Using CakePHP’s Shell Dispatcher: Building Command-Line Tools
In the realm of web development, CakePHP has earned its reputation as a powerful and versatile PHP framework. While it’s primarily known for simplifying web application development, it also offers a lesser-known gem: the Shell Dispatcher. This hidden treasure enables developers to build robust command-line tools effortlessly. In this blog, we’ll embark on a journey to explore CakePHP’s Shell Dispatcher, learning how to harness its capabilities to create powerful and efficient command-line tools for your PHP projects.
Table of Contents
1. Why Command-Line Tools Matter
Before we dive into the intricacies of CakePHP’s Shell Dispatcher, let’s take a moment to understand why command-line tools are crucial in modern web development.
- Automation: Command-line tools allow you to automate repetitive tasks, such as database migrations, data seeding, or code generation. This automation saves time and reduces the risk of human error.
- Server Maintenance: Managing servers and deploying applications often involves command-line interactions. Being able to script these actions can simplify operations significantly.
- Customization: Every project has unique requirements. Command-line tools let you build custom scripts tailored to your application’s needs, enhancing flexibility and efficiency.
Now that we appreciate the importance of command-line tools let’s explore how CakePHP’s Shell Dispatcher can be your trusted companion in building them.
2. Getting Started with CakePHP’s Shell Dispatcher
2.1. Installing CakePHP
Before we can begin working with CakePHP’s Shell Dispatcher, we need to have CakePHP installed. If you haven’t already, you can install it using Composer:
bash composer create-project --prefer-dist cakephp/app myapp
This command sets up a fresh CakePHP application named ‘myapp.’
2.2. Creating Your First Shell
CakePHP’s Shell Dispatcher is built upon the concept of “shells.” Shells are PHP classes that contain the logic for your command-line tools. To create your first shell, run the following command from your project’s root directory:
bash bin/cake bake shell MyShell
This command creates a shell named MyShell and places it in the src/Shell directory of your CakePHP project. You can choose any name you like for your shell.
2.3. Understanding the Shell Class
Open the newly created MyShell.php file inside the src/Shell directory. You’ll notice that CakePHP has generated a basic shell class structure for you:
php // src/Shell/MyShell.php namespace App\Shell; use Cake\Console\Shell; class MyShell extends Shell { public function main() { // Your code here } }
The MyShell class extends Cake\Console\Shell, and it contains a main method. The main method is the entry point for your shell’s logic.
3. Building Your Command-Line Tool
3.1. Adding Command-Line Actions
To make your command-line tool useful, you’ll want to define one or more actions within your shell. Each action is a public method in your shell class. Let’s add a simple action that displays a greeting:
php // src/Shell/MyShell.php public function hello() { $this->out('Hello, CakePHP Shell!'); }
Now, when you run the shell, you can execute the hello action:
bash bin/cake my_shell hello
You should see the output: “Hello, CakePHP Shell!”
3.2. Passing Arguments and Options
Command-line tools become even more powerful when they can accept arguments and options. In CakePHP’s Shell Dispatcher, you can define these by adding method parameters and docblock comments:
php // src/Shell/MyShell.php /** * Display a personalized greeting. * * @param string $name The name to greet. * @param bool $uppercase Whether to uppercase the greeting. * @return void */ public function greet($name = 'User', $uppercase = false) { $greeting = "Hello, $name!"; if ($uppercase) { $greeting = strtoupper($greeting); } $this->out($greeting); }
Now, you can use the greet action with arguments and options:
bash # Basic usage bin/cake my_shell greet Alice # Using the uppercase option bin/cake my_shell greet Bob --uppercase
By defining parameters in your method and adding corresponding docblock comments, CakePHP’s Shell Dispatcher automatically handles parsing and validation.
3.3. Interactive Shells
CakePHP’s Shell Dispatcher also supports interactive shells, which allow users to provide input during execution. You can use the $this->in() method to prompt for user input:
php // src/Shell/MyShell.php public function ask() { $name = $this->in('What is your name?'); $this->out("Hello, $name!"); }
When you run the ask action, it will prompt you for your name:
bash bin/cake my_shell ask What is your name? (Type your answer and press enter)
Interactive shells are useful for creating tools that require user input, such as setup wizards or configuration scripts.
4. Adding Help and Documentation
Good command-line tools provide clear and concise documentation to help users understand their capabilities. CakePHP’s Shell Dispatcher makes it easy to add documentation to your shells.
4.1. Adding a Description
You can add a brief description to your shell by setting the $this->description property:
php // src/Shell/MyShell.php public function initialize() { $this->description = 'A simple CakePHP shell for greeting users.'; }
This description will be displayed when users run bin/cake my_shell –help.
4.2. Documenting Actions
To document individual actions, use docblock comments above each action method. Include a @param tag for each argument and option, and add a @return tag to indicate the return type, if applicable:
php /** * Display a personalized greeting. * * @param string $name The name to greet. * @param bool $uppercase Whether to uppercase the greeting. * @return void */ public function greet($name = 'User', $uppercase = false) { // ... }
5. Running Shells
Running shells in CakePHP is straightforward. Simply use the bin/cake command followed by the shell name and action:
bash bin/cake my_shell hello
You can also use bin/cake without any arguments to list available shells and their actions:
bash bin/cake
This will display a list of available shells and actions for your CakePHP application.
Conclusion
CakePHP’s Shell Dispatcher is a valuable tool that can greatly enhance your PHP development experience by enabling you to build robust command-line tools effortlessly. Whether you need to automate tasks, manage server operations, or create custom scripts, CakePHP’s Shell Dispatcher has got you covered.
In this blog, we’ve covered the basics of creating a shell, defining actions, handling arguments and options, building interactive shells, and adding documentation. Armed with this knowledge, you’re well on your way to harnessing the full potential of CakePHP’s Shell Dispatcher for your projects.
So, start exploring the world of command-line tools with CakePHP today, and watch your development workflow become more efficient and powerful than ever before.
Happy coding!
Table of Contents
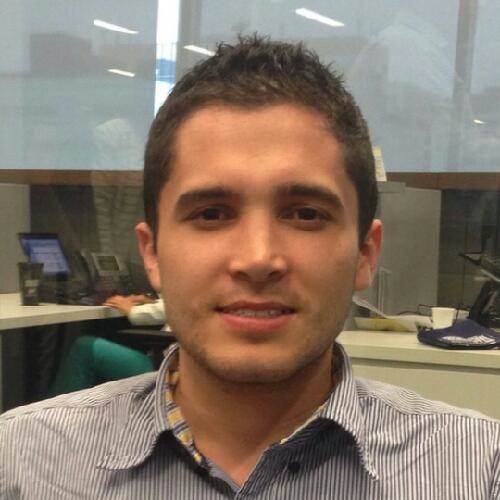
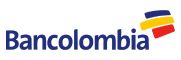