Building a RESTful API with CakePHP and HATEOAS
In the ever-evolving world of web development, building robust and efficient APIs is crucial for creating dynamic and interactive applications. Representational State Transfer (REST) has emerged as a standard architectural style for designing networked applications. In this blog, we will delve into the process of building a RESTful API using CakePHP, a popular PHP framework, and explore how to incorporate HATEOAS (Hypertext as the Engine of Application State) to create a more navigable and user-friendly API.
Table of Contents
1. Understanding RESTful APIs and HATEOAS
1.1. What is a RESTful API?
RESTful APIs provide a way for different software applications to communicate over the internet. They adhere to the principles of REST, which emphasizes a stateless client-server architecture and a set of well-defined operations (HTTP methods) for interacting with resources. RESTful APIs are characterized by their clear separation of concerns, allowing clients to access and manipulate server-side data using standardized HTTP methods.
1.2. Introducing HATEOAS
HATEOAS, or Hypertext as the Engine of Application State, is a constraint of the REST architecture that enhances the usability and navigability of APIs. It suggests that a client interacting with a RESTful API should be able to navigate the API’s endpoints through the hypermedia links embedded in the responses. In simpler terms, the API should guide the client by providing relevant links to related resources, enabling a more intuitive user experience and reducing the need for clients to have prior knowledge of the API structure.
2. Setting Up CakePHP for API Development
2.1. Installing CakePHP
Before we begin, ensure you have Composer installed, as CakePHP relies on it for package management. To install CakePHP, run the following command:
bash composer create-project --prefer-dist cakephp/app my_api
2.2. Creating the API Project
Once CakePHP is installed, navigate to the project directory and run the built-in development server:
bash cd my_api bin/cake server
Your CakePHP application will now be accessible at http://localhost:8765.
3. Designing the RESTful API
3.1. Defining Resources and Endpoints
In CakePHP, you can define resources and endpoints using routes. Open the config/routes.php file and add routes for your API endpoints:
php use Cake\Routing\Route\DashedRoute; Router::scope('/api', function ($routes) { $routes->setExtensions(['json']); // Allow JSON responses $routes->resources('Articles'); });
This code snippet defines a route for an ArticlesController, which will handle CRUD operations for articles.
3.2. Implementing CRUD Operations
Create the ArticlesController by running the following command:
bash bin/cake bake controller Articles
This will generate the controller and associated views. Implement the CRUD operations in the controller using CakePHP’s conventions.
php public function index() { $articles = $this->Articles->find('all'); $this->set([ 'articles' => $articles, '_serialize' => ['articles'] ]); } public function view($id) { $article = $this->Articles->get($id); $this->set([ 'article' => $article, '_serialize' => ['article'] ]); } // Implement other CRUD actions (add, edit, delete)
3.3. Serializing Data
Notice the _serialize key in the above code. This tells CakePHP which view variables to serialize into the response. CakePHP will automatically handle converting these variables into JSON.
4. Incorporating HATEOAS for Enhanced Navigation
4.1. Linking Resources
To incorporate HATEOAS, we need to include hypermedia links in our API responses. Modify the view action in the ArticlesController to include links to related resources:
php public function view($id) { $article = $this->Articles->get($id); $article['_links'] = [ 'self' => [ 'href' => Router::url(['action' => 'view', $id], true) ], 'collection' => [ 'href' => Router::url(['action' => 'index'], true) ] ]; $this->set([ 'article' => $article, '_serialize' => ['article'] ]); }
4.2. Dynamically Generating Links
CakePHP’s Router class helps us generate URLs, making it easy to dynamically create links. The above example demonstrates how to generate links to the current resource and the collection of resources.
4.3. Improving User Experience
By including hypermedia links, your API becomes more user-friendly. Clients can navigate through resources without needing prior knowledge of your API structure. This approach also makes it easier to evolve your API over time, as clients rely on the links provided rather than hardcoding endpoints.
5. Securing the API
5.1. Authentication and Authorization
Securing your API is crucial to protect sensitive data and ensure that only authorized users can access certain endpoints. CakePHP provides authentication and authorization components that can be easily integrated into your API.
5.2. Implementing API Tokens
One common method of authentication is using API tokens. Generate a token for each user upon registration and have clients include this token in the request headers for authentication.
6. Testing and Documentation
6.1. Writing Unit Tests
Maintaining a well-tested API is essential for a stable application. CakePHP offers a testing suite that allows you to write unit tests for your API endpoints.
6.2. Generating API Documentation
Clear documentation is key for developers who will be integrating with your API. Tools like Swagger or API Blueprint can help you generate interactive API documentation from annotations in your code.
Conclusion
In this blog post, we’ve explored the process of building a RESTful API using CakePHP and incorporating the principles of HATEOAS to enhance the user experience. We’ve covered setting up CakePHP, designing endpoints, implementing CRUD operations, and ensuring API security. By embracing HATEOAS, we’ve made our API more intuitive and adaptable, allowing clients to navigate through resources seamlessly. Remember that creating a successful API requires continuous refinement, testing, and documentation. Armed with the knowledge from this guide, you’re well on your way to developing APIs that empower your applications with flexibility and user-centric design.
Table of Contents
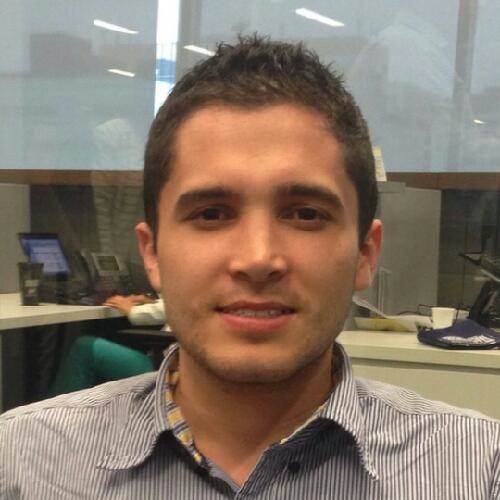
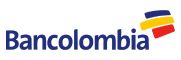