Building a Real-Time Dashboard with CakePHP and WebSockets
in today’s fast-paced digital world, having access to real-time data is crucial for making informed decisions. Whether you’re monitoring website traffic, tracking user activity, or analyzing system performance, a real-time dashboard can provide invaluable insights. In this tutorial, we will explore how to build a real-time dashboard using CakePHP, a popular PHP framework, and WebSockets, a technology that enables real-time communication between the server and client.
Table of Contents
1. Prerequisites
Before we dive into building our real-time dashboard, make sure you have the following prerequisites installed:
- PHP: You should have PHP installed on your development environment. You can download PHP from php.net.
- Composer: Composer is a dependency management tool for PHP. If you don’t have it installed, you can download it from getcomposer.org.
- Node.js and npm: You’ll need Node.js and npm to set up the front-end part of the application. You can download them from nodejs.org.
- CakePHP: We’ll use the CakePHP framework for the backend. You can install CakePHP via Composer by running the following command:
shell composer create-project --prefer-dist cakephp/app my_dashboard
Redis: Redis is a high-performance in-memory data store that we’ll use for Pub/Sub messaging in our WebSocket implementation. You can download Redis from redis.io.
- WebSocket Server: We’ll use Ratchet as our WebSocket server. You can install it via Composer:
shell composer require cboden/ratchet
2. Setting Up the CakePHP Application
2.1. Creating the Dashboard Controller
Our first step is to create a controller to handle dashboard-related functionality. In CakePHP, controllers are responsible for processing requests and generating responses. To create a dashboard controller, run the following command in your CakePHP project directory:
shell bin/cake bake controller Dashboard
This command will generate a DashboardController.php file in the src/Controller directory.
2.2. Adding Real-Time Dashboard View
Now, let’s create a view for our real-time dashboard. In the src/Template/Dashboard directory, create a new file named index.ctp. This file will contain the HTML structure and JavaScript code needed for our dashboard.
php <!-- src/Template/Dashboard/index.ctp --> <!-- Include WebSocket client library --> <script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/4.0.1/socket.io.js"></script> <!-- Dashboard HTML --> <div id="dashboard"> <h1>Real-Time Dashboard</h1> <div id="data-container"> <!-- Real-time data will be displayed here --> </div> </div> <!-- JavaScript --> <script> // Connect to the WebSocket server const socket = io('ws://localhost:8080'); // Listen for real-time data updates socket.on('data-update', (data) => { // Update the dashboard with new data const dataContainer = document.getElementById('data-container'); dataContainer.innerHTML = `<p>New Data: ${data}</p>`; }); </script>
In this code snippet, we include the Socket.io library to enable WebSocket communication on the client side. We also create a container for displaying real-time data updates and set up a WebSocket connection to ws://localhost:8080. When new data arrives, we update the dashboard with the received data.
2.3. Creating WebSocket Server
Now, let’s create a WebSocket server using Ratchet. Create a new file named WebSocket.php in the src/Shell directory of your CakePHP project:
php // src/Shell/WebSocket.php use Ratchet\Http\HttpServer; use Ratchet\Server\IoServer; use Ratchet\WebSocket\WsServer; use MyApp\WebSocketHandler; // Replace with your WebSocket handler namespace require dirname(__DIR__) . '/vendor/autoload.php'; $server = IoServer::factory( new HttpServer( new WsServer( new WebSocketHandler() // Create your WebSocket handler class ) ), 8080 ); $server->run();
In this code, we set up a WebSocket server on port 8080 and specify a custom WebSocket handler class. You should replace MyApp\WebSocketHandler with the actual namespace and class name of your WebSocket handler.
2.4. Creating the WebSocket Handler
Next, let’s create the WebSocket handler class that will handle WebSocket connections and data updates. Create a new file named WebSocketHandler.php in the src directory of your CakePHP project:
php // src/WebSocketHandler.php namespace MyApp; use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class WebSocketHandler implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new \SplObjectStorage(); } public function onOpen(ConnectionInterface $conn) { // Store the new connection $this->clients->attach($conn); echo "New connection! ({$conn->resourceId})\n"; } public function onMessage(ConnectionInterface $from, $msg) { // Broadcast the message to all clients foreach ($this->clients as $client) { if ($client !== $from) { $client->send($msg); } } } public function onClose(ConnectionInterface $conn) { // Remove the connection when the client closes the connection $this->clients->detach($conn); echo "Connection {$conn->resourceId} has disconnected\n"; } public function onError(ConnectionInterface $conn, \Exception $e) { echo "An error occurred: {$e->getMessage()}\n"; $conn->close(); } }
This WebSocket handler class implements the MessageComponentInterface from Ratchet and handles WebSocket events such as opening a connection, receiving messages, closing a connection, and handling errors.
2.5. Starting the WebSocket Server
To start the WebSocket server, run the following command in your CakePHP project directory:
shell php src/Shell/WebSocket.php
This command will start the WebSocket server on port 8080. Make sure you keep this process running while testing the real-time dashboard.
3. Integrating Real-Time Updates
With the WebSocket server in place, it’s time to integrate real-time updates into your CakePHP application. We’ll modify the DashboardController.php to send real-time updates to connected clients.
3.1. Sending Real-Time Data
Open the src/Controller/DashboardController.php file and add the following code to send real-time data to connected clients:
php // src/Controller/DashboardController.php namespace App\Controller; use Cake\Controller\Controller; use Ratchet\WebSocket\MessageComponentInterface; class DashboardController extends Controller { // ... Other controller methods ... public function sendRealTimeData() { // Get the WebSocket handler instance $webSocketHandler = $this->loadWebSocketHandler(); // Send real-time data to connected clients $data = "Real-time data from CakePHP!"; foreach ($webSocketHandler->clients as $client) { $client->send($data); } $this->autoRender = false; // Disable view rendering } protected function loadWebSocketHandler(): MessageComponentInterface { // Load the WebSocket handler from the WebSocket server $websocketServer = 'ws://localhost:8080'; // WebSocket server URL $websocketHandlerClass = 'MyApp\WebSocketHandler'; // Replace with your WebSocket handler class $client = new \WebSocket\Client($websocketServer); $client->send(json_encode(['handler' => $websocketHandlerClass])); return $client->receive(); } }
In this code, we define a sendRealTimeData method that sends a message to all connected WebSocket clients. We use the loadWebSocketHandler method to establish a connection to the WebSocket server and retrieve the WebSocket handler instance. Make sure to replace ‘MyApp\WebSocketHandler’ with your actual WebSocket handler class.
3.2. Updating the WebSocket Connection
To ensure that your WebSocket connection is updated with the latest data, let’s add a button in the dashboard view to trigger the data update. Modify the src/Template/Dashboard/index.ctp file as follows:
php <!-- src/Template/Dashboard/index.ctp --> <!-- ... Previous HTML code ... --> <!-- Button to trigger real-time data update --> <button onclick="sendRealTimeData()">Update Data</button> <!-- JavaScript --> <script> // ... Previous JavaScript code ... // Function to send real-time data update request to CakePHP function sendRealTimeData() { fetch('/dashboard/sendRealTimeData') .then((response) => { if (!response.ok) { console.error('Error updating data:', response.status, response.statusText); } }) .catch((error) => { console.error('Fetch error:', error); }); } </script>
We’ve added a button with an onclick event that triggers the sendRealTimeData function, which makes an AJAX request to the sendRealTimeData action in the DashboardController.
4. Testing the Real-Time Dashboard
Now that we’ve implemented the real-time components of our dashboard, it’s time to test the application:
- Start your CakePHP server by running the following command in your project directory:
shell bin/cake server
2. This will start the CakePHP development server.
3. Start the WebSocket server in a separate terminal:
shell php src/Shell/WebSocket.php
4. Ensure that both servers are running without errors.
5. Open your web browser and navigate to http://localhost:8765/dashboard. You should see the real-time dashboard with a “Update Data” button.
6. Click the “Update Data” button, and you should see real-time data updates on the dashboard.
Congratulations! You’ve successfully built a real-time dashboard using CakePHP and WebSockets. This dynamic dashboard can be customized further to display real-time data relevant to your specific use case.
Conclusion
In this tutorial, we’ve learned how to create a real-time dashboard using CakePHP and WebSockets. We started by setting up the CakePHP application, creating a WebSocket server using Ratchet, and implementing the WebSocket handler. We then integrated real-time data updates into the CakePHP application, allowing us to send real-time data to connected clients and update the dashboard dynamically. Real-time dashboards are a powerful tool for monitoring and analyzing data, and with the knowledge gained from this tutorial, you can adapt and expand your dashboard to suit various real-time use cases. Happy coding!
Table of Contents
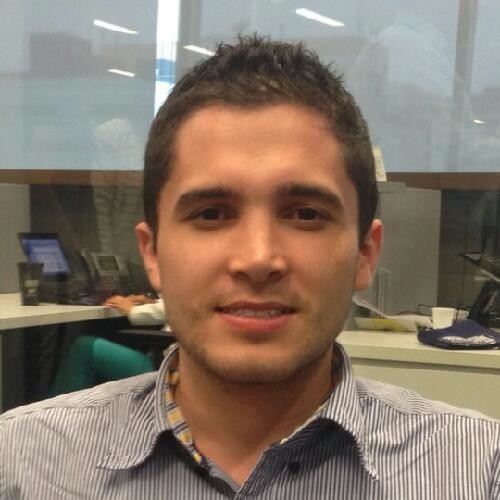
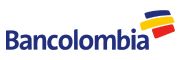