Implementing Role-Based Access Control (RBAC) in CakePHP
In the realm of web applications, security is of paramount importance. Ensuring that users have access only to the resources they need and are authorized to use is a critical aspect of safeguarding sensitive data and maintaining system integrity. This is where Role-Based Access Control (RBAC) comes into play. RBAC provides a structured approach to managing access rights by assigning roles to users and permissions to roles. In this tutorial, we will explore how to implement RBAC in a CakePHP application, bolstering its security foundation.
Table of Contents
1. Understanding Role-Based Access Control (RBAC)
1.1. What is RBAC?
Role-Based Access Control (RBAC) is a method used to manage access to resources in a system based on the roles assigned to users. Each user is associated with one or more roles, and each role is granted certain permissions. This hierarchical approach simplifies access management and enhances security by ensuring that users only have access to the features and data relevant to their roles.
1.2. Advantages of RBAC
RBAC offers several benefits for application security and management:
- Granular Access Control: RBAC allows fine-grained control over what users can and cannot do within the application.
- Simplified Management: Administrators can easily manage user permissions by assigning and revoking roles, without needing to modify individual user accounts.
- Scalability: As your application grows, RBAC makes it easier to maintain a structured and organized access control system.
- Audit Trails: RBAC provides an audit trail for user actions, making it easier to track who did what in the system.
2. Setting Up a CakePHP Project
Before we delve into implementing RBAC, let’s set up a CakePHP project if you haven’t already.
2.1. Installation and Configuration
Assuming you have Composer installed, create a new CakePHP project using the following command:
bash composer create-project --prefer-dist cakephp/app my_rbac_app
2.2. Navigate to the project directory:
bash cd my_rbac_app
Next, configure your database connection in config/app.php. Update the ‘Datasources’ section with your database details.
3. Database Design for RBAC
To implement RBAC, we need to design our database to store information about users, roles, and permissions.
Users, Roles, and Permissions Tables
We’ll create the following tables:
- users: Store user information (id, username, password, etc.).
- roles: Define different roles in the system (id, name, description).
- permissions: Define individual permissions (id, name, description).
- roles_users: Map users to roles (user_id, role_id).
- permissions_roles: Map roles to permissions (role_id, permission_id).
You can use CakePHP’s schema generation commands to create these tables. Run the following commands in your terminal:
bash bin/cake bake migration CreateUsers bin/cake bake migration CreateRoles bin/cake bake migration CreatePermissions bin/cake bake migration CreateRolesUsers bin/cake bake migration CreatePermissionsRoles
This will generate migration files in the config/Migrations directory. Edit these migration files to define the structure of each table and their relationships.
4. Implementing RBAC in CakePHP
With our database structure in place, let’s move on to implementing RBAC within our CakePHP application.
4.1. Creating Roles and Permissions
In your CakePHP application, you can create roles and permissions using CakePHP’s models and controllers. Here’s an example of how you might create a role:
php // src/Controller/RolesController.php public function add() { $role = $this->Roles->newEmptyEntity(); if ($this->request->is('post')) { $role = $this->Roles->patchEntity($role, $this->request->getData()); if ($this->Roles->save($role)) { $this->Flash->success(__('The role has been saved.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to add the role.')); } $this->set('role', $role); }
Similarly, you can create a permissions controller and views to manage permissions.
4.2. Assigning Roles to Users
To assign roles to users, you can create a form where administrators can select roles for each user. Here’s an example of how you might implement this:
php // src/Controller/UsersController.php public function assignRoles($userId) { $user = $this->Users->get($userId); if ($this->request->is(['patch', 'post', 'put'])) { $user = $this->Users->patchEntity($user, $this->request->getData(), [ 'associated' => ['Roles'] ]); if ($this->Users->save($user)) { $this->Flash->success(__('Roles have been assigned to the user.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to assign roles to the user.')); } $roles = $this->Users->Roles->find('list'); $this->set(compact('user', 'roles')); }
4.3. Checking Permissions
To check if a user has a specific permission, you can create a utility function in your User entity:
php // src/Model/Entity/User.php public function hasPermission($permissionName) { foreach ($this->roles as $role) { foreach ($role->permissions as $permission) { if ($permission->name === $permissionName) { return true; } } } return false; }
Now you can use this function to check permissions throughout your application.
5. Putting It All Together
5.1. Building a Secure User Dashboard
Suppose you have a user dashboard with various actions that require different permissions. You can use the hasPermission function we created earlier to restrict access:
php // src/Controller/DashboardController.php public function viewReports() { $user = $this->Auth->user(); if ($user->hasPermission('view_reports')) { // Allow access to view reports } else { // Redirect or display an error message } }
5.2. Handling Unauthorized Access
To handle unauthorized access, you can utilize CakePHP’s AuthComponent to automatically redirect users to a designated page:
php // src/Controller/AppController.php public function initialize() { parent::initialize(); $this->loadComponent('Auth', [ 'authorize' => 'Controller', 'loginRedirect' => [ 'controller' => 'Dashboard', 'action' => 'index' ], 'unauthorizedRedirect' => [ 'controller' => 'Users', 'action' => 'accessDenied' ] ]); }
Conclusion
Implementing Role-Based Access Control in CakePHP can significantly enhance the security and manageability of your web application. By structuring user roles, permissions, and access checks, you can ensure that users only have access to the resources they need. CakePHP’s powerful tools for managing database relationships and the built-in AuthComponent make implementing RBAC a streamlined process.
In this tutorial, we’ve covered the basics of setting up RBAC, designing the necessary database tables, creating roles and permissions, assigning roles to users, and checking permissions. Remember that security is an ongoing effort, so regularly review and update your RBAC setup to adapt to changing requirements and potential vulnerabilities. With the foundation of RBAC in place, your CakePHP application is better equipped to handle access control in a structured and secure manner.
Table of Contents
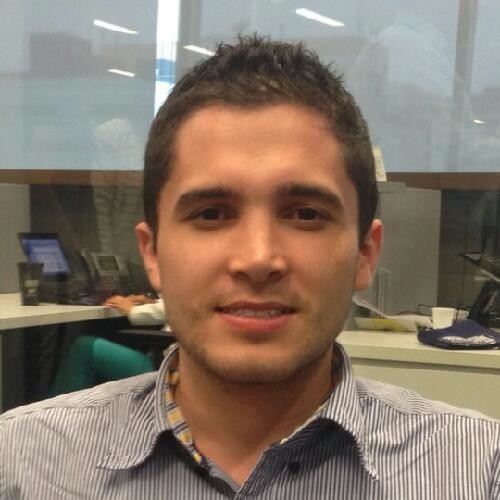
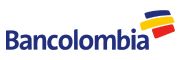