Optimizing CakePHP Queries: Improving Database Performance
When building web applications with CakePHP, database interactions play a crucial role in delivering data to users. However, inefficient database queries can significantly impact the application’s performance, resulting in slow page loads and degraded user experience. In this blog, we will explore various techniques to optimize CakePHP queries and improve database performance, ensuring your application runs smoothly even with a large user base.
1. Understanding the Importance of Database Optimization
Before diving into the optimization techniques, let’s understand why database performance is critical. A poorly optimized database can lead to:
- Slow Page Loads: Inefficient queries can cause the application to take longer to fetch and display data, leading to frustrating user experiences.
- Increased Server Load: Inadequate database optimization can overload the server, resulting in high CPU and memory usage.
- Scalability Issues: As your application grows and the database size increases, performance bottlenecks may become more evident.
2. Analyzing Queries with Debugging Tools
CakePHP provides built-in debugging tools that help analyze queries executed during the page load. These tools can be invaluable for identifying slow or repetitive queries that need optimization. To enable debugging, navigate to the config/app.php file and set the ‘debug’ value to true.
php // config/app.php 'debug' => true,
Once debugging is enabled, you can access the debug toolbar at the bottom of your application pages, which displays the number of queries executed, query execution time, and more. Use this information to identify the areas that require optimization.
3. Implementing Efficient Relationships
3.1 Use Containable Behavior:
When fetching associated data from multiple tables, CakePHP’s Containable behavior allows you to specify precisely which related data to retrieve, reducing unnecessary database joins and improving performance.
Example:
php // In your model public $actsAs = array('Containable'); // In your controller $this->Post->find('all', array( 'contain' => array('Comment') ));
3.2 Limit Fields in Queries:
Fetch only the required fields from the database instead of retrieving entire rows. This reduces the amount of data transferred from the database to the application, resulting in faster queries.
Example:
php // Fetch only 'title' and 'created' fields from the 'posts' table $this->Post->find('all', array( 'fields' => array('Post.title', 'Post.created') ));
4. Indexing for Improved Query Performance
4.1 Understanding Indexes:
Indexes are data structures that improve the speed of data retrieval operations on database tables. By creating appropriate indexes, you can significantly enhance the performance of read-heavy queries.
4.2 Choosing the Right Columns to Index:
Identify columns that are frequently used in the WHERE clause or involved in JOIN operations. These columns should be indexed for optimal performance.
4.3 Composite Indexes:
In some cases, a combination of columns might be frequently searched together. Creating a composite index on these columns can further improve query performance.
Example:
sql CREATE INDEX idx_user_email ON users (email); CREATE INDEX idx_user_status_created ON users (status, created);
5. Caching Database Queries
Caching is an effective technique to reduce the load on the database and improve the response time for repeated queries. CakePHP provides built-in caching support that allows you to cache query results for a specified duration.
5.1 Configure Caching:
Define the caching configuration in the config/app.php file. You can set up caching using various engines like File, Memcached, or Redis.
php // config/app.php 'Cache' => [ 'default' => [ 'className' => 'File', 'path' => CACHE, ], ],
5.2 Cache Query Results:
Use the cache option in your queries to enable caching for specific find operations.
Example:
php // Cache the query result for 1 hour $this->Post->find('all', array( 'cache' => [ 'key' => 'all_posts', 'duration' => '+1 hour', ], ));
6. Utilizing Paginator and Lazy Loading
6.1 Paginator:
When dealing with large datasets, CakePHP’s Paginator class enables you to retrieve data in smaller, manageable chunks. This prevents excessive memory usage and enhances overall application performance.
Example:
php // In your controller public $paginate = [ 'limit' => 10, ];
6.2 Lazy Loading:
Lazy loading allows you to load associated data only when needed, reducing the initial query’s complexity and enhancing performance.
Example:
php // In your controller $this->Post->contain('Comment'); // Associated data is not loaded yet // Somewhere else in the code $post = $this->Post->get($id); $comments = $post->comments; // Associated data is loaded here
7. Avoiding N+1 Query Problem
The N+1 query problem occurs when fetching multiple records and their associated data results in additional queries. This can be avoided using CakePHP’s contain and matching methods.
Example:
php // In your controller $posts = $this->Post->find('all') ->contain('Comment'); // OR using matching $posts = $this->Post->find('all') ->matching('Comment');
8. Using Raw Queries
For complex queries that cannot be easily optimized through CakePHP’s query builder, you can resort to using raw SQL queries.
Example:
php // In your controller $query = $this->Post->query("SELECT * FROM posts WHERE views > 1000"); $results = $query->fetchAll('assoc');
9. Regularly Update CakePHP and Database
Updating to the latest version of CakePHP ensures that you benefit from the latest performance optimizations and bug fixes. Additionally, keep your database up-to-date and consider periodic maintenance tasks, such as optimizing tables and rebuilding indexes.
Conclusion
Optimizing CakePHP queries and improving database performance is crucial for delivering a fast and responsive web application. By understanding the importance of database optimization, utilizing efficient relationships, indexing, caching, and other techniques, you can enhance your application’s performance and provide a better user experience. Regularly monitor and fine-tune your database performance to ensure that your application can handle increasing user demands. Happy optimizing!
Table of Contents
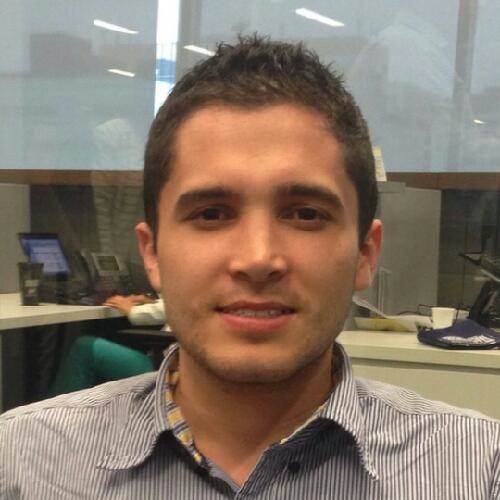
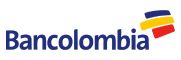