulate the logic required to respond to events without altering the code that fires the events. This separation of concerns is what makes CakePHP events and event listeners so powerful.
4. Implementing Events and Event Listeners in CakePHP
Let’s dive into the practical aspect of using events and event listeners in CakePHP. Assume you’re working on an e-commerce platform and want to implement a notification system for order updates.
Step 1: Defining an Event
First, you need to define an event that will be triggered when an order is updated:
php // In config/bootstrap.php use Cake\Event\EventManager; EventManager::instance()->on('Model.Order.updated', function ($event, $order) { // Logic for sending notifications });
Step 2: Triggering the Event
Next, you trigger the event whenever an order is updated:
php // In your Order model's update method $this->getEventManager()->dispatch(new Event('Model.Order.updated', $this, ['order' => $orderData]));
Step 3: Creating an Event Listener
Now, create an event listener that responds to the ‘Model.Order.updated’ event:
php // Create a new file: src/Event/OrderEventListener.php namespace App\Event; use Cake\Event\EventListenerInterface; class OrderEventListener implements EventListenerInterface { public function implementedEvents(): array { return [ 'Model.Order.updated' => 'sendNotifications', ]; } public function sendNotifications($event, $order) { // Notification sending logic } }
Step 4: Registering the Event Listener
Finally, register the event listener in your application:
php // In src/Application.php use Cake\Event\EventManager; EventManager::instance()->on(new OrderEventListener());
With these steps, your CakePHP application now has a functional event and event listener setup. Whenever an order is updated, the ‘Model.Order.updated’ event is triggered, and the associated event listener, OrderEventListener, sends out notifications.
Conclusion
CakePHP events and event listeners are crucial tools that streamline communication between different parts of your application. They promote modularity, flexibility, and a more organized workflow. By decoupling components and encapsulating logic, your code becomes more maintainable and adaptable to changes. Whether you’re building a small website or a complex web application, understanding and utilizing CakePHP events and event listeners will undoubtedly enhance the overall quality of your codebase. So, the next time you’re faced with the challenge of orchestrating interactions within your application, consider harnessing the power of events and event listeners offered by CakePHP. Your future self and fellow developers will thank you for the organized and efficient code you create.
Table of Contents
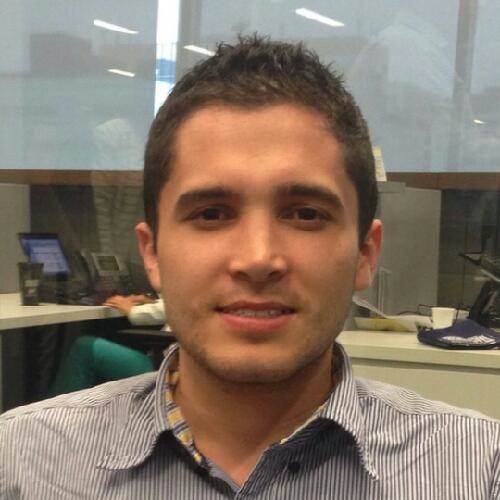
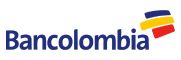