Working with CakePHP’s File Storage System: Local and Cloud
Managing files is an integral part of modern web applications, whether it’s handling user uploads, storing assets, or managing backups. CakePHP, a popular PHP framework, offers an efficient and versatile File Storage System that simplifies working with files. In this article, we’ll dive into CakePHP’s File Storage System, exploring its capabilities for both local and cloud-based storage solutions. Along the way, we’ll provide code samples and real-world examples to demonstrate how to leverage this system effectively.
Table of Contents
1. Setting Up CakePHP
Before we delve into the File Storage System, ensure you have a working CakePHP project. If not, follow these steps:
- Install Composer: If you don’t have Composer installed, download it from getcomposer.org.
- Create a New CakePHP Project: Open your terminal and run the following command:
bash composer create-project --prefer-dist cakephp/app myproject
- Navigate to the Project: Move to the project directory using:
bash cd myproject
2. Understanding CakePHP’s File Storage System
CakePHP’s File Storage System provides an abstraction layer for working with files, allowing seamless interaction with various storage backends. Two common storage solutions are local file storage and cloud-based storage, each offering its own advantages.
2.1. Local File Storage
Local file storage involves storing files on the server where your application is hosted. It’s suitable for managing user uploads, temporary files, and other assets that don’t require external access.
2.1.1. Setting Up Local Storage
CakePHP’s configuration file, config/app.php, contains settings for local storage. Open the file and find the ‘Storage’ configuration section. You can specify the ‘local’ adapter as follows:
php 'Storage' => [ 'local' => [ 'className' => 'Local', 'path' => WWW_ROOT . 'uploads/', // Path to the storage directory ], ],
In this example, files will be stored in the webroot/uploads/ directory of your CakePHP project.
2.1.2. Uploading Files Locally
Let’s create a simple example where users can upload profile pictures. First, generate a controller and views using CakePHP’s bake command:
bash bin/cake bake controller Profiles
Next, create a form in templates/Profiles/add.ctp for uploading a profile picture:
php <?= $this->Form->create(null, ['enctype' => 'multipart/form-data']) ?> <?= $this->Form->file('picture') ?> <?= $this->Form->button(__('Upload')) ?> <?= $this->Form->end() ?>
In your ProfilesController.php, handle the uploaded file:
php use Cake\Utility\Text; public function add() { if ($this->request->is('post')) { $picture = $this->request->getData('picture'); $filename = Text::slug($picture->getClientFilename()); $storage = StorageManager::get('local'); // Get the local storage adapter $path = 'profiles/' . $filename; if ($storage->store($path, $picture->getStream())) { $this->Flash->success(__('Picture uploaded successfully.')); } else { $this->Flash->error(__('Unable to upload picture.')); } } }
This code retrieves the uploaded file, generates a unique filename, and stores it using the local storage adapter.
2.2. Cloud-Based Storage
Cloud storage solutions like Amazon S3 and Google Cloud Storage provide scalable, secure, and reliable file storage. CakePHP’s File Storage System supports various cloud storage adapters.
2.2.1. Setting Up Cloud Storage
To work with cloud storage, you’ll need to install the corresponding CakePHP plugin. For example, to use Amazon S3, install the AWS SDK plugin:
bash composer require league/flysystem-aws-s3-v3
Next, configure the cloud storage settings in config/app.php:
php 'Storage' => [ 's3' => [ 'className' => 'League\Flysystem\AwsS3V3\AwsS3V3Adapter', 'client' => Aws\S3\S3Client::factory([ 'credentials' => [ 'key' => 'your-access-key', 'secret' => 'your-secret-key', ], 'region' => 'your-region', 'version' => 'latest', ]), 'bucket' => 'your-bucket-name', 'prefix' => 'optional-prefix/', // Optional ], ],
Replace ‘your-access-key’, ‘your-secret-key’, ‘your-region’, and ‘your-bucket-name’ with your actual AWS credentials and bucket information.
2.2.2. Uploading Files to Cloud Storage
Let’s modify the profile picture example to upload files to Amazon S3. You can reuse the same form and controller code, with a few adjustments:
php use Cake\Utility\Text; use League\Flysystem\AwsS3V3\AwsS3V3Adapter; public function add() { if ($this->request->is('post')) { $picture = $this->request->getData('picture'); $filename = Text::slug($picture->getClientFilename()); $storage = StorageManager::get('s3'); // Get the S3 storage adapter $path = 'profiles/' . $filename; if ($storage->store($path, $picture->getStream())) { $this->Flash->success(__('Picture uploaded to S3.')); } else { $this->Flash->error(__('Unable to upload picture.')); } } }
With this code, the uploaded files will be stored in your configured Amazon S3 bucket.
Conclusion
CakePHP’s File Storage System offers a powerful way to manage files in web applications, whether you’re dealing with local storage or leveraging the capabilities of cloud storage providers like Amazon S3. By abstracting the underlying complexities, CakePHP allows developers to focus on building robust features without getting bogged down in file management intricacies. Whether you’re building a simple upload mechanism or implementing a comprehensive cloud-backed storage solution, CakePHP has you covered.
In this article, we’ve covered the basics of setting up and using CakePHP’s File Storage System for both local and cloud-based storage scenarios. Armed with this knowledge, you can confidently handle various file-related tasks in your CakePHP projects.
Remember that while we’ve explored local and Amazon S3 storage here, CakePHP’s File Storage System supports various other storage backends, allowing you to choose the one that best suits your application’s needs. So go ahead, experiment, and take your CakePHP applications to the next level with efficient and organized file management.
Table of Contents
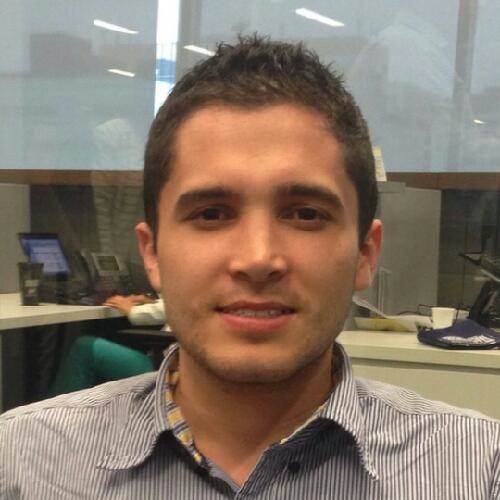
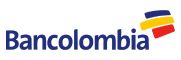