Implementing Ajax Functionality in CakePHP
As web development evolves, user expectations grow higher, demanding faster and more interactive experiences. Ajax (Asynchronous JavaScript and XML) is a powerful technology that allows you to achieve just that. In this tutorial, we will explore how to implement Ajax functionality in CakePHP, a popular PHP framework, to create dynamic and responsive web applications. We’ll cover the basics of Ajax, its benefits, and provide step-by-step instructions, along with code samples, to help you integrate Ajax seamlessly into your CakePHP projects.
Table of Contents
1. Understanding Ajax and Its Benefits:
Ajax is a client-side technology that enables data to be fetched from and sent to the server asynchronously without requiring a full page reload. This results in a smoother user experience and reduces the load on the server. Ajax empowers developers to create dynamic and interactive web applications, improving overall responsiveness and usability.
2. Setting Up the CakePHP Environment:
Before we dive into Ajax implementation, ensure you have a working CakePHP environment. If you haven’t installed CakePHP, visit the official website (https://cakephp.org/) and follow the installation instructions for your operating system.
3. Incorporating jQuery into CakePHP:
CakePHP makes it simple to include jQuery in your project. Download the latest jQuery library from the official website (https://jquery.com/) and place it in the webroot/js directory of your CakePHP application. Now, let’s modify the layout to include jQuery in all our pages.
php // File: src/Template/Layout/default.ctp <!DOCTYPE html> <html> <head> <!-- Add jQuery --> <?= $this->Html->script('jquery.min.js') ?> <!-- Your other header elements --> </head> <body> <!-- Your content here --> </body> </html>
4. Handling Ajax Requests:
CakePHP provides built-in support for Ajax requests, making it convenient to implement the necessary backend handling. To begin, let’s use CakePHP’s built-in Ajax Layout.
4.1. Using CakePHP’s Built-in Ajax Layout:
The Ajax layout in CakePHP is designed specifically for handling Ajax requests. It removes unnecessary elements, such as headers and footers, from the response, ensuring only the required data is sent back.
To utilize the Ajax layout, create a new file named ajax.ctp in your layout directory (src/Template/Layout/ajax.ctp). In this file, include only the content you want to return in the Ajax response.
php // File: src/Template/Layout/ajax.ctp <?= $this->fetch('content') ?>
Next, we need to configure the response to use the Ajax layout. In your controller action, use the RequestHandler component to set the layout to ‘ajax’.
php // File: src/Controller/YourController.php public function initialize(): void { parent::initialize(); $this->loadComponent('RequestHandler'); } public function ajaxAction() { $this->viewBuilder()->setLayout('ajax'); // Your logic to process the Ajax request and set necessary variables. }
4.2. Creating an Ajax Controller:
Using CakePHP’s built-in Ajax layout is helpful, but in more complex scenarios, you might need dedicated controllers to handle Ajax requests. Create a new controller, say AjaxController, and extend it from the main AppController.
php // File: src/Controller/AjaxController.php namespace App\Controller; use App\Controller\AppController; class AjaxController extends AppController { public function initialize(): void { parent::initialize(); $this->loadComponent('RequestHandler'); $this->viewBuilder()->setLayout('ajax'); } // Your action methods to handle Ajax requests. }
5. Making Ajax Requests from the Client-Side:
To send Ajax requests from the client-side, we’ll use jQuery’s $.ajax() function, which offers a straightforward way to interact with the server.
5.1. Using jQuery’s $.ajax() Function:
The $.ajax() function allows you to make HTTP requests with various settings, such as the URL, data to send, type of request, etc. Here’s a simple example of how to use $.ajax() to fetch data from the server.
javascript // File: webroot/js/custom.js $(document).ready(function() { $('#load-data-button').click(function() { $.ajax({ url: '/ajax_controller/ajax_action', type: 'GET', dataType: 'html', success: function(data) { $('#data-container').html(data); }, error: function(xhr, status, error) { console.error('Error:', error); } }); }); });
In this example, when the element with the ID load-data-button is clicked, an Ajax GET request is sent to the URL /ajax_controller/ajax_action, and the HTML response is appended to the element with the ID data-container.
5.2. Sending Data with Ajax Requests:
In many cases, you’ll need to send data to the server as part of your Ajax request. You can achieve this by setting the data option in the $.ajax() function.
javascript // File: webroot/js/custom.js $(document).ready(function() { $('#submit-form-button').click(function() { var formData = { username: $('#username-input').val(), email: $('#email-input').val(), // Other form fields }; $.ajax({ url: '/ajax_controller/save_data', type: 'POST', dataType: 'json', data: formData, success: function(response) { // Handle the server's JSON response. }, error: function(xhr, status, error) { console.error('Error:', error); } }); }); });
In this example, when the element with the ID submit-form-button is clicked, the form data is collected, serialized as JSON, and sent to the URL /ajax_controller/save_data as a POST request. The server should handle the data and return a JSON response.
6. Processing Ajax Responses:
Once the server processes an Ajax request, it needs to respond appropriately. We’ll cover two common scenarios: rendering views for Ajax requests and returning JSON responses.
6.1. Rendering Views for Ajax Requests:
When you want to return HTML content as an Ajax response, you can use CakePHP’s built-in render() method to render a specific view.
php // File: src/Controller/AjaxController.php public function ajaxAction() { // Your logic to process the Ajax request and set necessary variables. // Render the view without the layout $this->viewBuilder()->setLayout(false); $this->render('/ControllerName/ajax_action'); }
In this example, when the ajaxAction() method is called, it processes the request and renders the ajax_action.ctp view without the layout.
6.2. Returning JSON Responses:
Returning JSON responses is useful when you need to send structured data to the client for further processing. Use the response object to set the data and content type for the JSON response.
php // File: src/Controller/AjaxController.php public function saveData() { // Your logic to process the data and prepare the response. $responseData = [ 'success' => true, 'message' => 'Data saved successfully!', // Other response data ]; $this->response = $this->response->withType('application/json') ->withStringBody(json_encode($responseData)); return $this->response; }
In this example, when the saveData() method is called, it processes the data and prepares a JSON response with a success message.
7. Implementing a Live Search Feature:
A live search feature is a classic example of using Ajax to improve user experience. We’ll implement a simple live search using Ajax in CakePHP.
7.1. Creating the Search Form:
Start by creating a search form with an input field to capture the user’s query.
html <!-- File: src/Template/Ajax/live_search.ctp --> <form id="live-search-form"> <input type="text" id="search-query" placeholder="Enter your search query..."> <div id="search-results"></div> </form>
7.2. Handling Search Requests:
In the controller, process the search query and return relevant results as an HTML response.
php // File: src/Controller/AjaxController.php public function liveSearch() { $query = $this->request->getQuery('q'); // Your logic to perform the search and obtain search results. $results = $this->YourModel->find('all', [ 'conditions' => [ 'YourModel.field LIKE' => '%' . $query . '%' ], 'limit' => 10 ]); $this->set(compact('results')); $this->viewBuilder()->setLayout(false); $this->render('/Ajax/live_search_results'); }
In this example, the liveSearch() method processes the search query, retrieves relevant results from the model, and renders the live_search_results.ctp view without the layout.
7.3. Displaying Search Results with Ajax:
Now, let’s handle the client-side part to make the live search work.
javascript // File: webroot/js/custom.js $(document).ready(function() { $('#search-query').keyup(function() { var query = $(this).val(); $.ajax({ url: '/ajax_controller/live_search', type: 'GET', dataType: 'html', data: { q: query }, success: function(data) { $('#search-results').html(data); }, error: function(xhr, status, error) { console.error('Error:', error); } }); }); });
In this example, when the user types in the search input field, an Ajax request is sent to the URL /ajax_controller/live_search with the query parameter. The server processes the query, returns the HTML search results, and displays them in the search-results element.
8. Preventing CSRF Attacks with Ajax:
As with regular form submissions, Ajax requests are also susceptible to CSRF (Cross-Site Request Forgery) attacks. Protect your Ajax requests by including CSRF tokens.
javascript // File: webroot/js/custom.js // Include the CSRF token in all Ajax requests using jQuery $.ajaxSetup({ headers: { 'X-CSRF-Token': $('meta[name="csrf-token"]').attr('content') } });
In your layout file, include the CSRF token in the meta tags:
php // File: src/Template/Layout/default.ctp <!DOCTYPE html> <html> <head> <!-- Add jQuery --> <?= $this->Html->script('jquery.min.js') ?> <!-- CSRF token --> <?= $this->Html->meta('csrf-token', $this->request->getParam('_csrfToken')); ?> <!-- Your other header elements --> </head> <body> <!-- Your content here --> </body> </html>
Conclusion
Incorporating Ajax functionality into your CakePHP applications can significantly enhance user experience and interactivity. You’ve learned the basics of Ajax, setting up the environment, incorporating jQuery, handling Ajax requests, making requests from the client-side, processing responses, and implementing a live search feature. By following this step-by-step guide, you can create dynamic and responsive web applications that meet modern user expectations.
Remember that while Ajax offers many advantages, improper implementation can lead to potential security risks. Always sanitize and validate user inputs, and use CSRF protection to secure your Ajax requests.
With this newfound knowledge, you can elevate your CakePHP projects and create seamless user experiences that leave a lasting impression on your audience. Happy coding!
Table of Contents
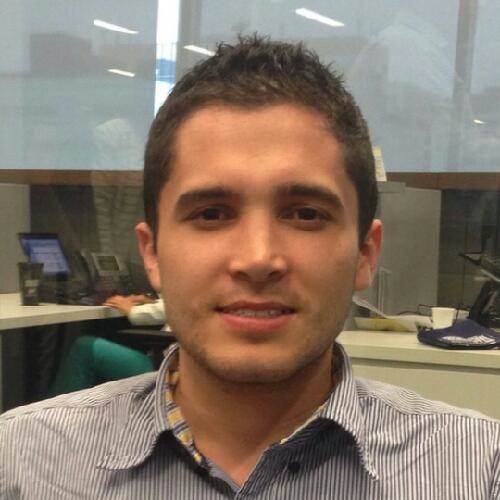
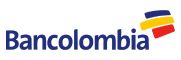