Using CakePHP’s Request and Response Objects: Handling HTTP
In the world of web development, handling HTTP requests and responses is a fundamental task. Efficiently processing incoming data, routing it to the appropriate controller actions, and generating proper responses are critical for a seamless user experience. CakePHP, a popular PHP framework, provides powerful tools for working with HTTP, particularly its Request and Response objects. In this blog post, we will explore how to leverage these objects to handle HTTP requests and responses effectively. From routing to data handling and beyond, let’s dive into the world of CakePHP’s Request and Response objects.
Table of Contents
1. Understanding the Basics:
1.1. What are HTTP Requests and Responses?
HTTP (Hypertext Transfer Protocol) is the foundation of data communication on the internet. Clients, typically web browsers, send HTTP requests to servers, and the servers respond with HTTP responses containing the requested data. Requests consist of methods like GET, POST, PUT, DELETE, etc., while responses carry the data back to the clients, accompanied by an HTTP status code indicating the outcome of the request.
1.2. Introducing CakePHP’s Request Object:
In CakePHP, the Request object represents an incoming HTTP request. It encapsulates all the information sent by the client, such as URL parameters, POST data, query strings, cookies, and more. CakePHP automatically populates the Request object, making it easily accessible throughout your application.
1.3. The Power of CakePHP’s Response Object:
On the other hand, the Response object in CakePHP allows you to construct and send HTTP responses back to the client. It enables you to set response headers, set the response body content, and manage other aspects of the response.
2. Handling HTTP Requests:
2.1. Routing Requests to Controller Actions:
The first step in handling HTTP requests is routing them to the appropriate controller action. CakePHP provides a flexible routing system that allows you to define custom routes to map URLs to specific controllers and actions. Let’s take a look at an example of defining a simple route:
php // app/config/routes.php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::scope('/', function (RouteBuilder $routes) { $routes->connect('/articles/:id', ['controller' => 'Articles', 'action' => 'view']) ->setPass(['id']); });
In this example, we define a route that maps URLs like “/articles/1” to the “view” action in the “Articles” controller, passing the “id” as a parameter.
2.2. Accessing Request Data:
Once a request is routed to a controller action, you can access the request data through the Request object. For example, to access query parameters:
php // ArticlesController.php public function view() { $articleId = $this->request->getQuery('id'); // ... }
2.3. Working with Query Parameters:
Query parameters are commonly used to pass data in the URL. In CakePHP, you can easily retrieve query parameters using the getQuery() method, as shown in the previous example. For URLs like “/articles/view?id=1&category=tech,” you can access the “category” parameter as follows:
php $category = $this->request->getQuery('category');
2.4. Handling POST Data:
When dealing with HTTP POST requests, you often need to access the submitted data. CakePHP makes it simple to retrieve POST data using the getData() method:
php // ArticlesController.php public function add() { if ($this->request->is('post')) { $data = $this->request->getData(); // Process the data... } }
2.5. Dealing with File Uploads:
Uploading files is a common task in web applications. When handling file uploads, you can access the files from the Request object. For example:
php // ArticlesController.php public function upload() { if ($this->request->is('post')) { $uploadedFile = $this->request->getData('file'); // Process the uploaded file... } }
3. Generating HTTP Responses:
3.1. Setting Response Headers:
Response headers provide important metadata about the response, such as content type, cache control, and cookies. Using the Response object, you can set custom headers easily:
php // ArticlesController.php public function view() { // Some code here... $this->response = $this->response->withHeader('X-Custom-Header', 'Hello'); }
3.2. Sending Different Response Types (JSON, XML, etc.):
CakePHP allows you to respond with various content types, such as JSON or XML. To send a JSON response:
php // ArticlesController.php public function api() { $data = ['message' => 'Success']; $this->response = $this->response->withType('application/json'); $this->response = $this->response->withStringBody(json_encode($data)); return $this->response; }
3.3. Rendering Views and Layouts:
In CakePHP, views are used to generate HTML content to be sent back as the response. You can use the render() method to generate a view:
php // ArticlesController.php public function view() { $article = $this->Article->get($id); $this->set('article', $article); $this->render('view'); }
3.4. Creating Custom Response Objects:
Sometimes, you may need to create custom response objects for unique use cases. For example, creating a custom response object to return a PDF file:
php // CustomPdfResponse.php use Cake\Http\Response; class CustomPdfResponse extends Response { public function withPdfContent(string $pdfContent) { return $this->withType('application/pdf') ->withStringBody($pdfContent); } }
4. Leveraging CakePHP’s Convenience Features:
4.1. Redirects and Flash Messages:
CakePHP provides simple methods to handle redirects and flash messages. For example, redirecting to another URL:
php // ArticlesController.php public function update($id) { // Update the article... return $this->redirect(['action' => 'view', $id]); }
4.2. Handling Errors and Exceptions:
In CakePHP, you can customize error handling for a more user-friendly experience. By extending the AppExceptionRenderer class, you can handle errors and exceptions according to your needs.
4.3. Caching Responses for Performance:
Caching responses can significantly improve the performance of your web application. CakePHP makes it easy to cache responses using various caching engines.
5. Best Practices for HTTP Handling:
5.1. Keeping Controllers Thin:
It’s essential to keep your controllers thin and move business logic into separate classes to maintain a clean and testable codebase.
5.2. Using Request and Response Objects in Plugins:
If you are developing CakePHP plugins, you can take advantage of the Request and Response objects for consistent and robust HTTP handling.
5.3. Writing Testable Code with Request and Response Mocks:
When writing tests, you can use mock objects for the Request and Response to simulate different scenarios and ensure proper functionality.
Conclusion
In this blog post, we explored the powerful features of CakePHP’s Request and Response objects for handling HTTP. From routing requests to controller actions and accessing data, to generating various responses and leveraging convenience features, CakePHP provides a robust foundation for building web applications with ease. By following best practices and utilizing these tools effectively, you can ensure your web applications are efficient, maintainable, and deliver an exceptional user experience. Happy coding with CakePHP!
Table of Contents
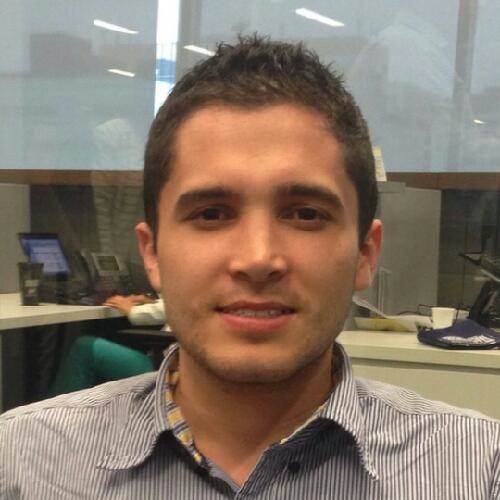
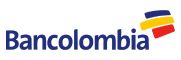