Implementing Single Sign-On (SSO) in CakePHP Applications
In today’s interconnected digital landscape, the ability to access multiple applications and services using a single set of credentials has become increasingly important. This is where Single Sign-On (SSO) comes into play. SSO simplifies the user experience, enhances security, and streamlines the authentication process. In this comprehensive guide, we will explore how to implement Single Sign-On in CakePHP applications, a popular PHP framework.
Table of Contents
1. Understanding Single Sign-On (SSO)
1.1. What is Single Sign-On (SSO)?
Single Sign-On (SSO) is an authentication process that allows users to access multiple applications or services with a single set of credentials. Instead of having to remember and enter separate usernames and passwords for each system, users log in once, and SSO securely authenticates them across all connected applications. This significantly improves the user experience and reduces the burden of managing multiple credentials.
1.2. Benefits of Implementing SSO
Implementing SSO in your CakePHP application can offer several advantages:
1.2.1. Simplified User Experience
Users appreciate the convenience of logging in once and gaining access to all associated services. It reduces the need to remember multiple passwords, making their interactions with your application smoother.
1.2.2. Enhanced Security
SSO centralizes authentication, making it easier to implement robust security measures. User credentials are stored securely, and it becomes easier to enforce policies like password complexity and multi-factor authentication.
1.2.3. Reduced Password Fatigue
Users tend to reuse passwords across different services, which can lead to security vulnerabilities. SSO minimizes this risk by eliminating the need for users to remember multiple passwords.
1.2.4. Streamlined User Management
Managing user access becomes more efficient as administrators can control access to multiple systems from a single point of control, reducing the risk of unauthorized access.
2. Preparing Your CakePHP Application
2.1. Setting Up Your Development Environment
Before implementing SSO in your CakePHP application, ensure you have a working development environment. This includes having PHP, CakePHP, and a database system installed and configured. If you haven’t already, download and install CakePHP by following the official installation guide.
2.2. Installing the Required Dependencies
To implement SSO, you’ll need to install some dependencies that can handle the authentication and authorization process. Two commonly used SSO protocols are OAuth 2.0 and SAML (Security Assertion Markup Language). Choose the one that suits your application’s requirements and proceed with its installation.
3. Choosing the SSO Protocol
3.1. OAuth 2.0
OAuth 2.0 is a widely adopted protocol for Single Sign-On that allows secure access to resources on behalf of a user. It is commonly used for scenarios where third-party applications or services need to access a user’s data with their permission. OAuth 2.0 involves the concepts of clients, authorization servers, and resource servers, making it versatile for various use cases.
3.2. SAML (Security Assertion Markup Language)
SAML is another robust protocol for Single Sign-On. It is often used in enterprise environments for web-based SSO. SAML exchanges XML-based assertions between the Identity Provider (IdP) and the Service Provider (SP) to authenticate users and communicate their attributes.
Choose the SSO protocol that aligns with your application’s needs, taking into consideration factors such as the type of applications you are integrating with and your security requirements.
4. Implementing SSO in CakePHP
Now that you’ve chosen your SSO protocol, it’s time to dive into the implementation process. We’ll walk through the steps to set up Single Sign-On in your CakePHP application.
4.1. Setting Up the Identity Provider (IdP)
In an SSO setup, the Identity Provider (IdP) is responsible for authenticating users and providing assertions to the Service Provider (SP). Depending on your chosen protocol, you may need to set up an IdP. This could be an external service or an internal system dedicated to handling authentication.
4.1.1. OAuth 2.0 Identity Provider Setup
For OAuth 2.0, you’ll need to register your application with the OAuth provider (e.g., Google, Facebook) to obtain client credentials (client ID and client secret). These credentials will be used to establish trust between your application and the IdP.
php // OAuth 2.0 Identity Provider Configuration (CakePHP) 'OAuth' => [ 'providers' => [ 'Google' => [ 'className' => 'League\OAuth2\Client\Provider\Google', 'clientId' => 'YOUR_CLIENT_ID', 'clientSecret' => 'YOUR_CLIENT_SECRET', 'redirectUri' => 'YOUR_REDIRECT_URI', ], ], ],
4.1.2. SAML Identity Provider Setup
For SAML, you may need to install and configure a SAML IdP solution like SimpleSAMLphp or use a commercial IdP service. Configuration will involve setting up metadata and certificates for secure communication.
4.2. Configuring the Service Provider (SP)
The Service Provider (SP) represents your CakePHP application, which relies on the IdP for user authentication. Configuration details for the SP will vary depending on your chosen protocol.
4.2.1. OAuth 2.0 Service Provider Configuration
For OAuth 2.0, configure the OAuth provider settings in your CakePHP application, specifying the authorization and token endpoints provided by the IdP.
php // OAuth 2.0 Service Provider Configuration (CakePHP) 'OAuth' => [ 'providers' => [ 'Google' => [ 'className' => 'League\OAuth2\Client\Provider\Google', 'clientId' => 'YOUR_CLIENT_ID', 'clientSecret' => 'YOUR_CLIENT_SECRET', 'redirectUri' => 'YOUR_REDIRECT_URI', ], ], ],
4.2.2. SAML Service Provider Configuration
For SAML, configure the SP settings in your CakePHP application, including the IdP metadata, certificates, and endpoints.
php // SAML Service Provider Configuration (CakePHP) 'SAML' => [ 'sp' => [ 'entityId' => 'YOUR_ENTITY_ID', 'assertionConsumerService' => [ 'url' => 'YOUR_ASSERTION_CONSUMER_SERVICE_URL', ], 'singleLogoutService' => [ 'url' => 'YOUR_SINGLE_LOGOUT_SERVICE_URL', ], 'NameIDFormat' => 'urn:oasis:names:tc:SAML:1.1:nameid-format:unspecified', ], ],
5. Handling User Authentication
With the IdP and SP configured, you can implement the user authentication flow in your CakePHP application. When a user accesses a protected resource, the application redirects them to the IdP for authentication. After successful authentication, the IdP sends an assertion back to your application, which verifies it and grants access to the user.
Here’s a simplified example of handling OAuth 2.0 authentication in CakePHP:
php // OAuth 2.0 Authentication Flow (CakePHP) public function login() { // Redirect the user to the OAuth provider for authentication $oauth = $this->loadComponent('OAuth.OAuth', [ 'providers' => ['Google'], // Use the configured OAuth provider ]); $oauth->authenticate('Google'); } public function callback() { // Handle the callback from the OAuth provider $oauth = $this->loadComponent('OAuth.OAuth', [ 'providers' => ['Google'], ]); $user = $oauth->getUser('Google'); // Use the user data to create or authenticate the user in your app }
5.1. Testing the SSO Integration
Testing is a crucial step in ensuring that your SSO implementation works smoothly. Test various scenarios, including successful login, failed authentication, and user provisioning. Use the developer tools provided by the IdP and SP to debug any issues that may arise.
6. Enhancing Security
While SSO enhances security by centralizing authentication, it’s essential to implement additional security measures to protect your CakePHP application further.
6.1. Implementing Multi-Factor Authentication (MFA)
Consider implementing Multi-Factor Authentication (MFA) to add an extra layer of security to user logins. MFA requires users to provide multiple forms of authentication, such as a password and a one-time code sent to their mobile device.
6.2. Monitoring and Auditing SSO Activity
Implement logging and monitoring to track SSO activity and identify any suspicious behavior or security threats. Regularly review logs and audit trails to ensure the security of your CakePHP application.
Conclusion
Implementing Single Sign-On (SSO) in your CakePHP application can greatly enhance the user experience while improving security and user management. By following the steps outlined in this guide, you can seamlessly integrate SSO into your application, choosing the protocol that best suits your needs. Remember to prioritize security by implementing MFA and monitoring SSO activity to ensure the safety of your users and their data.
With SSO in place, your CakePHP application will be better equipped to meet the demands of today’s interconnected digital landscape while maintaining a high level of security and convenience for your users.
Table of Contents
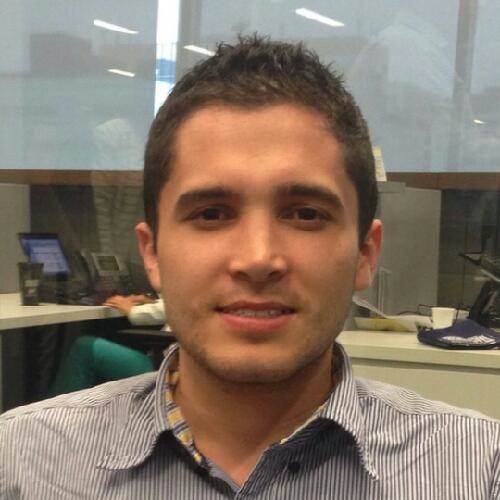
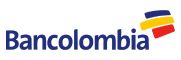