Exploring CakePHP’s Model-View-Controller (MVC) Architecture
In the world of web development, having a solid architectural foundation is crucial for building robust and maintainable applications. One popular architectural pattern used in many PHP frameworks is the Model-View-Controller (MVC) architecture. CakePHP, a powerful and flexible PHP framework, follows the MVC pattern, making it a popular choice for developers. In this blog post, we will dive deep into CakePHP’s MVC architecture, exploring its key components and benefits. Whether you are new to CakePHP or an experienced developer, this article will provide you with valuable insights and code samples to help you leverage the power of CakePHP’s MVC architecture effectively.
1. Understanding the Model-View-Controller (MVC) Pattern:
The Model-View-Controller (MVC) pattern is an architectural design pattern that separates an application into three distinct components: the Model, the View, and the Controller. Each component has a specific responsibility:
The Model represents the application’s data and business logic.
The View is responsible for presenting the data to the user in a user-friendly format.
The Controller handles user requests, manipulates the model’s data, and updates the view accordingly.
2. CakePHP’s Implementation of MVC:
CakePHP follows the traditional MVC pattern, providing a structured approach to web application development. Let’s take a closer look at each component in CakePHP’s implementation of MVC:
2.1. Model:
The Model in CakePHP is responsible for managing data and the business logic associated with it. It interacts with the database, performs data validations, and encapsulates the application’s data-related operations. CakePHP provides a set of conventions that make it easy to define and work with models. For example, a User model will typically have a corresponding “users” database table and a User class file.
2.2. View:
The View component in CakePHP is responsible for rendering the output and presenting it to the user. It takes the data from the controller and formats it into a user-friendly representation, such as HTML or JSON. Views in CakePHP are usually associated with a specific action in the controller and have corresponding template files for rendering the output.
2.3. Controller:
The Controller acts as an intermediary between the Model and the View. It receives user requests, interacts with the Model to fetch or manipulate data, and updates the View accordingly. Controllers in CakePHP are responsible for handling incoming requests, making decisions based on the request data, and managing the flow of the application. They serve as the glue that connects the Model and the View together.
3. The Benefits of CakePHP’s MVC Architecture:
CakePHP’s MVC architecture brings several benefits to the development process, making it a popular choice among developers. Let’s explore some of these advantages:
3.1. Separation of Concerns:
By separating the application into distinct components, CakePHP’s MVC architecture promotes the separation of concerns. Each component has a specific responsibility, making the codebase more organized and easier to maintain. Developers can focus on specific tasks without worrying about the entire application’s complexity.
3.2. Code Reusability:
CakePHP’s MVC architecture encourages code reusability. Models encapsulate the data-related operations, allowing developers to reuse the same model logic across different parts of the application. Similarly, Views can be reused across multiple actions or controllers, reducing code duplication and promoting efficiency.
3.3. Easy Maintenance and Testing:
With the clear separation between the Model, View, and Controller, maintaining and testing CakePHP applications becomes more manageable. Developers can modify or extend one component without affecting the others, reducing the risk of unintended side effects. It also facilitates unit testing as each component can be tested independently, ensuring the stability and reliability of the application.
4. CakePHP’s MVC in Action:
To better understand CakePHP’s MVC architecture, let’s walk through a simple example of creating a user management feature.
4.1. Creating a Model:
In CakePHP, creating a model is straightforward. Simply define a class that extends the Cake\ORM\Table class, representing the table in the database. You can define associations, validations, and custom business logic within the model.
php <?php // src/Model/Table/UsersTable.php namespace App\Model\Table; use Cake\ORM\Table; class UsersTable extends Table { // Define associations and other logic here }
4.2. Generating Views:
Views in CakePHP are associated with specific actions in the controller. You can generate views using CakePHP’s bake console tool or manually create them. Views are usually placed in the src/Template/ControllerName directory and have corresponding template files.
php <!-- src/Template/Users/index.ctp --> <h1>User List</h1> <table> <?php foreach ($users as $user): ?> <tr> <td><?php echo $user->name; ?></td> <td><?php echo $user->email; ?></td> </tr> <?php endforeach; ?> </table>
4.3. Handling Requests with Controllers:
Controllers handle user requests, interact with models, and update views accordingly. Here’s an example of a UsersController handling the index action, fetching users from the database, and passing them to the view.
php <?php // src/Controller/UsersController.php namespace App\Controller; use App\Controller\AppController; class UsersController extends AppController { public function index() { $users = $this->Users->find()->all(); $this->set(compact('users')); } }
Conclusion:
CakePHP’s Model-View-Controller (MVC) architecture provides a structured and efficient approach to web application development. By separating concerns and promoting code reusability, CakePHP simplifies the development process while ensuring easy maintenance and testing. Whether you are starting a new project or working on an existing one, leveraging CakePHP’s MVC architecture can significantly enhance your productivity and the quality of your applications. Happy coding!
Table of Contents
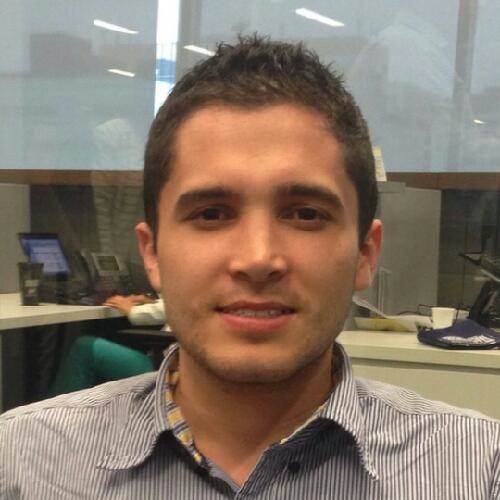
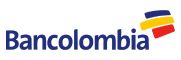