Securing CakePHP Applications with Two-Factor Authentication (2FA)
In today’s digital landscape, ensuring the security of user accounts is paramount. With the increasing number of cyber threats and data breaches, relying solely on traditional username and password authentication is no longer sufficient. This is where Two-Factor Authentication (2FA) comes into play as an additional layer of security that can greatly enhance the protection of user accounts. In this article, we’ll explore how to secure your CakePHP applications using Two-Factor Authentication.
Table of Contents
1. Understanding Two-Factor Authentication (2FA)
Two-Factor Authentication (2FA) is a security mechanism that requires users to provide two different authentication factors to verify their identity. These factors typically include something the user knows (like a password) and something the user possesses (like a mobile device or security token). By combining these two factors, 2FA adds an extra layer of security, making it significantly harder for unauthorized individuals to gain access to user accounts, even if they manage to obtain the user’s password.
2. The Benefits of Two-Factor Authentication
Implementing Two-Factor Authentication in your CakePHP application offers several key benefits:
- Enhanced Security: With an additional layer of authentication, even if a user’s password is compromised, attackers cannot access the account without the second authentication factor.
- Reduced Risk of Unauthorized Access: 2FA drastically reduces the risk of unauthorized access, protecting sensitive user data and confidential information.
- Mitigation of Phishing Attacks: Even if users fall victim to phishing attacks and reveal their passwords, attackers would still need the second factor to breach the account.
- Compliance Requirements: Some industries and regulatory standards require the use of multi-factor authentication to ensure the security of user data.
3. Implementing Two-Factor Authentication in CakePHP
Now, let’s delve into the process of implementing Two-Factor Authentication in your CakePHP application.
3.1. Choose a 2FA Method
There are several methods for the second authentication factor:
- Time-Based One-Time Password (TOTP): This involves generating a time-sensitive code using a shared secret and a mobile app like Google Authenticator.
- SMS or Email Codes: Sending a code to the user’s registered phone number or email address.
- Push Notifications: Sending a push notification to a mobile app for approval.
- Hardware Tokens: Physical devices that generate authentication codes.
- Choose the method that best suits your users and application.
3.2. Integrate the 2FA Library
CakePHP doesn’t have native 2FA support, but you can leverage third-party libraries to simplify the implementation. One such library is the “RobThree/TwoFactorAuth” library available via Composer.
Use the following Composer command to install the library:
bash composer require robthree/twofactorauth
3.3. User Setup and Configuration
For each user interested in enabling 2FA, generate a secret key and present it to the user. This key will be used to set up the authentication app. Store the encrypted secret key in the user’s database record.
Here’s an example of generating and storing a secret key using CakePHP’s syntax:
php use RobThree\Auth\TwoFactorAuth; $secretKey = (new TwoFactorAuth())->createSecret(); // Save $secretKey in the user's database record
3.4. QR Code Generation
To make it easier for users to set up 2FA, generate a QR code that they can scan with their authentication app. This QR code should contain the user’s email and the secret key.
Use the following code to generate a QR code in CakePHP:
php use RobThree\Auth\TwoFactorAuth; $authenticator = new TwoFactorAuth(); $qrCodeData = $authenticator->getQRCodeImageAsDataUri('Your App Name', $user->email, $secretKey); // Display the $qrCodeData as an image in your view
3.5. Verification and Authentication
When the user tries to log in, ask them for the authentication code from their chosen 2FA method. Verify this code using the secret key stored in the user’s record. If the code matches, allow the user to proceed; otherwise, deny access.
Here’s how you can verify the authentication code in CakePHP:
php use RobThree\Auth\TwoFactorAuth; $authenticator = new TwoFactorAuth(); $isValid = $authenticator->verifyCode($secretKey, $inputCode); if ($isValid) { // Grant access to the user } else { // Deny access }
3.6. Providing Backup Methods
It’s essential to provide backup methods for cases where the user can’t access their primary 2FA method (e.g., a lost phone). This could include email-based codes or security questions.
4. Best Practices for Two-Factor Authentication
While implementing 2FA, consider these best practices to ensure optimal security:
- Educate Users: Provide clear instructions on setting up and using 2FA to ensure user adoption.
- Use Trusted Libraries: Leverage well-established libraries like “RobThree/TwoFactorAuth” to avoid security vulnerabilities.
- Encourage Regular Updates: Prompt users to review and update their 2FA settings periodically.
- Offer Multiple Options: Allow users to choose from various 2FA methods for their convenience.
- Secure Key Storage: Safely store the secret key, preferably using encryption, to prevent unauthorized access.
- Graceful Recovery: Implement a process for users to regain access if they lose their 2FA device.
- Monitor and Audit: Keep track of 2FA events and monitor for any unusual activity.
Conclusion
Two-Factor Authentication (2FA) is a powerful tool that significantly enhances the security of your CakePHP applications by adding an extra layer of authentication. By implementing 2FA, you can mitigate the risks associated with password-based attacks and data breaches, safeguarding your users’ sensitive information. Through careful integration of 2FA methods and libraries, along with educating your users about the benefits, you can create a more secure online environment for your application. Remember, in the ever-evolving landscape of cybersecurity, staying one step ahead in protecting user accounts is not just a choice but a necessity.
Table of Contents
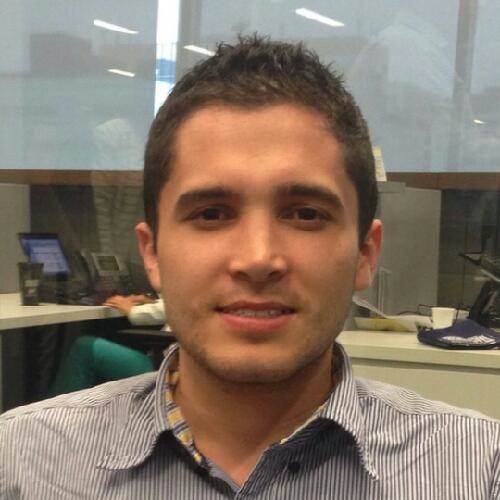
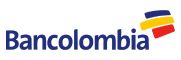