Exploring CakePHP’s Plugin Development Workflow
In the world of web development, frameworks play a pivotal role in simplifying and streamlining the process of building robust applications. CakePHP, a popular PHP framework, offers a range of tools and features that aid developers in creating efficient and maintainable web applications. One of the standout features of CakePHP is its plugin system, which allows developers to extend application functionality in a modular and organized manner. In this guide, we’ll delve into the intricacies of CakePHP’s plugin development workflow, from understanding the concept of plugins to implementing them effectively in your projects.
Table of Contents
1. Understanding CakePHP Plugins
Before we dive into the nitty-gritty of plugin development, let’s establish a clear understanding of what plugins are. In the context of CakePHP, a plugin is essentially a standalone module that encapsulates specific functionality or features. Think of plugins as building blocks that can be easily integrated into your CakePHP application to enhance its capabilities. These can range from simple components, helpers, and behaviors to more complex modules like authentication systems, caching mechanisms, and API integrations.
2. Benefits of Using CakePHP Plugins
Why should you bother with plugins in CakePHP? Here are some compelling reasons:
- Modularity: Plugins encourage a modular architecture, allowing you to compartmentalize distinct features of your application. This enhances maintainability and makes it easier to isolate and fix issues.
- Code Reusability: By creating plugins, you can reuse code across multiple projects. This saves time and effort, as well as promotes consistent practices.
- Isolation of Concerns: Plugins help maintain separation of concerns, keeping your codebase organized and promoting a cleaner development process.
- Collaboration: Plugins facilitate collaboration among developers by providing a standardized way to share and integrate features without affecting the main application.
3. Creating a CakePHP Plugin
Let’s walk through the steps involved in creating a basic CakePHP plugin:
Step 1: Generating the Plugin Structure
CakePHP provides a built-in console utility that simplifies the creation of plugins. Open your terminal and navigate to your project’s root directory. Use the following command to generate a new plugin named “ExamplePlugin”:
bash bin/cake bake plugin ExamplePlugin
This command creates the necessary directory structure and files for your plugin inside the plugins directory.
Step 2: Defining Plugin Configuration
Every plugin needs a configuration file to define its basic settings. Locate the config/bootstrap.php file within your plugin directory and configure your plugin by specifying routes, event listeners, and any other necessary settings.
php // plugins/ExamplePlugin/config/bootstrap.php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::plugin('ExamplePlugin', function (RouteBuilder $routes) { $routes->connect('/example', ['controller' => 'Examples', 'action' => 'index']); });
Step 3: Adding Functionality
To add functionality to your plugin, create controllers, models, views, and other necessary files within the respective directories of your plugin. For instance, to add a controller that displays a list of examples:
bash bin/cake bake controller Examples
This generates a controller file in the plugins/ExamplePlugin/src/Controller directory. Customize the actions and views as needed.
Step 4: Integrating the Plugin
Once your plugin is ready, integrate it into your main CakePHP application. In your main application’s config/bootstrap.php file, load the plugin using the Cake\Core\Plugin::load() function:
php // config/bootstrap.php Plugin::load('ExamplePlugin', ['bootstrap' => true, 'routes' => true]);
4. Best Practices for CakePHP Plugin Development
While creating plugins in CakePHP, it’s important to follow best practices to ensure code quality, maintainability, and compatibility. Here are some tips:
- Namespace Usage: Always use appropriate namespaces to prevent naming conflicts with other plugins or components.
- Clear Documentation: Document your plugin’s features, usage instructions, and any configuration options. This helps other developers (and your future self) understand and use the plugin effectively.
- Testing: Write unit tests for your plugin to ensure its functionality remains intact as your application evolves.
- Versioning: Implement versioning for your plugins, especially if you plan to release updates. This ensures compatibility and smooth upgrades for users.
- Dependency Management: Clearly define and manage dependencies to prevent unexpected issues when integrating your plugin with various projects.
Conclusion
CakePHP’s plugin development workflow opens up a world of possibilities for enhancing your applications with modular and reusable components. By creating well-structured plugins, you can streamline development, promote code reusability, and collaborate seamlessly with other developers. Remember to adhere to best practices and maintain clear documentation to ensure your plugins are easy to use and contribute to the overall success of your CakePHP projects. So, go ahead and explore the power of plugins to take your CakePHP applications to the next level of functionality and maintainability.
Table of Contents
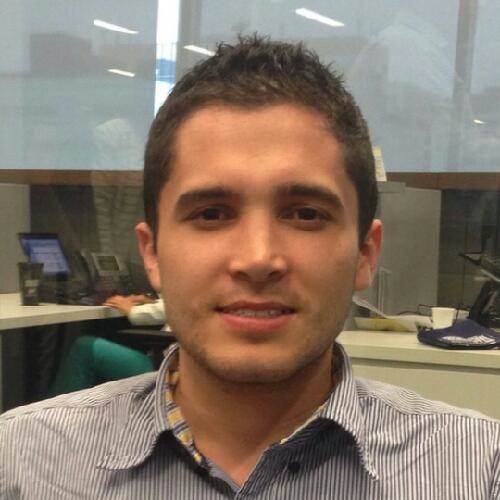
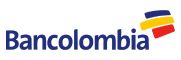