Building a Shopping Cart Application with CakePHP
In the world of e-commerce, having a well-designed shopping cart application is essential for providing a smooth and efficient online shopping experience to customers. CakePHP, a popular PHP framework, offers a powerful set of tools and features that can streamline the development of such applications. In this tutorial, we’ll walk through the process of building a shopping cart application using CakePHP, covering everything from setting up the project to implementing essential features.
Table of Contents
1. Prerequisites
Before we dive into the development process, make sure you have the following prerequisites in place:
- Basic understanding of PHP and CakePHP framework.
- A development environment with PHP and Composer installed.
- Familiarity with HTML, CSS, and JavaScript.
2. Setting Up the Project
Let’s start by setting up our CakePHP project. Open your terminal and navigate to the directory where you want to create the project.
bash composer create-project --prefer-dist cakephp/app shopping-cart
This command will create a new CakePHP project named “shopping-cart” using the latest stable version. Once the project is created, navigate into the project directory:
bash cd shopping-cart
3. Creating the Database Structure
Our shopping cart application will require a database to store product information, customer details, and cart contents. Let’s create the necessary database tables and relationships.
3.1. Products Table
We’ll start by creating a table to store product information, including the product name, price, and description.
php // src/Model/Table/ProductsTable.php use Cake\ORM\Table; class ProductsTable extends Table { public function initialize(array $config): void { parent::initialize($config); $this->setTable('products'); $this->addBehavior('Timestamp'); } // Define the schema and validation rules here }
3.2. Customers Table
Next, create a table to store customer information, such as name, email, and shipping address.
php // src/Model/Table/CustomersTable.php use Cake\ORM\Table; class CustomersTable extends Table { public function initialize(array $config): void { parent::initialize($config); $this->setTable('customers'); $this->addBehavior('Timestamp'); } // Define the schema and validation rules here }
3.3. Carts Table
Now, create a table to store cart contents, associating products with customers.
php // src/Model/Table/CartsTable.php use Cake\ORM\Table; class CartsTable extends Table { public function initialize(array $config): void { parent::initialize($config); $this->setTable('carts'); $this->belongsTo('Customers'); $this->belongsTo('Products'); } // Define the schema and validation rules here }
4. Building the Frontend
With the database structure in place, let’s move on to creating the frontend of our shopping cart application.
4.1. Product Listing Page
Start by creating a page that lists all the available products. You can use CakePHP’s baked templates to generate the initial CRUD views for the ProductsController.
bash bin/cake bake controller Products bin/cake bake template Products
Customize the generated template files to display the list of products, including their names, prices, and “Add to Cart” buttons.
4.2. Shopping Cart Page
Create a page to display the contents of the customer’s shopping cart. You can utilize the CartsController to retrieve cart contents and the associated products.
bash bin/cake bake controller Carts bin/cake bake template Carts
Customize the template to display the cart contents, including product details and quantities. Provide options to update quantities or remove items from the cart.
5. Implementing Cart Functionality
Now comes the crucial part: implementing the shopping cart functionality. We’ll cover adding products to the cart, updating quantities, and checking out.
5.1. Adding Products to Cart
In the product listing page, add an “Add to Cart” button for each product. When the button is clicked, send an AJAX request to the server to add the selected product to the customer’s cart.
javascript // public/js/cart.js $(document).ready(function() { $('.add-to-cart').on('click', function() { var productId = $(this).data('product-id'); $.ajax({ url: '/carts/add-to-cart/' + productId, method: 'POST', success: function(response) { // Update the cart UI or provide feedback } }); }); });
In the CartsController, implement the add-to-cart action to handle the AJAX request and add the product to the cart.
php // src/Controller/CartsController.php public function addToCart($productId) { // Implement logic to add product to cart }
5.2. Updating Cart Quantities
On the shopping cart page, provide input fields to update the quantities of items in the cart. Implement AJAX requests to update quantities without requiring a page reload.
javascript // public/js/cart.js $('.quantity-input').on('change', function() { var cartItemId = $(this).data('cart-item-id'); var newQuantity = $(this).val(); $.ajax({ url: '/carts/update-quantity/' + cartItemId + '/' + newQuantity, method: 'POST', success: function(response) { // Update the cart total or other relevant information } }); });
Implement the update-quantity action in the CartsController to handle quantity updates.
php // src/Controller/CartsController.php public function updateQuantity($cartItemId, $newQuantity) { // Implement logic to update cart item quantity }
5.3. Checkout Process
Finally, implement the checkout process, which involves creating an order, deducting the products’ quantities from the inventory, and clearing the cart.
php // src/Controller/CartsController.php public function checkout() { // Implement logic for the checkout process }
Conclusion
Congratulations! You’ve successfully built a shopping cart application using CakePHP. You’ve learned how to set up the project, create the necessary database structure, build the frontend, and implement crucial cart functionality. By following these steps, you’ve laid the foundation for an efficient and user-friendly online shopping experience. Feel free to enhance the application further by adding features like user authentication, order history, and payment integration. Happy coding!
Building a robust shopping cart application with CakePHP might seem like a complex task, but with the right approach and understanding of the framework’s capabilities, it becomes a rewarding endeavor. From setting up the project and creating the database structure to building the frontend and implementing core cart functionality, this tutorial has provided a comprehensive guide to building a functional shopping cart application. Whether you’re a seasoned CakePHP developer or a newcomer, creating an efficient online shopping experience is within your reach.
Table of Contents
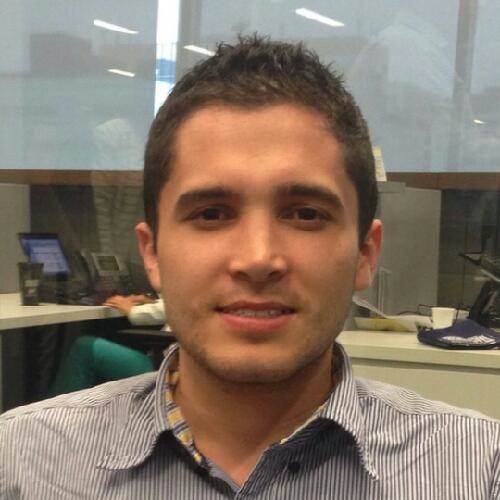
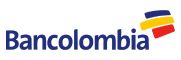