Implementing Full-Text Search in CakePHP with Elasticsearch
In today’s fast-paced digital world, providing users with a seamless and efficient search experience is crucial for any web application. Conventional database queries might fall short when it comes to handling complex search requirements. That’s where Elasticsearch comes into play. In this tutorial, we’ll explore how to integrate Elasticsearch with CakePHP to implement a robust full-text search feature, enabling your application to deliver lightning-fast and accurate search results.
Table of Contents
1. Why Elasticsearch?
Elasticsearch is an open-source, distributed search and analytics engine built on top of the Lucene library. It’s designed to handle large volumes of data and deliver near-real-time search capabilities. Elasticsearch’s advanced features like full-text search, faceted navigation, and scalability make it an ideal choice for applications that demand fast and complex search functionality.
2. Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites set up:
- CakePHP Project: Have a CakePHP project up and running.
- Elasticsearch: Install and configure Elasticsearch on your server. You can follow the official Elasticsearch documentation for installation instructions.
- Elasticsearch-PHP: Install the Elasticsearch-PHP library, which provides a convenient API for interacting with Elasticsearch from PHP applications. You can install it using Composer:
bash composer require elasticsearch/elasticsearch
3. Setting Up Elasticsearch Configuration
To begin, let’s configure CakePHP to communicate with the Elasticsearch server. Open config/app.php and add the following Elasticsearch configuration to the Datasources section:
php 'Datasources' => [ 'default' => [ // ... other configurations 'elasticsearch' => [ 'host' => 'localhost', // Elasticsearch host 'port' => 9200, // Elasticsearch port ], ], ],
4. Creating the Search Index
In Elasticsearch, data is organized into indices, which are analogous to databases in traditional SQL databases. Each index can contain multiple documents, and each document represents a searchable entity. For our CakePHP application, let’s assume we want to implement a full-text search for blog posts.
- Create Index: We’ll create an index named blog_posts to store our blog post data. You can create the index using the following command:
php bin/cake elastic createIndex blog_posts
2. Define Mapping: Elasticsearch requires a mapping to understand the structure of your documents. Create a file named blog_posts.php in the config/Elasticsearch/Indices directory of your CakePHP project. Define the mapping for your blog posts:
php // config/Elasticsearch/Indices/blog_posts.php return [ 'mappings' => [ 'properties' => [ 'title' => ['type' => 'text'], 'content' => ['type' => 'text'], // ... other fields ], ], ];
3. Apply Mapping: Apply the mapping to the blog_posts index:
php bin/cake elastic applyMapping blog_posts
5. Indexing Data
Now that our index is set up, let’s start indexing our blog post data into Elasticsearch.
- Fetching Data: In your CakePHP application, retrieve the blog post data that you want to index.
- Indexing Data: Iterate through the fetched data and index it into Elasticsearch using the Elasticsearch-PHP library. Here’s a sample code snippet to help you get started:
php use Elasticsearch\ClientBuilder; $client = ClientBuilder::create() ->setHosts([Configure::read('Datasources.elasticsearch.host') . ':' . Configure::read('Datasources.elasticsearch.port')]) ->build(); foreach ($blogPosts as $post) { $params = [ 'index' => 'blog_posts', 'id' => $post->id, 'body' => [ 'title' => $post->title, 'content' => $post->content, // ... other fields ], ]; $client->index($params); }
6. Implementing Full-Text Search
With the data indexed, it’s time to implement the actual full-text search functionality.
- Search Endpoint: Create a search endpoint in your CakePHP application that will handle user search queries.
- Searching: In the search action of your controller, use the Elasticsearch-PHP library to perform the search. Here’s a basic example:
php use Elasticsearch\ClientBuilder; $client = ClientBuilder::create() ->setHosts([Configure::read('Datasources.elasticsearch.host') . ':' . Configure::read('Datasources.elasticsearch.port')]) ->build(); $searchQuery = [ 'query' => [ 'match' => [ 'content' => $userQuery, ], ], ]; $params = [ 'index' => 'blog_posts', 'body' => $searchQuery, ]; $response = $client->search($params); $searchResults = $response['hits']['hits'];
7. Displaying Search Results
With the search results in hand, you can now display them to the user in a visually appealing manner.
- Rendering Results: In your view, iterate through the $searchResults array and display the relevant information.
- Linking to Original Content: Ensure that each search result links back to the original blog post on your CakePHP application.
8. Enhancements and Further Customization
Implementing a basic full-text search functionality is just the tip of the iceberg. Elasticsearch offers a wide range of features that you can leverage to enhance your search capabilities:
- Highlighting: Highlight the matched search terms within the content.
- Autocomplete: Implement an autocomplete feature to provide instant suggestions while users type.
- Faceted Navigation: Allow users to filter search results based on categories, tags, or other attributes.
- Boosting: Adjust the relevance of search results based on specific criteria.
- Synonyms and Stemming: Improve search accuracy by handling synonyms and word variations.
Conclusion
Integrating Elasticsearch with your CakePHP application empowers you to deliver a lightning-fast and accurate search experience to your users. By following the steps outlined in this tutorial, you’ve learned how to set up Elasticsearch, create search indices, index data, and perform full-text searches. Remember that Elasticsearch offers a plethora of advanced features that can further elevate your search functionality, making it an indispensable tool for building modern and efficient web applications. So, go ahead and take your CakePHP application’s search capabilities to the next level with Elasticsearch!
Table of Contents
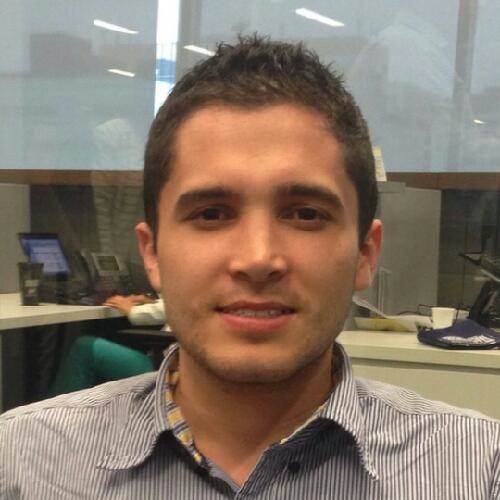
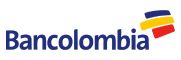