CakePHP and Microservices: Building a Distributed Architecture
In the ever-evolving landscape of web development, creating applications that are scalable, maintainable, and efficient is a top priority. One approach that has gained significant traction in recent years is the adoption of microservices architecture. Microservices allow you to break down complex applications into smaller, independently deployable services, promoting flexibility and agility in your development process. In this blog, we will explore how CakePHP, a popular PHP framework, can be used to build a distributed architecture based on microservices. We’ll delve into the core concepts, best practices, and provide code samples to help you get started on your journey towards creating robust, scalable web applications.
Table of Contents
1. Understanding Microservices
1.1. What Are Microservices?
Microservices are an architectural style that structures an application as a collection of small, loosely coupled services, each running in its own process and communicating with lightweight mechanisms like HTTP or message queues. These services are designed to be independently deployable and maintainable, allowing developers to work on specific components of the application without affecting the entire system.
1.2. Advantages of Microservices
- Scalability: Microservices enable you to scale individual components of your application independently, ensuring efficient resource utilization.
- Flexibility: Developers can choose the most suitable technology stack for each microservice, promoting innovation and adaptability.
- Resilience: Isolating services means that failures in one service won’t bring down the entire application.
- Easy Maintenance: Smaller codebases are easier to manage and update, reducing the complexity of maintenance.
Now that we have a basic understanding of microservices, let’s explore how CakePHP can be used to build a distributed architecture following microservices principles.
2. CakePHP: A Brief Overview
2.1. What is CakePHP?
CakePHP is a popular PHP web application framework known for its simplicity, flexibility, and convention over configuration (CoC) principles. It provides a structured foundation for building web applications quickly and efficiently, making it an ideal choice for developing microservices.
2.2. Why CakePHP for Microservices?
- Rapid Development: CakePHP’s built-in features, such as ORM (Object-Relational Mapping) and scaffolding, accelerate development.
- Scalability: CakePHP applications can be divided into microservices, allowing for scalable and modular development.
- Community and Ecosystem: CakePHP has a vibrant community and a rich ecosystem of plugins and extensions.
Now, let’s dive into the steps to build a distributed architecture using CakePHP and microservices.
3. Building Microservices with CakePHP
Step 1: Define Your Microservices
Before you start coding, it’s essential to define the boundaries of your microservices. Identify the specific functionalities or components that can be encapsulated into individual services. For example, in an e-commerce application, you might have microservices for user authentication, product catalog, and order processing.
Step 2: Create Independent CakePHP Projects
Each microservice should be a separate CakePHP project. You can create these projects using the CakePHP CLI (Command Line Interface). Here’s an example of how to create a new CakePHP project:
bash composer create-project --prefer-dist cakephp/app my-microservice
Repeat this step for each microservice you defined in the previous step.
Step 3: Define APIs for Communication
Microservices communicate with each other through APIs (Application Programming Interfaces). Design clear and well-documented APIs that allow services to exchange data seamlessly. CakePHP provides powerful tools for building RESTful APIs, making it an excellent choice for this purpose.
Here’s an example of defining a simple API endpoint in CakePHP:
php // In your CakePHP microservice controller public function getProduct($id) { $product = $this->Products->get($id); $this->set([ 'product' => $product, '_serialize' => ['product'], ]); }
Step 4: Use Message Queues for Asynchronous Communication
Microservices often need to communicate asynchronously to improve responsiveness and scalability. Utilize message queues like RabbitMQ or Apache Kafka to implement asynchronous communication between services. CakePHP can be integrated with these message queues to facilitate seamless data flow.
php // Publishing a message to a RabbitMQ queue in CakePHP $this->loadComponent('Queue.Queue', [ 'engine' => 'QueueRabbitMQ', 'connection' => 'default', ]); $this->Queue->push('your_queue_name', $data);
Step 5: Implement Authentication and Authorization
Securing microservices is crucial to prevent unauthorized access. CakePHP provides robust authentication and authorization mechanisms out of the box. You can use components like AuthComponent and AclComponent to enforce security policies for your microservices.
php // Example of using AuthComponent in CakePHP $this->loadComponent('Auth', [ 'authorize' => 'Controller', 'authenticate' => [ 'Basic' => [ 'fields' => ['username' => 'email', 'password' => 'password'], 'userModel' => 'Users', ], ], ]);
Step 6: Implement Service Discovery and Load Balancing
As your microservices grow, managing their endpoints becomes challenging. Implement service discovery and load balancing to automate the process of locating and distributing requests to the appropriate services. Tools like Consul and Kubernetes can help achieve this.
Step 7: Monitor and Log Your Microservices
Monitoring and logging are critical for maintaining the health and performance of your microservices. Use tools like Prometheus for monitoring and ELK (Elasticsearch, Logstash, Kibana) for centralized logging.
Now that we’ve outlined the steps to build microservices with CakePHP, let’s address some best practices to ensure the success of your distributed architecture.
4. Best Practices for CakePHP Microservices
4.1. Maintain Strong Data Consistency
When dealing with distributed data, maintaining consistency can be challenging. Use techniques like two-phase commits or event sourcing to ensure data consistency across microservices.
4.2. Version Your APIs
APIs are the contract between your microservices. Always version your APIs to prevent breaking changes from disrupting other services. Consider using semantic versioning (e.g., v1, v2) to indicate changes.
4.3. Implement Circuit Breaker Patterns
To handle service failures gracefully, implement circuit breaker patterns in your microservices. Tools like Hystrix can help you achieve this.
4.4. Automate Testing and Deployment
Embrace automated testing and deployment pipelines to ensure the reliability of your microservices. Tools like Jenkins or GitLab CI/CD can automate these processes.
4.5. Document Your Microservices
Comprehensive documentation is crucial for developers who need to work with your microservices. Use tools like Swagger or OpenAPI to generate documentation for your APIs.
Conclusion
CakePHP and microservices make a formidable combination for building distributed architectures that are scalable, maintainable, and efficient. By following the steps outlined in this blog and adhering to best practices, you can leverage the power of CakePHP to create a robust ecosystem of microservices that work seamlessly together.
Remember that the journey towards a distributed architecture is ongoing, and you should continuously monitor, optimize, and evolve your microservices as your application grows. With CakePHP as your foundation, you’re well-equipped to tackle the challenges and reap the benefits of a microservices-based approach to web development. So, roll up your sleeves, start coding, and embark on the path to building a distributed architecture that will empower your web applications for years to come.
Table of Contents
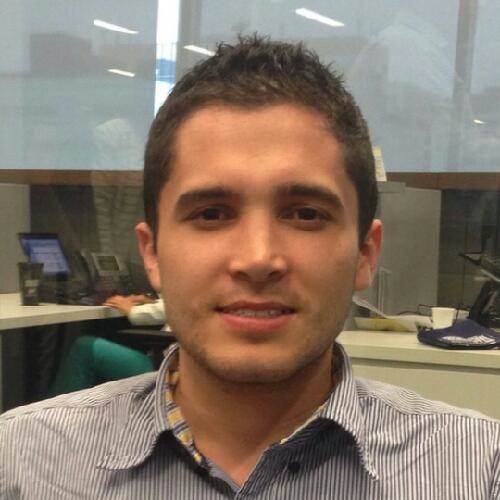
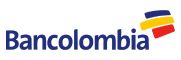