Working with CakePHP’s Security Component: Protecting Your App
In today’s digital landscape, web application security is paramount. Ensuring that your CakePHP application is safeguarded against common vulnerabilities like cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection is crucial. Fortunately, CakePHP provides a robust solution for this: the Security Component. In this comprehensive guide, we’ll explore how to harness the power of CakePHP’s Security Component to protect your application effectively.
Table of Contents
1. Introduction to CakePHP’s Security Component
CakePHP’s Security Component is a versatile tool that helps developers address common security concerns in web applications. It offers a wide range of features designed to mitigate risks associated with user input, form submissions, and data handling. By utilizing this component effectively, you can significantly reduce the chances of your application falling victim to various types of attacks.
2. Setting Up the Security Component
2.1. Enabling the Security Component
Before you can start leveraging the Security Component, you need to enable it in your CakePHP application. To do this, open your AppController.php file and add the following line to the initialize method:
php public function initialize(): void { parent::initialize(); $this->loadComponent('Security'); }
This line loads the Security Component and makes it available throughout your application.
2.2. Configuration Options
The Security Component comes with a range of configuration options that allow you to tailor its behavior to your application’s specific needs. Some essential configuration options include:
- csrfCheck: Enables or disables CSRF protection. Set it to true to enable CSRF protection globally.
- unlockedActions: An array of controller actions that should be exempt from security checks.
- blackHoleCallback: A callback function that handles security violations, such as CSRF token mismatch.
Here’s an example of how to configure the Security Component to enable CSRF protection globally and specify a custom callback function:
php public function initialize(): void { parent::initialize(); $this->loadComponent('Security', [ 'csrfCheck' => true, 'blackHoleCallback' => 'handleSecurityError', ]); }
These are just a few of the many configuration options available, and you should adjust them according to your application’s requirements.
3. Cross-Site Scripting (XSS) Protection
Cross-Site Scripting (XSS) is a widespread security vulnerability where an attacker injects malicious scripts into a web application, which are then executed in the context of a user’s browser. The Security Component helps prevent XSS attacks by automatically escaping output data.
To utilize this feature, ensure that you use CakePHP’s built-in methods for outputting data in your views. For example:
php <?= h($data) ?>
The h() function automatically escapes the data, making it safe to display in HTML.
4. Cross-Site Request Forgery (CSRF) Prevention
CSRF attacks occur when an attacker tricks a user into performing actions they didn’t intend to on a web application. To mitigate CSRF vulnerabilities, CakePHP’s Security Component provides built-in protection in the form of tokens.
4.1. Generating CSRF Tokens
To generate CSRF tokens, use the FormHelper in your CakePHP forms. Here’s an example:
php <?= $this->Form->create() ?> <?= $this->Form->input('username') ?> <?= $this->Form->input('password') ?> <?= $this->Form->button(__('Submit')) ?> <?= $this->Form->end() ?>
This code generates a hidden input field containing the CSRF token. When the form is submitted, CakePHP validates the token automatically.
4.2. Handling CSRF Violations
If a CSRF token validation fails, the Security Component triggers a black hole callback. You can customize this callback to handle CSRF violations gracefully, such as logging the incident or redirecting the user to a safe page.
Here’s an example of a black hole callback function:
php public function handleSecurityError($type) { if ($type === 'csrf') { $this->Flash->error(__('CSRF token validation failed.')); return $this->redirect('/'); } }
5. SQL Injection Prevention
SQL injection is a severe security threat that occurs when an attacker injects malicious SQL queries into an application’s database queries. CakePHP’s Security Component helps mitigate this risk by using prepared statements and parameterized queries by default.
When using CakePHP’s ORM (Object-Relational Mapping), queries are automatically parameterized, making it challenging for attackers to inject malicious SQL code.
For example, instead of writing raw SQL queries like:
php $query = "SELECT * FROM users WHERE username = '$username'";
Use CakePHP’s query builder:
php $query = $this->Users->find() ->where(['username' => $username]) ->toArray();
This way, your queries are automatically sanitized and protected against SQL injection.
6. Input Validation and Sanitization
Validating and sanitizing user input is a fundamental aspect of web application security. CakePHP provides robust tools for input validation and sanitization.
6.1. Validation Rules
You can define validation rules for your models using CakePHP’s built-in validation features. Here’s an example of defining validation rules in a CakePHP model:
php // In src/Model/Table/UsersTable.php public function validationDefault(Validator $validator): Validator { $validator ->requirePresence('username') ->notEmptyString('username', 'Please enter a username.') ->add('username', 'unique', [ 'rule' => 'validateUnique', 'provider' => 'table', 'message' => 'This username is already taken.', ]) ->requirePresence('email') ->notEmptyString('email', 'Please enter an email address.') ->add('email', 'valid-email', [ 'rule' => 'email', 'message' => 'Please enter a valid email address.', ]); return $validator; }
These validation rules ensure that data entered into the username and email fields meets specific criteria.
6.2. Sanitization
Sanitization is the process of cleaning user input to remove potentially dangerous characters or code. CakePHP offers built-in sanitization methods like trim(), stripTags(), and htmlentities().
You can use these methods to sanitize user input before storing it in the database or displaying it in views. For example:
php $cleanedInput = trim($this->request->getData('user_input'));
By using these validation and sanitization techniques, you can significantly reduce the risk of security vulnerabilities caused by malicious user input.
7. Best Practices for Secure Coding
While CakePHP’s Security Component provides essential tools to enhance the security of your application, secure coding practices go beyond using security features. Here are some additional best practices:
7.1. Regularly Update Dependencies
Keep your CakePHP framework and other dependencies up to date. Security patches and updates are released regularly, so ensure your application benefits from the latest security improvements.
7.2. Implement Proper Authentication and Authorization
Use CakePHP’s built-in authentication and authorization features to control access to your application’s resources. Define roles and permissions to ensure that only authorized users can perform specific actions.
7.3. Secure Configuration
Keep sensitive information like database credentials and API keys out of your version control system. Use environment variables or configuration files outside of your web root to store such information securely.
7.4. Security Audits and Penetration Testing
Regularly perform security audits and penetration testing on your application to identify vulnerabilities and weaknesses. Address any issues promptly.
7.5. Educate Your Team
Ensure that your development team is aware of security best practices. Conduct training sessions and stay updated on the latest security threats and mitigation techniques.
Conclusion
In the ever-evolving landscape of web application security, CakePHP’s Security Component is a valuable ally in safeguarding your application against threats. By following the best practices outlined in this guide, you can strengthen your application’s defenses and minimize the risk of security breaches. Remember that security is an ongoing process, and staying vigilant is key to maintaining a secure CakePHP application.
Table of Contents
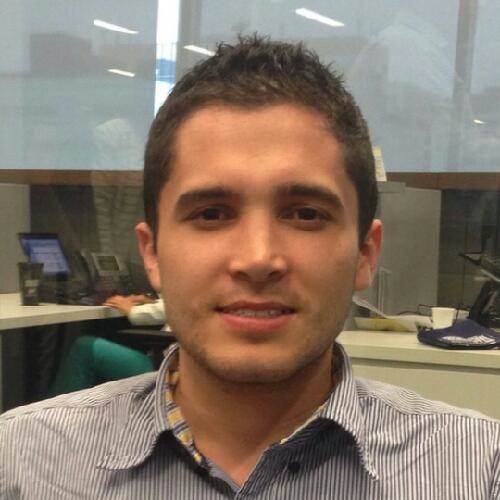
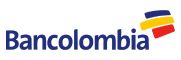