Implementing Real-Time Notifications in CakePHP with WebSockets
In today’s fast-paced digital world, user engagement and real-time interactions are crucial for the success of web applications. One effective way to enhance user engagement is by providing real-time notifications. These notifications can inform users about various events, such as new messages, friend requests, or updates to their content. In this blog post, we will explore how to implement real-time notifications in CakePHP using WebSockets, taking your CakePHP application to the next level of user experience.
Table of Contents
1. Why Real-Time Notifications?
Before we dive into the technical details, let’s understand why real-time notifications are so important for web applications.
1.1. Enhancing User Engagement
Real-time notifications provide instant updates to users, keeping them engaged and informed about relevant activities on your platform. This can lead to increased user satisfaction and retention.
1.2. Improving User Experience
Users no longer have to manually refresh the page to check for updates. Real-time notifications provide a seamless and dynamic user experience, making your application feel more modern and responsive.
1.3. Boosting Collaboration
In collaborative applications like messaging platforms or project management tools, real-time notifications are essential for keeping team members in sync. They enable quick responses and facilitate better communication.
Now that we understand the importance of real-time notifications, let’s explore how to implement them in CakePHP using WebSockets.
2. Prerequisites
Before we begin, make sure you have the following prerequisites in place:
- A CakePHP application (if you don’t have one, you can start with the official CakePHP tutorial).
- Basic knowledge of PHP and CakePHP.
- Node.js installed on your server or development environment.
3. Setting Up the WebSocket Server
To implement real-time notifications, we need a WebSocket server. We’ll use Socket.io, a popular library for building real-time applications. Here’s how to set it up:
3.1. Install Socket.io
First, navigate to your CakePHP project’s root directory and install Socket.io using npm:
bash npm install socket.io
3.2. Create a WebSocket Server
Next, create a WebSocket server script (e.g., websocket-server.js) in your CakePHP project directory:
javascript const http = require('http'); const io = require('socket.io')(http); const PORT = 3000; // Customize the port as needed io.on('connection', (socket) => { console.log('A user connected'); socket.on('disconnect', () => { console.log('User disconnected'); }); }); http.listen(PORT, () => { console.log(`WebSocket server is running on port ${PORT}`); });
This script sets up a basic WebSocket server using Socket.io. Users can connect and disconnect from this server, and you can handle events like user authentication and message broadcasting within the connection event.
3.3. Start the WebSocket Server
To start the WebSocket server, run the following command in your project directory:
bash node websocket-server.js
Your WebSocket server should now be up and running on the specified port (in this example, port 3000).
4. Integrating WebSocket with CakePHP
Now that we have our WebSocket server in place, let’s integrate it with our CakePHP application.
4.1. Include Socket.io Client Library
In your CakePHP application’s layout or specific view, include the Socket.io client library to enable communication between the client (your CakePHP app) and the WebSocket server:
html <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); </script>
4.2. Emit Events
You can emit events from your CakePHP application to the WebSocket server using the socket.emit method. For example, when a user receives a new message, you can emit a “new message” event:
javascript // In your CakePHP controller or view socket.emit('new message', { message: 'Hello, world!' });
4.3. Listen for Events
On the client side, you can listen for events emitted from the WebSocket server using socket.on. For instance, to handle incoming messages:
javascript // In your CakePHP view or JavaScript file socket.on('new message', (data) => { // Handle the new message here console.log('New message received:', data.message); });
By emitting and listening for events, you can implement real-time notifications for various use cases within your CakePHP application.
5. Practical Use Cases
Let’s explore some practical use cases for real-time notifications in CakePHP:
5.1. Chat Application
Enhance your CakePHP chat application by implementing real-time message notifications. When a user sends a message, all participants in the chat can instantly see the new message without refreshing the page.
5.2. Social Networking
In a social networking platform built with CakePHP, real-time notifications can notify users about friend requests, likes, comments, and mentions as soon as they happen.
5.3. Collaborative Tools
For project management or document collaboration tools, real-time notifications ensure that team members are instantly informed of updates, changes, or new comments related to their projects or documents.
6. Security Considerations
When implementing real-time notifications with WebSockets, security is paramount. Here are some important considerations:
6.1. Authentication
Ensure that your WebSocket server authenticates users properly before allowing them to connect. You can use middleware or session data from your CakePHP application to verify user identities.
6.2. Authorization
Implement authorization checks to ensure that users can only access the notifications relevant to them. For example, a user should only receive notifications about their own content or interactions.
6.3. Data Validation
Validate data sent through WebSocket events to prevent malicious payloads or attacks.
6.4. Rate Limiting
Implement rate limiting to prevent abuse or overloading of your WebSocket server. This can help maintain server performance and prevent resource exhaustion.
Conclusion
Real-time notifications using WebSockets can significantly enhance the user experience of your CakePHP application. By following the steps outlined in this blog post, you can create dynamic and engaging features that keep your users informed and engaged.
Remember to consider security best practices when implementing real-time notifications to ensure the safety of your application and its users. With the right approach, you can take your CakePHP application to the next level and provide a modern, responsive, and engaging experience for your users.
Table of Contents
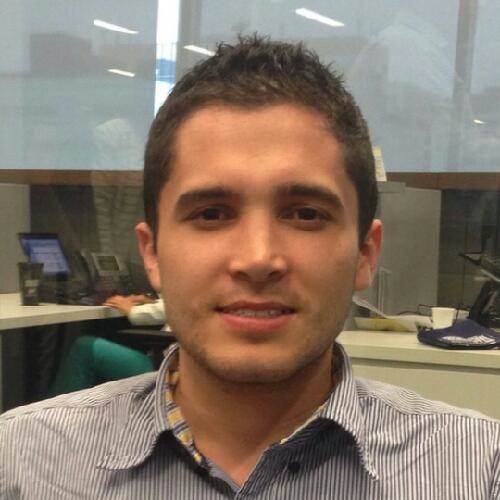
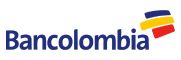