Implementing Custom Authentication in CakePHP
CakePHP is a powerful PHP framework that simplifies web application development. One of its crucial aspects is user authentication, which is fundamental for most web applications. While CakePHP provides built-in authentication mechanisms, there are situations where you might need a custom approach to meet specific requirements. In this tutorial, we’ll delve into the world of custom authentication in CakePHP, exploring how to enhance security and tailor authentication to your application’s needs.
Table of Contents
1. Why Custom Authentication in CakePHP?
CakePHP offers a robust built-in authentication system that supports various authentication methods like Form, Basic, Digest, and more. These built-in methods are excellent for many scenarios, providing a solid foundation for user authentication. However, there are cases where custom authentication becomes necessary:
1.1. Integration with External Systems
You might need to integrate CakePHP with an external authentication system such as LDAP, OAuth, or a third-party API. In such cases, custom authentication allows you to adapt CakePHP to work seamlessly with these systems.
1.2. Complex User Authentication Rules
Your application might require complex authentication rules beyond what the built-in methods offer. Custom authentication empowers you to implement intricate logic while keeping your codebase clean and maintainable.
1.3. Enhanced Security
Custom authentication lets you implement additional security measures, such as two-factor authentication (2FA) or custom password hashing algorithms, to protect user data effectively.
2. Getting Started with Custom Authentication
To implement custom authentication in CakePHP, follow these steps:
2.1. Create a Custom Authentication Adapter
Start by creating a custom authentication adapter that extends CakePHP’s BaseAuthenticate class. This adapter will contain the logic for authenticating users based on your custom rules.
Here’s a simplified example of a custom authentication adapter:
php // src/Auth/CustomAuthenticator.php namespace App\Auth; use Cake\Auth\BaseAuthenticate; use Cake\Http\Response; use Cake\Http\ServerRequest; class CustomAuthenticator extends BaseAuthenticate { public function authenticate(ServerRequest $request, Response $response) { // Your custom authentication logic goes here } }
2.2. Configure Authentication
Next, you need to configure your custom authentication adapter in CakePHP’s authentication component. Open your config/app.php file and locate the Authentication section. Add your custom adapter to the identifiers and authenticators arrays:
php // config/app.php 'Authentication' => [ 'identifiers' => [ 'Password' => [ 'className' => 'Authentication.Password', 'fields' => [ 'username' => 'email', 'password' => 'password', ], ], ], 'authenticators' => [ 'Custom' => [ 'className' => 'App\Auth\CustomAuthenticator', ], ], // ... ],
This configuration tells CakePHP to use your custom authentication adapter alongside other built-in methods like ‘Password’.
2.3. Implement the Authentication Logic
In your custom authentication adapter (CustomAuthenticator.php), implement the authenticate method. This is where you define your custom logic to authenticate users.
php // src/Auth/CustomAuthenticator.php namespace App\Auth; use Cake\Auth\BaseAuthenticate; use Cake\Http\Response; use Cake\Http\ServerRequest; class CustomAuthenticator extends BaseAuthenticate { public function authenticate(ServerRequest $request, Response $response) { // Your custom authentication logic goes here $user = $this->_findUser($request->getData('username')); if (!$user) { return null; } $password = $request->getData('password'); if (password_verify($password, $user['password_hash'])) { return $user; } return null; } }
In this example, we’re checking the submitted username and password against a user record in the database using the password_verify function. Modify this logic according to your application’s requirements.
2.4. Update Login Action
Now, update your login action in your controller to use the custom authentication adapter. Here’s a sample login action in a UsersController:
php // src/Controller/UsersController.php use Cake\Controller\Controller; use Cake\Event\EventInterface; class UsersController extends Controller { public function initialize(): void { parent::initialize(); $this->loadComponent('Authentication.Authentication'); } public function login() { if ($this->request->is('post')) { $result = $this->Authentication->getResult(); if ($result->isValid()) { // Redirect authenticated users to the desired location return $this->redirect(['controller' => 'Dashboard', 'action' => 'index']); } $this->Flash->error('Invalid username or password'); } } }
In the initialize method, we load the Authentication component, and in the login action, we use $this->Authentication->getResult() to check the authentication result.
2.5. Create a Custom User Provider (Optional)
If you need to customize user data retrieval further, you can create a custom user provider. A user provider retrieves user data for authentication and authorization purposes. Here’s an example:
php // src/Auth/CustomUserProvider.php namespace App\Auth; use Cake\Auth\BaseAuthenticate; use Cake\Http\ServerRequest; use Cake\Utility\Security; class CustomUserProvider extends BaseAuthenticate { public function findUser($username, $password) { // Custom logic to retrieve user data } }
Configure your custom user provider in config/app.php:
php // config/app.php 'Authentication' => [ 'identifiers' => [ 'Password' => [ 'className' => 'Authentication.Password', 'fields' => [ 'username' => 'email', 'password' => 'password', ], ], ], 'authenticators' => [ 'Custom' => [ 'className' => 'App\Auth\CustomAuthenticator', ], ], 'providers' => [ 'Custom' => [ 'className' => 'App\Auth\CustomUserProvider', ], ], // ... ],
Now, the custom user provider (CustomUserProvider.php) is responsible for retrieving user data during authentication.
3. Enhancing Security with Custom Authentication
Custom authentication in CakePHP allows you to implement additional security measures beyond the built-in options. Here are some security enhancements you can implement:
3.1. Two-Factor Authentication (2FA)
To add an extra layer of security, implement two-factor authentication (2FA) in your custom authentication logic. This can be achieved by sending a one-time code to the user’s registered email or mobile device and validating it during login.
3.2. Custom Password Hashing
You can use custom password hashing algorithms, such as bcrypt or Argon2, to securely store and verify user passwords. CakePHP’s custom authentication allows you to use these algorithms easily.
3.3. Rate Limiting
Implement rate limiting to protect against brute force attacks. Limit the number of login attempts from a single IP address within a specified timeframe.
3.4. Session Management
Enhance session management by implementing features like session expiration, automatic logout after a period of inactivity, and session regeneration to prevent session fixation attacks.
Conclusion
Custom authentication in CakePHP provides the flexibility and control needed to implement advanced authentication mechanisms tailored to your application’s requirements. Whether you need to integrate with external systems, implement complex authentication rules, or enhance security, CakePHP’s custom authentication allows you to achieve your goals effectively.
In this tutorial, we’ve covered the essential steps to get started with custom authentication in CakePHP, from creating a custom authentication adapter to configuring the authentication component. Additionally, we explored ways to enhance security through two-factor authentication, custom password hashing, rate limiting, and session management.
By mastering custom authentication in CakePHP, you can ensure that your web applications are not only secure but also capable of meeting the most demanding authentication requirements.
So, go ahead and start implementing custom authentication in your CakePHP applications, and take control of your authentication process today. Your users and your application’s security will thank you for it!
Table of Contents
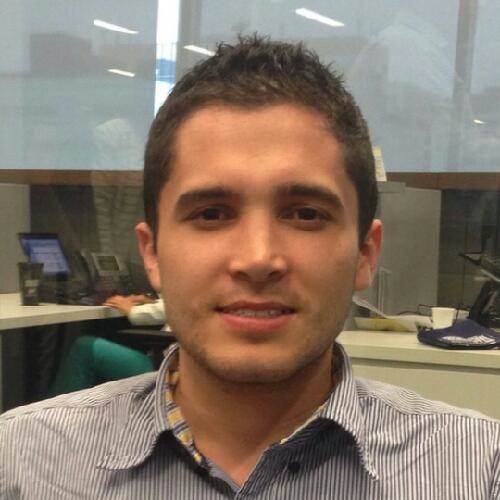
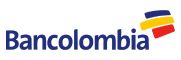