CakePHP and JavaScript: Leveraging the Power of JS Frameworks
In the world of web development, the marriage of server-side frameworks with client-side JavaScript has become increasingly crucial to deliver dynamic and interactive web applications. CakePHP, a popular PHP framework, and JavaScript, a versatile and widely-used programming language, can form a powerful duo when integrated seamlessly. In this blog, we will explore how you can leverage the power of JavaScript frameworks within CakePHP to create feature-rich, efficient, and modern web applications.
Table of Contents
1. Understanding the CakePHP Framework:
Before diving into the world of JavaScript integration, let’s briefly introduce CakePHP for those new to the framework.
1.1. What is CakePHP?
CakePHP is an open-source PHP web framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a robust set of libraries and features, making web application development faster and easier.
1.2. Benefits of CakePHP:
- Rapid development: CakePHP’s code generation features allow developers to create prototypes and fully-functional applications quickly.
- MVC architecture: The separation of concerns in MVC promotes maintainability and scalability.
- Built-in ORM: Object-Relational Mapping simplifies database interactions, enhancing data handling.
- Security features: CakePHP offers built-in security mechanisms to prevent common web application vulnerabilities.
- Extensive community: An active community provides support, plugins, and extensions.
2. Integrating JavaScript with CakePHP:
JavaScript plays a significant role in modern web development by adding interactivity and responsiveness to user interfaces. Integrating JavaScript libraries or frameworks with CakePHP can take your application to the next level.
2.1. Including JavaScript in CakePHP:
To start using JavaScript in CakePHP, you can include it in your layout or view files. The most common way is by linking to external JavaScript files or using inline scripts.
Example:
In the default.ctp layout file:
html <!DOCTYPE html> <html> <head> <!-- Other meta tags and CSS links --> <?php echo $this->Html->script('jquery.min'); ?> <?php echo $this->fetch('script'); ?> </head> <body> <!-- Content goes here --> </body> </html>
In a specific view file, let’s say index.ctp:
php <?php $this->start('script'); ?> <script> // Your custom JavaScript code here $(document).ready(function() { // Code to execute after the DOM is ready }); </script> <?php $this->end(); ?>
2.2. Utilizing JavaScript Frameworks:
JavaScript frameworks provide a structured way to build complex frontend applications. Let’s explore two popular JavaScript frameworks, React and Vue.js, and see how they can be integrated into CakePHP.
2.2.1. Using React with CakePHP:
React is a powerful JavaScript library for building user interfaces. It excels in creating dynamic, reusable components, making it a great choice for large-scale applications.
Integration Steps:
Step 1: Install React dependencies using npm:
bash npm install react react-dom @babel/preset-react
Step 2: Create a new React component, for example, MyComponent.jsx:
jsx import React from 'react'; const MyComponent = () => { return ( <div> <h1>Hello, I'm a React component!</h1> </div> ); }; export default MyComponent;
Step 3: Compile the JSX code using Babel. You can use Webpack or any other build tool to bundle your React components into a single file.
Step 4: Include the compiled JavaScript file in your CakePHP view:
php // In your view file, e.g., index.ctp $this->Html->script('path_to_compiled_js_file');
2.2.2. Integrating Vue.js with CakePHP:
Vue.js is another popular JavaScript framework that is known for its simplicity and ease of integration.
Integration Steps:
Step 1: Include Vue.js in your CakePHP application using a CDN or by installing it via npm.
html <!-- Using CDN --> <script src="https://unpkg.com/vue@latest"></script> bash Copy code # Using npm npm install vue
Step 2: Create a new Vue component, e.g., MyComponent.vue:
vue <template> <div> <h1>Hello, I'm a Vue component!</h1> </div> </template> <script> export default { // Vue component logic here }; </script>
Step 3: Compile the Vue component using a bundler like Webpack.
Step 4: Include the compiled JavaScript file in your CakePHP view:
php // In your view file, e.g., index.ctp $this->Html->script('path_to_compiled_js_file');
3. Handling AJAX Requests in CakePHP:
Asynchronous JavaScript and XML (AJAX) allows for dynamic content loading without refreshing the entire page. CakePHP provides built-in support for handling AJAX requests, making it easy to integrate JavaScript-powered functionalities into your application.
3.1. AJAX with jQuery:
jQuery simplifies AJAX requests, and since CakePHP includes jQuery by default, handling AJAX becomes straightforward.
Example:
In your view file, let’s say ajax_example.ctp:
html <!-- HTML content --> <div id="result"></div> <script> $(document).ready(function() { $.ajax({ url: '<?php echo $this->Url->build(["controller" => "MyController", "action" => "ajaxFunction"]); ?>', type: 'POST', dataType: 'json', data: { param1: 'value1', param2: 'value2' }, success: function(data) { $('#result').html(data.message); }, error: function() { $('#result').html('An error occurred.'); } }); }); </script>
In your MyController.php:
php public function ajaxFunction() { $this->autoRender = false; // Disable rendering of the layout and view $data = [ 'message' => 'This message is loaded asynchronously with AJAX!' ]; $this->response->withType('json')->getBody()->write(json_encode($data)); return $this->response; }
4. Enhancing Form Validation with JavaScript:
Client-side form validation provides instant feedback to users, reducing server load and improving user experience. JavaScript can be used to enhance CakePHP’s server-side form validation.
Example:
In your view file, e.g., add.ctp:
php <?php // Helper for generating form elements echo $this->Form->create('User'); // Your form fields here... // Use JavaScript to handle form submission echo $this->Form->button('Submit', [ 'id' => 'submit-button', 'type' => 'button', 'onclick' => 'validateFormAndSubmit()', ]); echo $this->Form->end(); ?> <script> function validateFormAndSubmit() { // Perform client-side form validation if (/* Validation passes */) { document.getElementById('submit-button').setAttribute('disabled', 'disabled'); document.forms[0].submit(); // Submit the form if validation passes } else { // Display error messages to the user // ... } } </script>
5. Implementing Real-time Communication with CakePHP and WebSocket:
WebSocket enables real-time communication between clients and servers, allowing data to be pushed from the server to the client without the need for continuous polling. By integrating WebSocket with CakePHP, you can build real-time features like chat applications or notifications.
Example:
Step 1: Set up a WebSocket server. You can use packages like Ratchet (https://github.com/ratchetphp/Ratchet) to implement WebSocket servers in PHP.
Step 2: Create a WebSocket component in your CakePHP application to handle real-time communication between the WebSocket server and the CakePHP application.
Conclusion
Combining CakePHP with JavaScript opens up a world of possibilities in web development. By leveraging the power of JavaScript frameworks like React and Vue.js, handling AJAX requests, enhancing form validation, and implementing real-time communication, you can create sophisticated, interactive, and efficient web applications. This synergy between CakePHP and JavaScript empowers developers to deliver outstanding user experiences and stay ahead in the ever-evolving world of web development.
Table of Contents
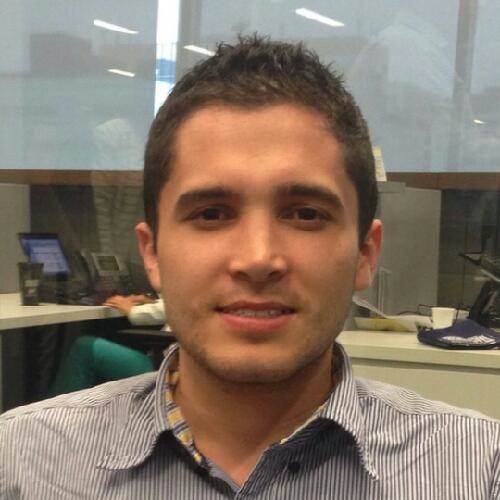
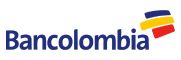