Implementing File Uploads in CakePHP: Handling User Files
File uploads are a common requirement in web applications, allowing users to share images, documents, and other types of files. When working with the CakePHP framework, efficiently handling user files becomes a crucial aspect of creating a seamless user experience. In this tutorial, we will walk you through the process of implementing file uploads in CakePHP. We will cover the necessary steps, best practices, and code samples to help you integrate file upload functionality into your CakePHP application.
Table of Contents
1. Introduction to File Uploads in CakePHP
File uploads involve allowing users to send files from their local systems to a web server. This feature is commonly used in content management systems, social media platforms, and various other web applications. In CakePHP, implementing file uploads involves a series of steps, including creating a form for users to upload files, validating the uploaded files, managing the files on the server, and displaying them to users as needed.
2. Setting Up Your CakePHP Environment
Before we dive into implementing file uploads, ensure you have a functional CakePHP environment set up. This includes having the CakePHP framework installed, a database configured, and a basic understanding of CakePHP’s MVC architecture.
3. Creating the File Upload Form
To start, you’ll need a form that allows users to select and upload files. In your CakePHP application’s view, create a form that includes an input field of type “file”:
php <?= $this->Form->create(null, ['type' => 'file']) ?> <?= $this->Form->file('file') ?> <?= $this->Form->button(__('Upload File')) ?> <?= $this->Form->end() ?>
This code creates a form with a file input field and a submit button. The ‘type’ => ‘file’ option ensures that the form supports file uploads.
4. Handling File Uploads in the Controller
Next, let’s move on to the controller to handle the uploaded files. In your controller action, you can access the uploaded file using the $this->request->getData(‘file’) method. Let’s assume the controller action is named upload:
php public function upload() { if ($this->request->is('post')) { $uploadedFile = $this->request->getData('file'); // Process the uploaded file } }
4.1. Validating Uploaded Files
Before processing the uploaded file, it’s essential to validate it to ensure it meets your application’s requirements. CakePHP provides validation rules for files, such as checking file type, size, and other properties. You can create a validation rule in your controller action like this:
php $validator = new \Cake\Validation\Validator(); $validator ->add('file', 'fileType', [ 'rule' => ['mimeType', ['image/jpeg', 'image/png']], 'message' => 'Only JPEG and PNG files are allowed.' ]) ->add('file', 'fileSize', [ 'rule' => ['fileSize', '<=', '2MB'], 'message' => 'File size must be less than 2MB.' ]); $errors = $validator->validate($this->request->getData()); if (empty($errors)) { // File is valid, proceed with processing } else { // Invalid file, display error messages $this->Flash->error(__('File upload validation failed.')); }
4.2. Moving Uploaded Files to the Destination
Once you’ve validated the uploaded file, you need to move it to a designated location on your server. CakePHP makes this process straightforward. Choose a suitable directory for your uploads (e.g., webroot/uploads) and use the move_uploaded_file function:
php $uploadDir = WWW_ROOT . 'uploads'; $filename = time() . '_' . $uploadedFile['name']; $filePath = $uploadDir . DS . $filename; if (move_uploaded_file($uploadedFile['tmp_name'], $filePath)) { // File uploaded successfully } else { // Error in uploading }
5. Displaying Uploaded Files and File Management
Once files are uploaded and saved on your server, you might want to display them to users. You can create a view that lists the uploaded files and provides links for users to download or view them. Fetch the list of uploaded files and pass it to the view:
php public function viewFiles() { $files = scandir(WWW_ROOT . 'uploads'); $this->set(compact('files')); } In the corresponding view, you can loop through the list of files and generate links for each: php Copy code <ul> <?php foreach ($files as $file): ?> <li> <?= h($file) ?> - <?= $this->Html->link('Download', ['action' => 'download', $file]) ?> </li> <?php endforeach; ?> </ul>
6. Security Considerations
When implementing file uploads, security is paramount. Apply measures to prevent unauthorized access to uploaded files. It’s recommended to place an .htaccess file in your upload directory to restrict direct access:
apache # Deny access to all files in this directory Deny from all
Additionally, sanitize the file names to prevent malicious attempts, and consider using a secure file storage solution if your application handles sensitive data.
Conclusion
In this tutorial, we’ve explored the process of implementing file uploads in CakePHP. From creating the upload form to handling and validating uploaded files, as well as displaying them to users, you’ve gained a solid understanding of the essential steps involved. Remember to prioritize security when implementing file uploads and consider the specific requirements of your application. By following these guidelines and best practices, you can seamlessly integrate file upload functionality into your CakePHP application, enhancing the user experience and expanding your application’s capabilities.
Table of Contents
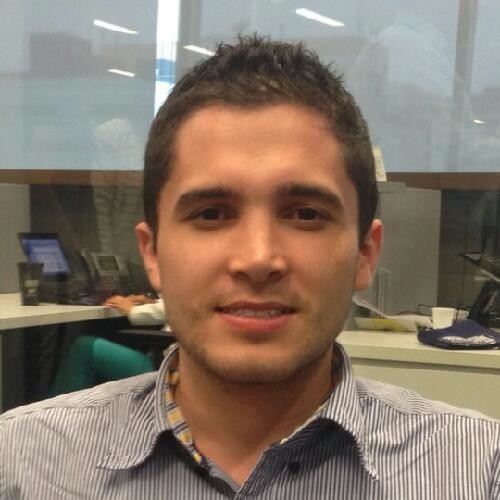
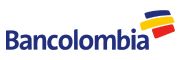