CakePHP and AI: Integrating Artificial Intelligence
In today’s fast-paced digital landscape, web applications are becoming increasingly sophisticated, offering users personalized and intelligent experiences. One of the key technologies driving this transformation is Artificial Intelligence (AI). Integrating AI into web applications can unlock a world of possibilities, from predictive analytics to natural language processing. In this comprehensive guide, we will delve into the exciting realm of integrating AI with CakePHP, a popular PHP framework. We will explore the benefits, techniques, and provide code samples to help you get started on your journey to creating AI-powered web applications.
Table of Contents
1. Understanding the Power of AI in Web Applications
1.1. Why Integrate AI with CakePHP?
Artificial Intelligence has evolved from being a buzzword to becoming an indispensable part of modern web applications. Here are some compelling reasons to integrate AI with CakePHP:
1.1.1. Enhanced User Experience
AI can analyze user behavior, preferences, and history to provide personalized content and recommendations. This leads to higher user engagement and satisfaction.
1.1.2. Predictive Analytics
With AI, web applications can predict user actions, helping businesses make data-driven decisions. For example, an e-commerce site can predict which products a user is likely to purchase based on their browsing history.
1.1.3. Automation
AI can automate repetitive tasks, such as data entry, customer support, and content moderation, freeing up human resources for more strategic activities.
1.1.4. Improved Security
AI can identify and respond to security threats in real-time. This is crucial for protecting sensitive user data and maintaining the integrity of your web application.
1.2 The Versatility of CakePHP
CakePHP is a robust PHP framework known for its simplicity and ease of use. It provides a strong foundation for building web applications quickly and efficiently. Some of the key features of CakePHP include:
- MVC Architecture: CakePHP follows the Model-View-Controller (MVC) architectural pattern, making it easy to separate application logic from presentation.
- Database Integration: CakePHP offers built-in tools for database interaction, simplifying data retrieval and manipulation.
- Scaffolding: With CakePHP’s scaffolding feature, you can generate basic CRUD (Create, Read, Update, Delete) functionality with minimal code.
- Security: CakePHP comes with security features like data validation, SQL injection prevention, and CSRF protection, crucial for secure web applications.
Now that we understand the benefits of integrating AI with CakePHP and the strengths of the framework itself, let’s move on to setting up our environment.
2. Preparing Your Environment
Before we dive into integrating AI with CakePHP, it’s essential to have the necessary tools and libraries in place. Here are the key steps to prepare your environment:
2.1 Setting Up CakePHP
To get started, you’ll need to install CakePHP. You can follow the official CakePHP installation guide, which provides detailed instructions for different setups. Here’s a summary of the basic steps:
bash # Use Composer to create a new CakePHP project composer create-project --prefer-dist cakephp/app myproject # Navigate to your project directory cd myproject # Start the built-in development server bin/cake server
This will set up a new CakePHP project and start a development server. You can access your CakePHP application at http://localhost:8765.
2.2 AI Tools and Libraries
To integrate AI into your CakePHP application, you’ll need access to AI tools and libraries. Some popular AI libraries for PHP include:
- PHP-ML: PHP-ML is a machine learning library for PHP that provides various algorithms for classification, regression, and clustering.
- TensorFlow-PHP: If you’re working with deep learning and TensorFlow models, TensorFlow-PHP provides bindings for using TensorFlow in PHP applications.
- Natural Language Toolkit (NLTK): NLTK is a Python library for natural language processing. You can use it in combination with PHP for tasks like sentiment analysis.
Make sure to install and configure these libraries based on your specific AI requirements.
Now that your environment is set up, let’s explore how AI can enhance your CakePHP application.
3. AI-Powered User Personalization
User personalization is a crucial aspect of modern web applications. AI can play a significant role in providing personalized experiences to your users.
3.1 Recommender Systems
Recommender systems, also known as recommendation engines, are a classic example of AI-powered user personalization. These systems analyze user behavior and preferences to suggest content or products that users are likely to be interested in.
3.1.1. How Recommender Systems Work
Recommender systems work by leveraging user data, such as browsing history, purchase history, and user demographics, to make personalized recommendations. There are two main types of recommender systems:
- Collaborative Filtering: This approach identifies users who have similar preferences and recommends items that those similar users have liked. Collaborative filtering can be user-based or item-based.
- Content-Based Filtering: Content-based filtering recommends items based on their attributes and descriptions. It focuses on the features of the items and matches them with the user’s preferences.
3.2 Implementing a Recommender System with CakePHP and AI
Let’s see how you can implement a basic content-based recommender system in a CakePHP application using the PHP-ML library. This example assumes you have a database table called products with attributes like product_id, name, and description.
php // Load the PHP-ML library require 'vendor/autoload.php'; use Phpml\FeatureExtraction\TfIdfTransformer; use Phpml\FeatureExtraction\TokenCountVectorizer; use Phpml\Math\Distance\Cosine; // Sample product data (you would fetch this from your database) $products = [ ['product_id' => 1, 'name' => 'Product A', 'description' => 'Description of Product A'], ['product_id' => 2, 'name' => 'Product B', 'description' => 'Description of Product B'], // Add more products here ]; // User's preferences (for demonstration purposes) $userPreferences = [ 'Product A' => 5, // Liked Product A ]; // Extract product descriptions $descriptions = array_column($products, 'description'); // Initialize TF-IDF transformer $vectorizer = new TokenCountVectorizer(new TfIdfTransformer()); $vectorizer->fit($descriptions); $vectorizer->transform($descriptions); // Calculate cosine similarity between user preferences and product descriptions $cosine = new Cosine(); $similarities = []; foreach ($descriptions as $key => $description) { $similarity = $cosine->similarity($userPreferences, $description); $similarities[$key] = $similarity; } // Sort products by similarity (higher similarity means a better recommendation) arsort($similarities); // Get recommended products $recommendedProducts = []; foreach ($similarities as $key => $similarity) { $recommendedProducts[] = $products[$key]; } // Display recommended products foreach ($recommendedProducts as $product) { echo "Product ID: {$product['product_id']}, Name: {$product['name']}\n"; }
In this code sample, we first load the PHP-ML library and define sample product data and user preferences. We then extract product descriptions and calculate the cosine similarity between the user preferences and product descriptions. Finally, we sort the products by similarity and display the recommended products.
This is a simplified example, and in a real-world application, you would use a more extensive dataset and refine your recommendation algorithm for better results. Nonetheless, it demonstrates how AI can enhance user personalization in your CakePHP application.
4. Natural Language Processing (NLP) in CakePHP
Natural Language Processing (NLP) is another domain of AI that can add significant value to your CakePHP application. NLP techniques enable your application to understand and interpret human language.
4.1 Sentiment Analysis
Sentiment analysis, also known as opinion mining, is a common NLP task that involves determining the sentiment or emotion expressed in a piece of text. For example, you can use sentiment analysis to gauge whether user reviews are positive, negative, or neutral.
4.1.1. Code Sample: Sentiment Analysis in CakePHP
Let’s explore how to perform sentiment analysis in a CakePHP application using the NLTK library for Python. While NLTK is a Python library, you can use it in combination with CakePHP by setting up an API endpoint for sentiment analysis.
First, you’ll need to install NLTK in your Python environment:
bash pip install nltk
Next, create a Python script for sentiment analysis, let’s call it sentiment_analysis.py:
python import nltk from nltk.sentiment.vader import SentimentIntensityAnalyzer # Download NLTK data nltk.download('vader_lexicon') # Initialize the sentiment analyzer analyzer = SentimentIntensityAnalyzer() def analyze_sentiment(text): # Get sentiment scores sentiment_scores = analyzer.polarity_scores(text) # Determine sentiment based on the compound score sentiment = "positive" if sentiment_scores['compound'] > 0 else "negative" if sentiment_scores['compound'] < 0 else "neutral" return sentiment
Now, let’s create a CakePHP controller that communicates with the Python script via an API endpoint. Create a file named SentimentController.php:
php // app/Controller/SentimentController.php class SentimentController extends AppController { public function analyze() { // Get the text to analyze from the request $text = $this->request->getData('text'); // Call the Python script for sentiment analysis $sentiment = exec("python path/to/sentiment_analysis.py '$text'"); // Return the sentiment result as JSON $this->set(compact('sentiment')); $this->viewBuilder()->setOption('serialize', ['sentiment']); } }
In this code, we create a SentimentController with an analyze action. This action receives text data from the client, calls the Python script for sentiment analysis, and returns the sentiment result as JSON.
You can now create a route in your config/routes.php file to map to this controller action.
This example demonstrates how you can leverage NLP and external libraries like NLTK to perform sentiment analysis in a CakePHP application, enhancing your ability to understand and respond to user-generated content.
5. AI-Enhanced Security
Security is a paramount concern for web applications, and AI can be a valuable ally in identifying and mitigating security threats.
5.1 Intrusion Detection
Intrusion detection systems (IDS) are critical for identifying and responding to security breaches and malicious activities. AI-powered IDS can analyze patterns of activity and detect anomalies in real-time.
5.1.1. Integrating Intrusion Detection with CakePHP
To integrate an AI-powered intrusion detection system with CakePHP, you can follow these steps:
- Collect and centralize log data from your CakePHP application, including server logs, access logs, and application-specific logs.
- Implement an AI model that can analyze log data and detect anomalies. You can use machine learning algorithms for this purpose, such as clustering or anomaly detection.
- Set up real-time monitoring of log data and trigger alerts when anomalies are detected. You can use tools like Elasticsearch, Logstash, and Kibana (ELK Stack) to accomplish this.
- Implement response mechanisms to mitigate threats when anomalies are detected, such as blocking suspicious IP addresses or alerting administrators.
By integrating AI-enhanced security measures, you can proactively protect your CakePHP application and user data from potential threats.
Conclusion
Integrating Artificial Intelligence with CakePHP opens up a world of possibilities for enhancing user experiences, making data-driven decisions, and improving security. Whether you’re implementing recommender systems for personalized content, performing sentiment analysis for user-generated content, or bolstering security with AI-powered intrusion detection, the combination of AI and CakePHP can take your web application to new heights.
As you embark on your journey to integrate AI with CakePHP, remember that the field of AI is constantly evolving. Stay updated with the latest advancements, experiment with different AI techniques, and continuously refine your application to deliver the best possible experience to your users.
With the power of CakePHP and the intelligence of AI, you have the tools to create web applications that are not just functional but also intuitive and adaptive to the needs of your users. Embrace the future of web development by harnessing the capabilities of AI in your CakePHP projects.
Table of Contents
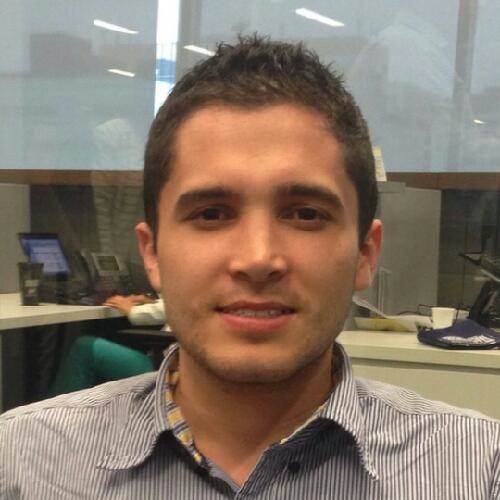
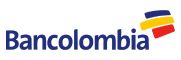