Securing CakePHP Applications with Cross-Site Request Forgery (CSRF) Protection
In today’s interconnected digital landscape, web application security is paramount. One of the critical security concerns developers face is Cross-Site Request Forgery (CSRF) attacks, which can compromise the integrity of web applications. CakePHP, a popular PHP framework, offers robust features to counter these threats and secure your applications. In this blog, we’ll explore the ins and outs of CSRF attacks, understand how they affect CakePHP applications, and delve into implementing CSRF protection with practical code examples.
Table of Contents
1. Understanding Cross-Site Request Forgery (CSRF) Attacks:
Cross-Site Request Forgery (CSRF) is a type of attack where an attacker tricks a user into unknowingly executing malicious actions on a web application. This occurs when the attacker convinces the victim’s browser to make requests to the application without the victim’s consent. CSRF attacks exploit the fact that browsers automatically include the user’s session cookies with each request to the same domain, regardless of the origin of the request.
2. CSRF Attack Scenario:
Imagine a user is logged into their online banking application. While still authenticated, they visit a malicious website that contains hidden requests to transfer funds from their account to the attacker’s account. Since the user’s browser includes their banking session cookie, the malicious requests are authenticated, and funds are transferred without the user’s knowledge.
3. CSRF Protection in CakePHP:
CakePHP provides built-in mechanisms to defend against CSRF attacks and protect your application’s users. The framework implements this protection through a combination of unique tokens, automatic verification, and user authentication.
4. Implementation Steps:
To secure your CakePHP application against CSRF attacks, follow these steps:
Step 1: Configure CSRF Component:
Open the AppController.php file, which is the base controller for your application, and add the CSRF component to its initialize() method:
php // app/Controller/AppController.php public function initialize() { parent::initialize(); $this->loadComponent('Csrf'); }
Step 2: Adding CSRF Token to Forms:
For every form in your CakePHP application, you’ll need to include a CSRF token. The token is generated automatically and should be added as a hidden field within your forms:
php // In a CakePHP view file echo $this->Form->create(); echo $this->Form->hidden('_csrfToken', ['value' => $this->request->getAttribute('csrfToken')]); // ... other form fields and submit button echo $this->Form->end();
Step 3: Verifying CSRF Token:
CakePHP handles the verification of the CSRF token automatically. If a submitted token doesn’t match the expected token, CakePHP will throw an exception, preventing the action from being executed. There’s no need for manual token verification.
5. Benefits of Using CakePHP’s CSRF Protection:
- Automatic Token Generation: CakePHP takes care of generating unique CSRF tokens for each request, reducing the risk of token reuse.
- Simplified Implementation: By simply including the CSRF component and using the FormHelper to generate the token field, developers can ensure protection against CSRF attacks with minimal effort.
- Enhanced Security: The built-in CSRF protection in CakePHP helps prevent unauthorized actions, maintaining the integrity of your application’s data and functionality.
6. Best Practices for CSRF Protection:
- Use HTTPS: Transmitting sensitive data, including CSRF tokens, over an encrypted connection (HTTPS) ensures that attackers cannot intercept or manipulate the token.
- Limit Token Lifespan: Configure your application to invalidate tokens after a certain period or after a specific number of requests, reducing the window of opportunity for attackers.
- Unique Tokens: Ensure that each request has a unique token, making it difficult for attackers to predict or reuse tokens.
- Logout on Inactivity: Implement an automatic logout mechanism for users who have been inactive for a certain period. This reduces the chances of an attacker using a session to execute unauthorized actions.
Conclusion
Securing CakePHP applications against Cross-Site Request Forgery (CSRF) attacks is a crucial aspect of maintaining the integrity and trustworthiness of your web applications. By following the implementation steps outlined in this blog, you can leverage CakePHP’s built-in CSRF protection mechanisms to safeguard your users’ data and interactions. Stay proactive about security, keep your application up-to-date, and regularly review your code to ensure a robust defense against CSRF attacks and other potential threats. Your users deserve a safe and seamless online experience, and a well-protected CakePHP application can deliver just that.
Table of Contents
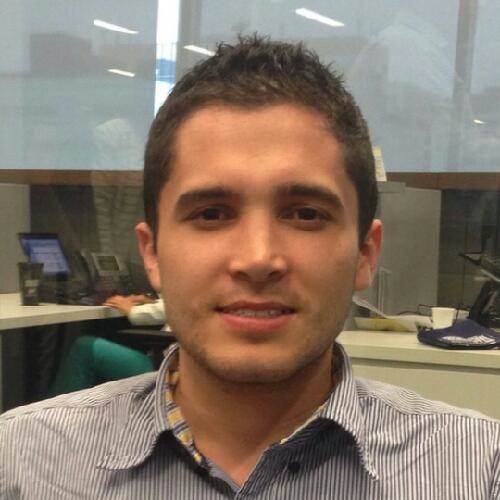
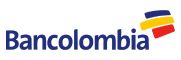