Implementing Real-Time Notifications in CakePHP
In today’s fast-paced digital landscape, providing users with real-time updates has become an essential aspect of user engagement and retention. Users expect to be informed promptly about relevant events, such as new messages, updates, or activities within an application. Implementing real-time notifications can greatly enhance the user experience and keep users engaged with your CakePHP application. In this blog post, we’ll explore how to implement real-time notifications in CakePHP using WebSocket technology, step by step.
Table of Contents
1. Understanding the Need for Real-Time Notifications
In a traditional web application, users have to manually refresh the page to check for updates or wait for a periodic polling mechanism. However, this approach is not only inefficient but also less user-friendly. Real-time notifications enable users to receive updates instantly as they occur, without the need for constant page refreshing. Whether it’s a social media platform notifying users about new messages, a project management tool updating users on task assignments, or an e-commerce site alerting users about order status changes, real-time notifications are crucial for enhancing user experience.
2. Introduction to WebSockets
WebSockets provide a full-duplex communication channel over a single TCP connection, allowing bidirectional communication between the client (browser) and the server. Unlike the traditional request-response cycle of HTTP, WebSockets allow the server to send data to the client whenever there’s new information available. This technology is perfect for implementing real-time features such as notifications, live chats, and collaborative editing.
3. Setting Up a CakePHP Project
Before we dive into implementing real-time notifications, make sure you have a CakePHP project up and running. You can either create a new project or use an existing one. If you’re new to CakePHP, their official documentation provides a comprehensive guide to getting started.
4. Installing the WebSocket Library
To implement real-time notifications in CakePHP, we’ll use the Ratchet library. Ratchet is a PHP library that makes it easy to create WebSocket applications. Begin by installing Ratchet using Composer, the dependency management tool for PHP.
bash composer require cboden/ratchet
5. Creating the Notification System
5.1. Database Setup
Start by setting up the database for storing notifications. CakePHP’s ORM makes it straightforward to manage database interactions. Configure your database connection in config/app.php if you haven’t already.
5.2. Creating the Notification Table
Run the following CakePHP shell command to generate a migration for the notification table:
bash bin/cake bake migration CreateNotifications
This command will create a migration file in the config/Migrations directory. Define the necessary fields for your notifications, such as id, user_id, message, created, etc. Run the migration to create the table:
bash bin/cake migrations migrate
5.3. Publishing Events
In your application’s logic where an event triggers a notification, publish the event to the WebSocket server. For example, when a user receives a new message:
php // In your controller or service $notification = $this->Notifications->newEntity([ 'user_id' => $recipientUserId, 'message' => 'You have a new message.' ]); if ($this->Notifications->save($notification)) { // Publish the event to WebSocket server $this->WebSocket->publish('new_notification', [ 'user_id' => $recipientUserId, 'message' => 'You have a new message.' ]); }
5.4. Receiving and Displaying Notifications
On the client side, use JavaScript to connect to the WebSocket server and listen for events. When a new notification event is received, update the user interface to display the notification to the user:
javascript const socket = new WebSocket('ws://your-websocket-server-url'); socket.addEventListener('message', event => { const data = JSON.parse(event.data); if (data.event === 'new_notification') { // Update UI with the new notification const notificationElement = document.createElement('div'); notificationElement.textContent = data.message; // Add the notification to the notifications container notificationsContainer.appendChild(notificationElement); } });
6. Implementing the WebSocket Server
6.1. Installing Ratchet
To create the WebSocket server, we’ll use the Ratchet library. Install it using Composer:
bash composer require cboden/ratchet
6.2. WebSocket Server Initialization
Create a new file named WebSocketServer.php in your CakePHP project’s src directory. Initialize the WebSocket server in this file:
php namespace App\Shell; use Cake\Console\Shell; use Ratchet\Http\HttpServer; use Ratchet\Server\IoServer; use Ratchet\WebSocket\WsServer; class WebSocketServerShell extends Shell { public function main() { $server = IoServer::factory( new HttpServer(new WsServer(new YourWebSocketApp())), 8080 ); $server->run(); } }
6.3. Broadcasting Notifications
In your YourWebSocketApp class, handle incoming WebSocket connections and broadcast events to connected clients:
php use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class YourWebSocketApp implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new \SplObjectStorage(); } public function onOpen(ConnectionInterface $conn) { $this->clients->attach($conn); } public function onMessage(ConnectionInterface $from, $msg) { // Handle incoming messages if needed } public function onClose(ConnectionInterface $conn) { $this->clients->detach($conn); } public function onError(ConnectionInterface $conn, \Exception $e) { $conn->close(); } // Broadcast a message to all connected clients public function broadcast($message) { foreach ($this->clients as $client) { $client->send($message); } } }
Conclusion
Implementing real-time notifications in your CakePHP application can significantly enhance user engagement and provide a seamless user experience. By leveraging WebSocket technology and the Ratchet library, you can keep users informed about relevant events as they happen. Users will appreciate the instant updates and responsiveness, leading to higher user satisfaction and retention. Remember to follow best practices for security and performance while implementing WebSocket-based features. Happy coding!
Table of Contents
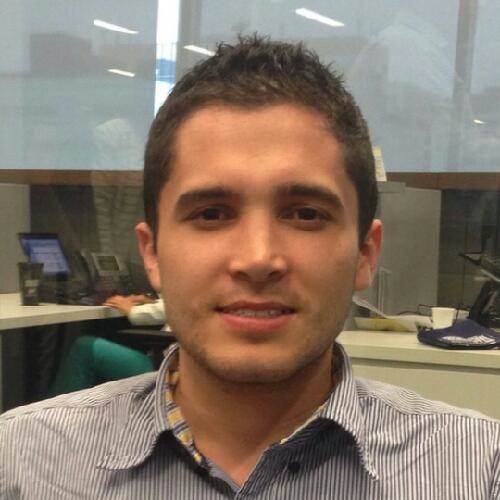
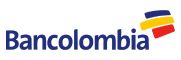