Implementing GraphQL in CakePHP: Query Language Integration
CakePHP, a popular PHP framework, provides developers with a robust foundation for building web applications. However, as applications grow in complexity, the need for efficient data querying and retrieval becomes increasingly important. GraphQL, a query language for your API, offers a powerful solution to this challenge. In this blog post, we’ll explore how to integrate GraphQL into your CakePHP project, enabling you to harness the benefits of flexible and optimized data querying.
Table of Contents
1. Why GraphQL in CakePHP?
Before diving into the implementation details, let’s briefly explore why GraphQL is a valuable addition to your CakePHP application.
1.1. Efficient Data Fetching
Traditional RESTful APIs often suffer from over-fetching or under-fetching of data. With GraphQL, clients can request precisely the data they need, eliminating unnecessary network requests and reducing the load on your server.
1.2. Reduced Versioning Complexity
In REST APIs, versioning is often necessary to avoid breaking client applications when the API evolves. GraphQL eliminates the need for versioning by allowing clients to specify the structure of the response they expect.
1.3. Faster Development
GraphQL provides a clear and self-documenting schema, which makes it easier for frontend and backend teams to collaborate. This can lead to faster development cycles and fewer misunderstandings.
Now that we’ve established the benefits of GraphQL, let’s walk through the process of integrating it into your CakePHP project.
2. Prerequisites
Before we begin, ensure that you have the following prerequisites in place:
- CakePHP Project: You should have an existing CakePHP project to integrate GraphQL into.
- Composer: Make sure Composer is installed on your system. You’ll need it to manage dependencies.
- Basic Knowledge of GraphQL: Familiarize yourself with the basics of GraphQL, such as queries, mutations, and schemas.
Step 1: Install Required Dependencies
To get started with GraphQL in CakePHP, you’ll need to install some dependencies. Open your terminal and navigate to your CakePHP project’s root directory. Then, use Composer to add the required packages:
bash composer require webonyx/graphql-php composer require cakephp/plugin-installer composer require cakephp/graphql
The webonyx/graphql-php package is a PHP implementation of GraphQL, while cakephp/graphql provides the integration with CakePHP.
Step 2: Create GraphQL Schema
In GraphQL, the schema defines the types of data that can be queried and the structure of those queries. To create a schema for your CakePHP project, you’ll need to define the types and queries specific to your application.
Let’s create a basic schema example for a blog application:
php // config/graphql/schema.graphql type Post { id: ID! title: String! content: String! } type Query { post(id: ID!): Post allPosts: [Post] }
In this example, we’ve defined a Post type with fields for id, title, and content. We’ve also created two queries: post to fetch a single post by ID and allPosts to fetch all posts.
Step 3: Configure Routes
To enable GraphQL queries in your CakePHP application, you need to configure routes. Open your config/routes.php file and add the following code:
php // config/routes.php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::plugin( 'GraphQL', ['path' => '/graphql'], function (RouteBuilder $routes) { // Register the GraphQL endpoint $routes->connect('/', ['controller' => 'GraphQL', 'action' => 'index'] ); } );
This code sets up a route for the GraphQL endpoint, making it accessible at /graphql.
Step 4: Create a GraphQL Controller
Next, you’ll need to create a GraphQL controller to handle incoming GraphQL queries. Generate the controller using CakePHP’s CLI:
bash bin/cake bake controller GraphQL
This command will create a GraphQLController.php file in your src/Controller directory.
Now, let’s implement the controller action to handle GraphQL queries:
php // src/Controller/GraphQLController.php use Cake\Controller\Controller; use Cake\Event\EventInterface; class GraphQLController extends Controller { public function index() { // Get the GraphQL schema $schema = include ROOT . '/config/graphql/schema.graphql'; // Handle the GraphQL request $data = $this->request->input('json_decode', true); $result = \GraphQL\GraphQL::executeQuery( $schema, $data['query'], null, null, $data['variables'] )->toArray(); $this->set([ 'data' => $result['data'], '_serialize' => ['data'], ]); } }
In this controller action, we retrieve the GraphQL schema, parse the incoming JSON request, and use the webonyx/graphql-php library to execute the query against the schema. The query, variables, and result are then set in the controller’s response.
Step 5: Testing Your GraphQL Endpoint
With everything set up, it’s time to test your GraphQL endpoint. You can use tools like GraphQL Playground or Apollo Studio to interact with your API.
Access the GraphQL Playground by visiting http://your-cakephp-app-url/graphql. You can now write and test GraphQL queries against your CakePHP application.
Step 6: Securing Your GraphQL Endpoint
GraphQL endpoints should be secure to prevent unauthorized access and potential abuse. You can implement authentication and authorization mechanisms like JWT (JSON Web Tokens) or OAuth2. Additionally, you can use CakePHP’s built-in authentication and authorization components to protect your GraphQL endpoint.
Step 7: Optimizing Queries
One of the advantages of GraphQL is the ability to optimize queries to reduce the risk of over-fetching or under-fetching data. You can implement data loader patterns to efficiently fetch related data and avoid the N+1 query problem.
Here’s a simplified example of a DataLoader for fetching related posts in the Post type:
php // src/GraphQL/DataLoader/PostDataLoader.php namespace App\GraphQL\DataLoader; use Cake\ORM\TableRegistry; use GraphQL\Executor\Promise\Promise; use GraphQL\Executor\Promise\PromiseAdapter; class PostDataLoader { private $promiseAdapter; public function __construct(PromiseAdapter $promiseAdapter) { $this->promiseAdapter = $promiseAdapter; } public function batchLoad($keys) { // Load posts by IDs $posts = TableRegistry::getTableLocator()->get('Posts') ->find() ->where(['Posts.id IN' => $keys]) ->toArray(); // Group posts by ID $postMap = []; foreach ($posts as $post) { $postMap[$post->id] = $post; } // Create promises for each key $promises = []; foreach ($keys as $key) { $promises[$key] = $this->promiseAdapter->createFulfilled($postMap[$key] ?? null); } return $promises; } }
In this DataLoader, we batch-load posts by their IDs, avoiding the N+1 query problem. You can extend this pattern to load related data efficiently.
Conclusion
Integrating GraphQL into your CakePHP application empowers you to build more efficient and flexible APIs. By following the steps outlined in this guide, you can seamlessly add GraphQL support to your project, enabling clients to request exactly the data they need while reducing the complexity of versioning.
To further enhance your GraphQL implementation, consider adding features like authentication, authorization, and query optimization. With GraphQL, you can provide a robust and user-friendly API experience for your application’s users and developers.
Now that you’ve learned how to integrate GraphQL into CakePHP, start experimenting and adapting it to your specific project’s needs. Happy coding!
Table of Contents
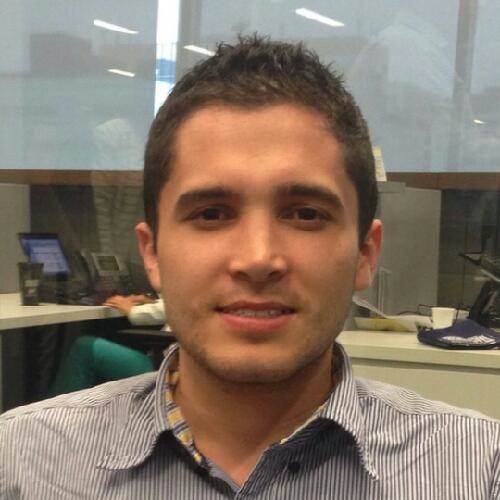
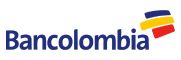