CakePHP Security Best Practices: Protecting Your Application
In today’s digital world, where cyber threats are a constant concern, securing web applications is of paramount importance. CakePHP, a popular PHP framework, offers a robust set of security features and practices that can help protect your application from various vulnerabilities. By implementing these best practices, you can ensure that your CakePHP application remains resilient against potential attacks, safeguarding your data and users’ sensitive information.
Table of Contents
1. Keep Your CakePHP and its Components Updated
One of the foundational steps in maintaining a secure application is to use the latest version of CakePHP and its associated components. The CakePHP development team actively works to address security issues and releases regular updates. By keeping your framework up to date, you ensure that your application benefits from the latest security patches, bug fixes, and performance improvements.
Code Example:
bash # Update CakePHP using composer composer update
2. Enable and Configure CSRF Protection
Cross-Site Request Forgery (CSRF) attacks are a common threat that can trick authenticated users into performing unwanted actions. CakePHP provides built-in CSRF protection that guards against such attacks by generating and validating tokens for each user session.
To enable CSRF protection, navigate to the AppController.php file and add the following code:
Code Example:
php // File: src/Controller/AppController.php use Cake\Controller\Controller; use Cake\Event\EventInterface; class AppController extends Controller { public function initialize(): void { parent::initialize(); $this->loadComponent('Security'); } public function beforeFilter(EventInterface $event) { $this->getEventManager()->off($this->Security); $this->Security->setConfig('unlockedActions', ['your_unprotected_action']); } }
Replace ‘your_unprotected_action’ with the name of any action that doesn’t require CSRF protection.
3. Implement Input Validation and Data Sanitization
User input is one of the primary sources of security vulnerabilities. CakePHP offers validation and sanitization features that help prevent malicious data from reaching your application’s core logic.
Code Example:
php // File: src/Model/Table/UsersTable.php use Cake\ORM\Table; use Cake\Validation\Validator; class UsersTable extends Table { public function validationDefault(Validator $validator): Validator { $validator ->notEmptyString('username', 'A username is required.') ->notEmptyString('password', 'A password is required.') ->email('email', 'Invalid email address.') ->add('email', 'unique', ['rule' => 'validateUnique', 'provider' => 'table', 'message' => 'This email is already taken.']); return $validator; } }
4. Protect Sensitive Data with Encryption
Data encryption is essential to protect sensitive information such as passwords, credit card numbers, or any other personally identifiable information (PII). Utilize CakePHP’s built-in encryption features to store such data securely in your database.
Code Example:
php // File: src/Model/Entity/User.php use Cake\Auth\AbstractPasswordHasher; class User extends Entity { protected function _setPassword(string $password): ?string { if (strlen($password) > 0) { return (new DefaultPasswordHasher())->hash($password); } return null; } }
The above code demonstrates how to encrypt the user’s password before saving it to the database.
5. Sanitize User Output to Prevent XSS Attacks
Cross-Site Scripting (XSS) attacks are a significant security risk where attackers inject malicious scripts into your application’s output. CakePHP offers automatic output escaping to help prevent XSS vulnerabilities.
Code Example:
php // File: src/Template/Users/view.ctp <h1><?php echo h($user->name); ?></h1> <p><?php echo h($user->bio); ?></p>
Using the h() function in your templates automatically escapes any user-generated content, making it safe to display in the browser.
6. Implement Authentication and Authorization Properly
Proper authentication and authorization are critical aspects of web application security. CakePHP provides a flexible and powerful authentication system that can be easily integrated into your application.
Code Example:
php // File: src/Controller/AppController.php use Cake\Controller\Controller; use Cake\Event\EventInterface; use Cake\Auth\DefaultPasswordHasher; class AppController extends Controller { public function initialize(): void { parent::initialize(); $this->loadComponent('Auth', [ 'loginAction' => [ 'controller' => 'Users', 'action' => 'login', ], 'authenticate' => [ 'Form' => [ 'fields' => ['username' => 'email', 'password' => 'password'], 'passwordHasher' => DefaultPasswordHasher::class, ] ], 'loginRedirect' => [ 'controller' => 'Dashboard', 'action' => 'index', ], 'logoutRedirect' => [ 'controller' => 'Users', 'action' => 'login', ], 'unauthorizedRedirect' => [ 'controller' => 'Pages', 'action' => 'display', 'home', ], ]); } }
In this example, we configure the Auth component to use the Form authentication adapter with the DefaultPasswordHasher.
7. Implement Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) restricts certain actions or pages to authorized users based on their roles. This mechanism is crucial for managing user permissions and preventing unauthorized access to sensitive parts of your application.
Code Example:
php // File: src/Controller/Admin/UsersController.php class UsersController extends AppController { public function initialize(): void { parent::initialize(); $this->Auth->deny(['index', 'edit']); $this->Auth->allow('view'); } }
In this example, we deny access to the ‘index’ and ‘edit’ actions for all users and only allow access to the ‘view’ action.
8. Enable HTTP Security Headers
HTTP Security Headers add an extra layer of protection by mitigating potential security risks. CakePHP allows you to set HTTP headers to enhance security by reducing the risk of attacks such as Cross-Site Script Inclusion (XSSI) and Clickjacking.
Code Example:
php // File: src/Application.php use Cake\Http\BaseApplication; class Application extends BaseApplication { public function middleware($middlewareQueue) { // ... $middlewareQueue->add(function ($req, $res, $next) { $res = $res->withHeader('X-Content-Type-Options', 'nosniff') ->withHeader('X-Frame-Options', 'SAMEORIGIN') ->withHeader('X-XSS-Protection', '1; mode=block'); return $next($req, $res); }); // ... } }
In this example, we add middleware to the application to include security headers in the response.
Conclusion
Securing your CakePHP application is an ongoing process that requires vigilance and adherence to best practices. By keeping the framework and its components up-to-date, enabling CSRF protection, validating and sanitizing input, encrypting sensitive data, and implementing proper authentication and authorization mechanisms, you can significantly reduce the risk of security breaches.
Remember to conduct regular security audits and stay informed about the latest security trends and threats. With a proactive approach to security, you can ensure your CakePHP application remains resilient against potential attacks, protecting your data and providing a safe experience for your users.
Table of Contents
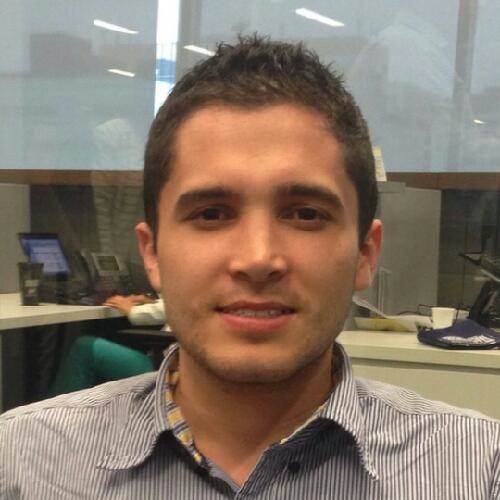
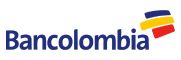