CakePHP Performance Optimization: Speeding Up Your Application
In the fast-paced world of web development, performance is paramount. A sluggish application can drive users away and negatively impact your business. When it comes to CakePHP, a popular PHP framework for building web applications, optimizing performance is crucial to ensure a seamless user experience. In this guide, we’ll explore various strategies and techniques to turbocharge your CakePHP application and deliver lightning-fast responses.
Table of Contents
1. Why Performance Optimization Matters
Before diving into the nitty-gritty of CakePHP performance optimization, let’s understand why it matters. A slow-loading website can frustrate users, leading to increased bounce rates and decreased user engagement. Additionally, search engines consider page speed as a ranking factor, affecting your site’s visibility in search results. In essence, optimizing your CakePHP application not only enhances user experience but also positively impacts your site’s overall success.
2. Caching for Rapid Responses
2.1. Leveraging CakePHP Caching
Caching is a tried-and-true technique for boosting performance. CakePHP offers built-in caching mechanisms that can significantly reduce the time taken to fetch and render data. By caching database queries, view templates, and other frequently accessed data, you can dramatically improve page load times.
CakePHP supports various caching methods, including File, Memcached, and Redis caching. Let’s take a look at an example of how to use File caching:
php // In your controller's action public function view($id) { $data = $this->loadModel('Article')->find('all', [ 'conditions' => ['Article.id' => $id], 'cache' => ['key' => 'article_' . $id, 'duration' => '1 hour'] ])->first(); $this->set(compact('data')); }
In this example, the queried data is cached using the cache option. The cache key includes the $id of the article, and the data will be cached for one hour.
2.2. HTTP Caching for Static Resources
Leverage browser-side caching by setting appropriate HTTP headers for static resources like CSS, JavaScript, and images. This allows clients to store these resources locally, reducing the need to fetch them from the server on subsequent visits. Implementing HTTP caching in CakePHP involves modifying your .htaccess file or using the CacheHelper.
php // In your view file echo $this->Html->css('styles.css', ['cache' => true]); echo $this->Html->script('script.js', ['cache' => true]);
3. Database Optimization
3.1. Efficient Querying with contain() and select()
CakePHP provides the contain() and select() methods to fine-tune your database queries. Use contain() to specify related models you want to retrieve alongside the main model. This prevents the infamous “N+1 query” problem where each related record triggers an additional query. Meanwhile, select() lets you retrieve only the columns you need, reducing the data transferred over the network.
php // In your controller $articles = $this->Articles->find() ->contain('Comments') ->select(['title', 'body']); $this->set(compact('articles'));
3.2. Indexing for Faster Retrieval
Proper indexing can significantly speed up database queries. Identify columns frequently used in WHERE, JOIN, and ORDER BY clauses and add indexes to them. CakePHP’s schema tool allows you to define indexes directly in your migration files.
php // In your migration file $this->table('articles') ->addColumn('title', 'string') ->addColumn('body', 'text') ->addIndex(['title', 'body']) ->create();
4. Optimize Frontend Assets
4.1. Minification and Concatenation
Reducing the size of your frontend assets, such as CSS and JavaScript files, can lead to faster page load times. Minification involves removing unnecessary whitespace, comments, and renaming variables to shorter names. Additionally, concatenating multiple files into one reduces the number of requests the browser needs to make.
Consider using tools like CakePHP’s AssetCompress plugin or external tools like Grunt or Webpack for efficient asset management.
php // In your layout file echo $this->Html->css('all_styles.min.css'); echo $this->Html->script('all_scripts.min.js');
4.2. Lazy Loading with AJAX
Lazy loading is an excellent technique for optimizing image-heavy pages. Images are loaded only when they come into the user’s viewport, saving bandwidth and improving initial page load times. You can implement lazy loading using JavaScript and AJAX calls.
javascript // Using jQuery for simplicity $(document).ready(function() { $('.lazy-load').each(function() { $(this).attr('src', $(this).data('src')); }); });
5. Server and Hosting Considerations
5.1. Utilize Content Delivery Networks (CDNs)
CDNs distribute your content across multiple servers located in different geographic regions. This reduces the physical distance between users and your server, resulting in faster content delivery. Integrate a CDN into your CakePHP application to serve static assets like images, stylesheets, and JavaScript files.
php // In your layout or view file echo $this->Html->image('image.jpg', ['fullBase' => true]);
5.2. Choose a Performance-Optimized Hosting Provider
Your choice of hosting provider can significantly impact your CakePHP application’s performance. Opt for a hosting provider that offers high-performance servers, solid-state drives (SSDs), and server-side caching solutions. Research user reviews and benchmark tests to find the best-suited provider for your needs.
6. Profiling and Monitoring
6.1. Identify Performance Bottlenecks
Regularly profile your CakePHP application to identify performance bottlenecks. Tools like Xdebug or Blackfire can help you pinpoint slow code segments, excessive database queries, and memory usage. Addressing these issues can lead to substantial performance improvements.
6.2. Monitor Application Health
Implement monitoring tools to keep track of your application’s health and performance over time. Services like New Relic, Datadog, or custom solutions can provide insights into server response times, error rates, and resource usage. Monitoring allows you to detect and resolve issues proactively before they impact users.
Conclusion
Optimizing the performance of your CakePHP application is not just a technical necessity but a strategic advantage. By implementing caching strategies, fine-tuning database queries, optimizing frontend assets, and making informed server and hosting decisions, you can deliver a seamless and lightning-fast user experience. Remember, performance optimization is an ongoing process, so regularly review and refine your strategies to ensure your CakePHP application remains at peak performance.
With these proven techniques in your toolkit, you’re well-equipped to supercharge your CakePHP application and provide users with an exceptional online journey.
Remember, a faster application leads to happier users and better business outcomes. So, start implementing these performance optimization techniques today and watch your CakePHP application reach new levels of speed and efficiency!
Table of Contents
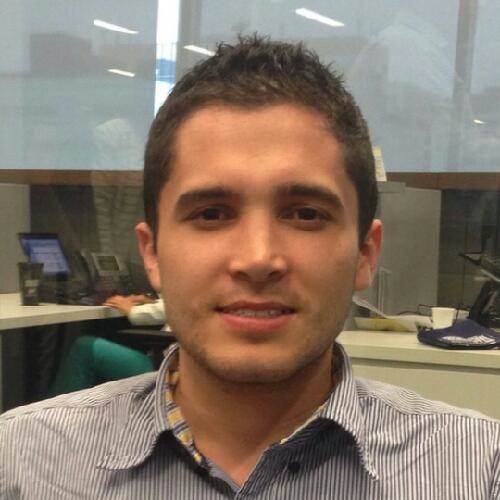
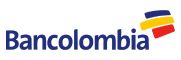