Implementing Geolocation Functionality in CakePHP
In today’s digital world, incorporating geolocation functionality into web applications has become crucial for enhancing user experiences and providing location-based services. CakePHP, a popular PHP framework known for its rapid development capabilities, offers a seamless way to integrate geolocation features into your application. In this guide, we’ll delve into the process of implementing geolocation functionality in CakePHP, covering everything from setting up geocoding services to displaying location-based data on your site.
Table of Contents
1. Understanding Geolocation and its Applications
Geolocation refers to the process of determining a user’s physical location using various data sources such as GPS, IP addresses, and Wi-Fi signals. The applications of geolocation are diverse, ranging from e-commerce platforms showing nearby stores to social media apps tagging posts with location information. By implementing geolocation in your CakePHP application, you can enhance user engagement and tailor services based on user location.
2. Setting Up Geocoding Services
Geocoding is the process of converting addresses into geographical coordinates (latitude and longitude). To get started with geolocation in CakePHP, you’ll need to choose a geocoding service. Google Maps Geocoding API and OpenStreetMap’s Nominatim API are popular options.
2.1. Obtaining API Key (Google Maps)
If you’re using the Google Maps Geocoding API, you’ll need an API key. Sign up for the Google Cloud Platform, create a new project, and enable the Geocoding API. Once you have the API key, you can integrate it into your CakePHP application’s configuration.
2.2. Configuring Geocoding Service
In your CakePHP project, navigate to the config directory and open app.php. Add the API key and configure the geocoding service settings:
php 'Geocoding' => [ 'GoogleMaps' => [ 'apiKey' => 'YOUR_GOOGLE_API_KEY', 'url' => 'https://maps.googleapis.com/maps/api/geocode/json' ] ]
3. Implementing Geolocation in CakePHP
Now that you have set up the foundation, it’s time to implement geolocation features in your CakePHP application.
3.1. Geocoding Addresses
Let’s say you have a user profile page, and you want to display the user’s location on a map. In your controller, retrieve the user’s address and use the Geocoding service to convert it into coordinates:
php public function viewProfile($user_id) { $user = $this->Users->get($user_id); $address = $user->address; $geocodingService = new GeocodingService(); $coordinates = $geocodingService->getCoordinates($address); $this->set(compact('user', 'coordinates')); }
Here, GeocodingService is a class you’ll create to interact with the geocoding service. It should handle making API requests and parsing responses.
3.2. Displaying the Map
In your profile view template, you can use JavaScript and a mapping library (e.g., Google Maps JavaScript API) to display the user’s location:
html <div id="map" style="width: 100%; height: 400px;"></div> <script> function initMap() { var map = new google.maps.Map(document.getElementById('map'), { center: {lat: <?php echo $coordinates['lat']; ?>, lng: <?php echo $coordinates['lng']; ?>}, zoom: 12 }); } </script> <script async defer src="https://maps.googleapis.com/maps/api/js?key=YOUR_GOOGLE_API_KEY&callback=initMap"></script>
Ensure that you replace YOUR_GOOGLE_API_KEY with your actual Google Maps API key.
4. Adding Location-Based Search
Another powerful use case for geolocation is implementing location-based searches. For instance, if you’re running a restaurant directory, users might want to find nearby restaurants.
4.1. Getting User’s Location
To provide location-based results, you need to retrieve the user’s location. This can be done using HTML5 Geolocation API:
javascript if ("geolocation" in navigator) { navigator.geolocation.getCurrentPosition(function(position) { var userLat = position.coords.latitude; var userLng = position.coords.longitude; // Pass userLat and userLng to the server for search }); }
4.2. Implementing the Search
In your CakePHP controller, receive the user’s location and perform a search query using the obtained coordinates:
php public function searchNearbyRestaurants() { $userLat = $this->request->getData('userLat'); $userLng = $this->request->getData('userLng'); $restaurants = $this->Restaurants->find('near', [ 'origin' => [$userLat, $userLng], 'limit' => 10 ]); $this->set(compact('restaurants')); }
Here, ‘near’ is a custom finder method you’ll need to implement in your RestaurantsTable class.
Conclusion
Integrating geolocation functionality into your CakePHP application opens up a world of possibilities for enhancing user experiences and delivering location-specific services. By setting up geocoding services, implementing location displays, and enabling location-based searches, you can create a more engaging and relevant platform for your users. CakePHP’s flexibility and ease of use make the process relatively straightforward, allowing you to focus on creating feature-rich geolocation-powered applications.
Remember, the examples provided in this guide are simplified to illustrate the core concepts. Depending on your application’s complexity, you might need to handle additional considerations such as error handling, caching, and user privacy. With a solid foundation in geolocation implementation, you’re well-equipped to take your CakePHP projects to the next level.
In this comprehensive guide, we explored the process of implementing geolocation functionality in CakePHP. From setting up geocoding services to displaying location-based data and enabling location-based searches, you now have the knowledge to enhance your CakePHP applications with powerful geolocation features. Whether you’re building a business directory, social network, or e-commerce platform, geolocation can add a new dimension of functionality and engagement for your users.
Table of Contents
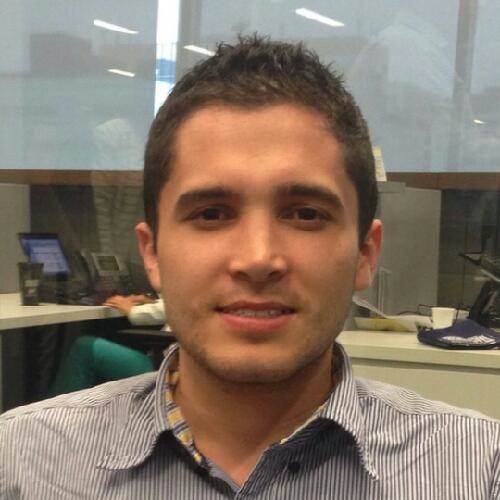
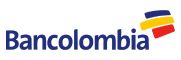