Implementing Versioning in CakePHP APIs
As software applications grow and evolve, so do their APIs. In order to maintain compatibility, provide a seamless user experience, and accommodate changes and improvements, versioning becomes a critical aspect of API development. In this article, we’ll explore how to implement versioning in CakePHP APIs effectively. We’ll cover the importance of versioning, strategies for versioning, and step-by-step guidelines, accompanied by code samples, to ensure a smooth transition for both developers and consumers.
Table of Contents
1. Why Versioning Matters
API versioning allows developers to make changes and updates to an API without disrupting existing clients or applications. It provides a structured way to handle changes and maintain backward compatibility. Without versioning, even a minor change in the API could lead to breaking changes for existing users, causing frustration and potential downtime for applications relying on the API.
Versioning also enables developers to release new features, enhancements, and bug fixes without impacting existing functionality. This is crucial for long-term maintainability and the ability to iterate on your API design over time.
2. Versioning Strategies
There are several strategies for implementing versioning in APIs, each with its own advantages and drawbacks. Let’s explore a few common strategies:
2.1. URL Path Versioning:
In this approach, the version is included as part of the URL path. For example:
bash https://api.example.com/v1/users
This strategy makes it explicit which version of the API you are using, but it can lead to longer URLs and potentially cluttered code.
2.2. Header Versioning:
Versioning can also be indicated through a custom header in the HTTP request. This keeps the URL clean and allows for better separation between the API endpoint and version information.
2.3. Media Type Versioning:
This approach involves using different media types (MIME types) for different versions of the API. For instance:
bash Accept: application/vnd.example.v1+json
For CakePHP APIs, URL path versioning is commonly used due to its simplicity and ease of implementation. In the following sections, we’ll focus on implementing URL path versioning in CakePHP.
3. Step-by-Step Implementation
Let’s dive into the step-by-step process of implementing versioning in CakePHP APIs using URL path versioning.
3.1. Set Up Your CakePHP Project
If you haven’t already, create a new CakePHP project or use an existing one as the basis for your API development.
3.2. Create Subfolders for Each Version
In your CakePHP project’s src/Controller directory, create subfolders for each API version. For example, create folders named v1 and v2.
3.3. Duplicate Controllers
Duplicate the necessary controllers from the main Controller directory into the version-specific subfolders. These duplicated controllers will handle version-specific logic while keeping the main controllers unchanged for backward compatibility.
3.4. Update Namespace and Routes
Update the namespace of the duplicated controllers to reflect the version. For example, if you have a UsersController in the src/Controller/v1 folder, update its namespace to:
php namespace App\Controller\v1;
Next, update your routes configuration (config/routes.php) to map the versioned URLs to the corresponding controllers:
php $builder->connect('/v1/users', ['controller' => 'v1/Users', 'action' => 'index']);
3.5. Implement Version-Specific Logic
In the duplicated controllers, you can now implement version-specific logic and changes. This could involve adding new endpoints, modifying existing ones, or updating response formats.
3.6. Handle Shared Logic
While version-specific controllers handle their respective logic, there might be shared logic that remains consistent across versions. You can keep this logic in the main controllers or create separate components to be used by all versions.
3.7. Documentation and Communication
Clearly document the API endpoints and their versions for developers and consumers. This documentation should detail the differences between versions, how to migrate from one version to another, and any deprecated endpoints.
4. Best Practices
To ensure a successful versioning strategy, consider these best practices:
- Semantic Versioning: Use a versioning scheme that follows semantic versioning (e.g., v1.0.0). This helps convey the nature of changes in each version.
- Long-Term Support: Provide long-term support for older versions to give clients ample time to transition.
- Deprecation Period: When introducing a new version, provide a deprecation period for the older version. During this time, inform users about the upcoming changes and encourage them to migrate.
- Clear Documentation: Maintain clear and up-to-date documentation that outlines version differences and migration steps.
- Automated Testing: Implement automated tests to ensure that changes made to one version do not inadvertently affect other versions.
Conclusion
Versioning is a fundamental aspect of API development that ensures a smooth evolution of your software while maintaining compatibility with existing clients. By adopting versioning strategies and following the step-by-step implementation guide outlined in this article, you can effectively manage API versions in your CakePHP project. Remember to prioritize clear communication, provide adequate documentation, and consider best practices to create a positive experience for both API developers and consumers.
Table of Contents
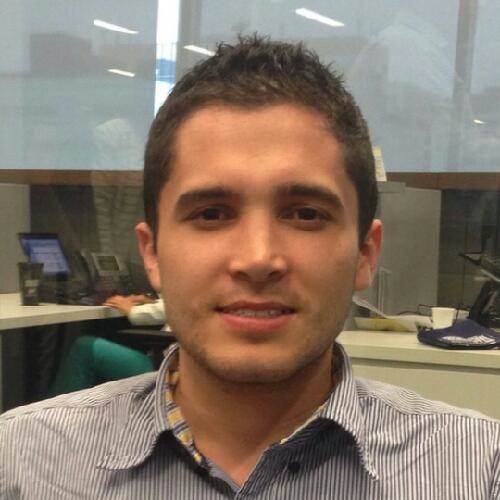
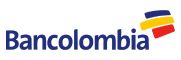