Implementing Internationalization and Localization in CakePHP
In today’s interconnected world, building applications that cater to users from diverse linguistic backgrounds is essential. To achieve this, developers need to implement Internationalization (i18n) and Localization (l10n) in their web applications. CakePHP, a popular PHP framework, provides excellent support for making your application multilingual. In this blog, we will explore how to implement Internationalization and Localization in CakePHP to create versatile, user-friendly, and truly global web applications. We will cover everything from configuring the framework to translating content and handling pluralization.
1. What is Internationalization and Localization?
Before diving into the technical aspects, let’s understand the concepts of Internationalization and Localization. Internationalization (i18n) is the process of designing and developing an application in a way that makes it easy to adapt to various languages and cultural conventions. Localization (l10n), on the other hand, is the process of adapting the application for a specific locale or language. By combining these two, you can create a multilingual application that can serve users from different regions seamlessly.
2. Setting Up CakePHP for Multilingual Support
CakePHP comes with built-in support for i18n and l10n. To enable multilingual support in your CakePHP application, you need to make a few configurations.
Step 1: Configure App.php
In your config/app.php file, set the default language and any additional languages you want to support. Make sure you have the corresponding language folders under src/Locale.
php // config/app.php return [ 'App' => [ 'defaultLocale' => 'en_US', 'defaultTimezone' => 'UTC', 'supportedLocales' => [ 'en_US', 'es_ES', // Add more languages as needed ], ], ];
3. Preparing Language Files
CakePHP uses .po (Portable Object) files to store translations. These files contain key-value pairs, where the key represents the original text in the source language (usually English), and the value represents the translation in the target language.
Step 1: Create Language Files
Create a folder for each supported language under src/Locale. Inside each folder, create a subfolder named LC_MESSAGES. This is where you will store the .po files.
Example folder structure:
css src/ Locale/ en_US/ LC_MESSAGES/ es_ES/ LC_MESSAGES/ …
Step 2: Generate POT File
A POT (Portable Object Template) file contains all the translatable strings in your application. You can generate this file using the CakePHP shell:
bash bin/cake i18n extract
This command will create a default.pot file in the src/Locale directory. You can now use this file to generate .po files for each language.
Step 3: Generate PO Files
Use a translation tool like POEdit to create .po files based on the default.pot file. Save the .po files inside the respective language folders.
4. Translating Static Text
Once you have prepared the .po files, you can start translating static text in your CakePHP application.
Step 1: Load the Localization Component
In your AppController.php, load the Localization component.
php // src/Controller/AppController.php public function initialize() { parent::initialize(); $this->loadComponent('Localization'); }
Step 2: Translate Static Text
In your views and templates, use the __() function to translate static text. The __() function takes the original text as the first parameter and returns the translated text based on the user’s selected language.
php // src/Template/Posts/index.ctp <h1><?= __('Welcome to our Blog') ?></h1> <p><?= __('Read our latest posts and stay updated.') ?></p>
5. Translating Database Content
Translating static text is only part of the process. Often, you’ll need to translate content stored in the database, such as user-generated content or product descriptions.
Step 1: Prepare the Database
Assuming you have a posts table with a title and body column that need to be translated, add two additional columns for each translatable field. For example, if you have English as the default language, add title_es and body_es for Spanish translations.
Step 2: Translate Database Content
In your controller or model, retrieve the appropriate translated content based on the user’s selected language.
php // src/Controller/PostsController.php public function view($id) { $post = $this->Posts->get($id); // Load the Localization component to get the current language $language = $this->Localization->getCurrentLanguage(); // Choose the appropriate translated field based on the current language $translatedTitle = $post->{"title_$language"}; $translatedBody = $post->{"body_$language"}; // Pass the translated content to the view $this->set(compact('post', 'translatedTitle', 'translatedBody')); }
6. Handling Pluralization
In some languages, words change depending on the quantity. CakePHP provides support for pluralization in the translations.
Step 1: Define Plural Forms in PO Files
Edit your .po files to include plural forms for translatable strings that require it.
po msgid "apple" msgid_plural "apples" msgstr[0] "manzana" msgstr[1] "manzanas"
Step 2: Use n__() for Plural Translations
In your views or templates, use the n__() function to handle plural translations.
php // src/Template/Posts/index.ctp <?php $appleCount = 3; // The number of apples to display echo n__('One apple', '%d apples', $appleCount, $appleCount); ?>
7. Language Switching
Allowing users to switch between languages is crucial for a multilingual application. We can achieve this by using a language switcher.
Step 1: Create the Language Switcher
In your layout or view, create a language switcher with links to switch the language.
php // src/Template/Layout/default.ctp <ul> <li><?= $this->Html->link(__('English'), ['controller' => 'Pages', 'action' => 'changeLanguage', 'en_US']) ?></li> <li><?= $this->Html->link(__('Español'), ['controller' => 'Pages', 'action' => 'changeLanguage', 'es_ES']) ?></li> <!-- Add more languages as needed --> </ul>
Step 2: Implement Language Change Action
In your controller, create an action to change the language and store it in a session variable.
php // src/Controller/PagesController.php public function changeLanguage($locale) { $this->request->getSession()->write('Config.locale', $locale); return $this->redirect($this->request->referer()); }
Conclusion
Congratulations! You have successfully implemented Internationalization and Localization in your CakePHP application. You’ve learned how to configure the framework, prepare language files, translate static text and database content, handle pluralization, and implement language switching. Your web application is now ready to serve users from various regions, making it more inclusive and accessible to a global audience.
Building multilingual applications is essential for reaching a broader user base, and CakePHP’s robust i18n and l10n support makes the process smooth and efficient. Keep exploring and enhancing your application to provide an outstanding user experience for all your users, regardless of their language or location. Happy coding!
Table of Contents
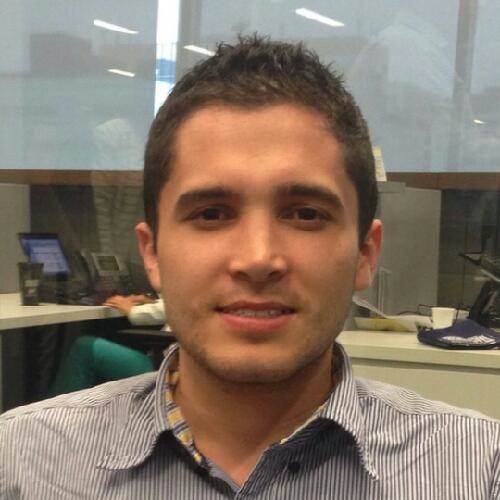
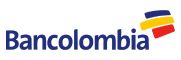