Implementing Real-Time Chat with CakePHP and Pusher
In today’s fast-paced digital landscape, real-time communication has become a cornerstone of user engagement and interaction. Whether it’s in a social networking app, an e-commerce platform, or a collaborative tool, integrating real-time chat features can significantly enhance user experience. In this tutorial, we will explore how to implement real-time chat functionality into a CakePHP application using Pusher, a powerful real-time communication platform.
Table of Contents
1. Prerequisites
Before we dive into the implementation, let’s make sure we have everything set up:
- Basic knowledge of CakePHP: Familiarity with CakePHP’s structure and concepts will be helpful.
- CakePHP Project: Have a CakePHP project up and running.
- Pusher Account: Sign up for a Pusher account (free tier available).
- Composer: Make sure you have Composer installed to manage CakePHP dependencies.
2. Setting Up Pusher
Pusher simplifies the process of adding real-time functionality to your application. It provides libraries for various programming languages, including JavaScript, which we’ll use for the client-side integration. Here’s how you can set up Pusher for your CakePHP application:
- Create Pusher Account: Sign up for a Pusher account at pusher.com if you haven’t already.
- Create a New App: After logging in to your Pusher dashboard, create a new app. This will provide you with credentials required for integration.
- Get Credentials: From your app’s dashboard, you will find the app_id, key, secret, and cluster. Keep these handy, as we’ll need them soon.
3. Implementation Steps
Now that we have Pusher set up, let’s proceed with implementing real-time chat functionality in our CakePHP application.
Step 1: Installing the Pusher PHP Library
We’ll start by installing the Pusher PHP library using Composer. Open your terminal and navigate to your CakePHP project directory. Run the following command:
bash composer require pusher/pusher-php-server
This command will install the Pusher PHP library in your project, allowing you to interact with Pusher’s server API.
Step 2: Configuring Pusher Credentials
Next, we need to configure Pusher credentials in our CakePHP application. Open the config/app.php file and add the following Pusher configuration in the ‘Datasources’ section:
php 'Pusher' => [ 'app_id' => 'YOUR_APP_ID', 'key' => 'YOUR_KEY', 'secret' => 'YOUR_SECRET', 'cluster' => 'YOUR_CLUSTER', 'useTLS' => true, ],
Replace YOUR_APP_ID, YOUR_KEY, YOUR_SECRET, and YOUR_CLUSTER with the credentials you obtained from your Pusher app’s dashboard.
Step 3: Creating the Chat Table
In this tutorial, we’ll create a simple chat system between users. We need a database table to store chat messages. Run the following CakePHP command to generate a migration for the chat table:
bash bin/cake bake migration CreateChat
Open the generated migration file in config/Migrations and define the structure of the chats table. For example:
php public function change() { $table = $this->table('chats'); $table->addColumn('user_id', 'integer') ->addColumn('message', 'text') ->addColumn('created', 'datetime') ->create(); }
Run the migration to create the table:
bash bin/cake migrations migrate
Step 4: Creating the Chat Model and Controller
Generate a model and a controller for the chat:
bash bin/cake bake model Chat bin/cake bake controller Chat
Step 5: Updating Routes
Open config/routes.php and add a new route for the chat:
php Router::scope('/', function (RouteBuilder $routes) { // Other routes $routes->connect('/chat', ['controller' => 'Chat', 'action' => 'index']); });
Step 6: Implementing the Chat Interface
Now comes the exciting part: creating the chat interface! Let’s create the necessary views and templates.
Create Views: In your src/Template directory, create a new directory named Chat if it doesn’t exist. Inside the Chat directory, add the following files:
index.ctp: The main chat interface.
element/message.ctp: An element to render chat messages.
Coding the Views: The index.ctp file will include a form to send messages and a section to display messages using the message.ctp element.
index.ctp:
php <h1>Real-Time Chat</h1> <div id="chat"> <div id="messages"> <?= $this->element('message', ['messages' => $messages]) ?> </div> <div id="input"> <?= $this->Form->create(null, ['id' => 'chat-form']) ?> <?= $this->Form->textarea('message', ['id' => 'message-field']) ?> <?= $this->Form->button('Send', ['id' => 'send-button']) ?> <?= $this->Form->end() ?> </div> </div> <script> // JavaScript code for sending and displaying messages </script>
element/message.ctp:
php <?php foreach ($messages as $message): ?> <div class="message"> <strong><?= $message->user->username ?></strong> <p><?= h($message->message) ?></p> <span class="timestamp"><?= $message->created->format('H:i') ?></span> </div> <?php endforeach; ?>
Step 7: Implementing Real-Time Functionality with Pusher
To achieve real-time updates, we’ll use Pusher’s JavaScript library on the client side to send and receive messages in real time.
Include Pusher JavaScript Library: Add the following script tag to the <head> section of your src/Template/Layout/default.ctp file:
html <script src="https://js.pusher.com/7.0/pusher.min.js"></script>
JavaScript Code for Real-Time Updates: Add the following JavaScript code to your index.ctp view:
javascript <script> // Initialize Pusher const pusher = new Pusher('YOUR_PUSHER_KEY', { cluster: 'YOUR_PUSHER_CLUSTER', encrypted: true, }); // Subscribe to the chat channel const channel = pusher.subscribe('chat'); // Listen for new messages channel.bind('new-message', function(data) { const messageContainer = document.getElementById('messages'); const messageElement = document.createElement('div'); messageElement.className = 'message'; messageElement.innerHTML = ` <strong>${data.username}</strong> <p>${data.message}</p> <span class="timestamp">${data.timestamp}</span> `; messageContainer.appendChild(messageElement); }); // Send message using AJAX const sendMessage = () => { const message = document.getElementById('message-field').value; if (message.trim() !== '') { const xhr = new XMLHttpRequest(); xhr.open('POST', '<?= $this->Url->build(['controller' => 'Chat', 'action' => 'send']) ?>'); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.send(`message=${encodeURIComponent(message)}`); document.getElementById('message-field').value = ''; } }; document.getElementById('chat-form').addEventListener('submit', function(event) { event.preventDefault(); sendMessage(); }); document.getElementById('send-button').addEventListener('click', function() { sendMessage(); }); </script>
Step 8: Processing and Broadcasting Messages
Update the ChatController to handle message sending and broadcasting:
src/Controller/ChatController.php:
php use Cake\Event\EventInterface; use Pusher\Pusher; class ChatController extends AppController { public function index() { // Retrieve existing messages $messages = $this->Chat->find('all') ->contain(['Users']) ->order(['created' => 'ASC']) ->limit(50) ->toArray(); $this->set(compact('messages')); } public function send() { if ($this->request->is('ajax')) { $messageText = $this->request->getData('message'); if (!empty($messageText)) { $message = $this->Chat->newEntity([ 'user_id' => $this->Auth->user('id'), 'message' => $messageText, ]); if ($this->Chat->save($message)) { $pusher = new Pusher( Configure::read('Pusher.key'), Configure::read('Pusher.secret'), Configure::read('Pusher.app_id'), ['cluster' => Configure::read('Pusher.cluster')] ); $pusher->trigger('chat', 'new-message', [ 'username' => $this->Auth->user('username'), 'message' => $messageText, 'timestamp' => date('H:i'), ]); $this->response = $this->response->withType('application/json') ->withStringBody(json_encode(['status' => 'success'])); } else { $this->response = $this->response->withStatus(400) ->withType('application/json') ->withStringBody(json_encode(['status' => 'error'])); } } else { $this->response = $this->response->withStatus(400) ->withType('application/json') ->withStringBody(json_encode(['status' => 'error'])); } } return $this->response; } }
Conclusion
Congratulations! You’ve successfully integrated real-time chat functionality into your CakePHP application using Pusher. This implementation provides a foundation for building interactive and engaging user experiences across various types of applications. With real-time communication, users can seamlessly exchange messages, enhancing collaboration and user engagement.
In this tutorial, we covered the key steps involved in implementing real-time chat, including setting up Pusher, configuring credentials, creating database tables, generating models and controllers, creating the chat interface, and implementing real-time updates using Pusher’s JavaScript library.
By following these steps and understanding the core concepts, you can easily adapt and extend this implementation to suit your specific application’s requirements and create a dynamic and responsive chat system.
Table of Contents
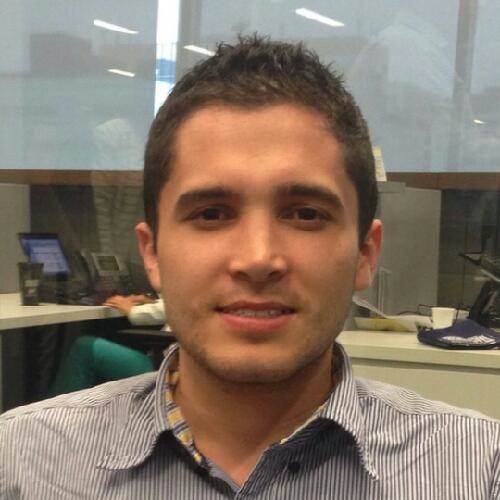
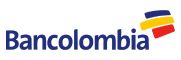