Exploring CakePHP’s Template System: Views and Layouts
As a web developer, you understand the importance of creating dynamic and visually appealing web pages. The Template System in CakePHP provides a robust and flexible way to achieve this by allowing you to separate the presentation layer from the business logic. This separation not only enhances code maintainability but also facilitates collaboration between designers and developers.
Table of Contents
In this blog, we’ll dive deep into CakePHP’s Template System, specifically focusing on Views and Layouts. We’ll explore how to leverage these components effectively to create stunning web applications while maintaining clean and organized code.
1. Introduction to CakePHP’s Template System
CakePHP is a powerful and popular PHP framework that follows the Model-View-Controller (MVC) architectural pattern. The “View” in MVC represents the presentation layer of your application, and CakePHP’s Template System is responsible for handling it. The Template System uses Views, Layouts, Elements, and Helpers to create a clear separation between the HTML and PHP code.
Views are responsible for rendering the final HTML output and presenting data to users. Layouts, on the other hand, are the wrapper around Views and define the common structure of web pages, like headers, footers, and sidebars. Elements, similar to partial views, allow you to reuse components across multiple pages. Helpers assist in generating HTML elements and logic within the View.
Now, let’s delve into each of these components to better understand how they work together to form a robust Template System in CakePHP.
2. Understanding Views
2.1. Creating Views
In CakePHP, Views are represented by files with a .ctp extension (which stands for CakePHP Template). These files are usually placed in the src/Template directory of your application, organized according to the corresponding controller and action. For example, if you have a PostsController with an index action, the corresponding view file would be index.ctp located in src/Template/Posts.
Here’s a simple example of a src/Template/Posts/index.ctp:
php <!-- src/Template/Posts/index.ctp --> <h1>Welcome to the Posts index page!</h1> <p>Here are the latest posts:</p> <ul> <?php foreach ($posts as $post): ?> <li><?php echo $post->title; ?></li> <?php endforeach; ?> </ul>
2.2. Rendering Views
To render a view, you use the render() method inside the controller’s action. The render() method loads the corresponding view file and passes data to it. For example, within the PostsController, the index action would render the index.ctp view we created earlier:
php // src/Controller/PostsController.php class PostsController extends AppController { public function index() { $posts = $this->Posts->find('all'); $this->set('posts', $posts); } }
In this example, we retrieve all posts from the database and pass them to the view using the set() method. The data is then accessible within the index.ctp view using the $posts variable.
2.3. Using Variables in Views
As seen in the previous example, you can pass data from the controller to the view using the set() method. In the view, you can access this data directly as variables. Besides controller data, you can also use CakePHP’s built-in variables and functions, such as $this->Html or $this->Form, which provide helper methods to generate HTML elements and forms, respectively.
2.4. Layouts Within Views
Views are often combined with Layouts to create a consistent look and feel across different pages of your application. Layouts act as a wrapper around views and define the common structure of web pages. A layout contains the header, footer, sidebar, and any other elements you want to appear on all pages.
To associate a layout with a view, you set the $layout variable in the controller’s action:
php // src/Controller/PostsController.php class PostsController extends AppController { public function index() { // ... // Set the layout for the index action $this->viewBuilder()->setLayout('default'); } }
In this example, we set the layout to default.ctp, which is usually located in the src/Template/Layout directory. If you don’t explicitly set a layout, CakePHP uses the default layout named default.ctp.
3. Utilizing Layouts
3.1. Customizing Layouts
While using the default layout can be sufficient for many applications, you can easily customize layouts to match your specific design requirements. Layout files are placed in the src/Template/Layout directory and typically contain HTML markup along with placeholders for the View content, denoted by the <?= $this->fetch(‘content’) ?> tag.
Here’s an example of a customized src/Template/Layout/custom.ctp:
html <!-- src/Template/Layout/custom.ctp --> <!DOCTYPE html> <html> <head> <title><?= $this->fetch('title') ?></title> </head> <body> <header> <!-- Your header content here --> </header> <main> <?= $this->fetch('content') ?> </main> <footer> <!-- Your footer content here --> </footer> </body> </html>
To use this custom layout in a controller’s action, you set the layout as shown before:
php // src/Controller/PostsController.php class PostsController extends AppController { public function index() { // ... // Set the layout for the index action $this->viewBuilder()->setLayout('custom'); } }
3.2. Multiple Layouts
In some cases, you may need to use different layouts for different pages. CakePHP allows you to set different layouts for different actions within a controller. For instance:
php // src/Controller/PostsController.php class PostsController extends AppController { public function index() { // ... // Set the layout for the index action $this->viewBuilder()->setLayout('custom'); } public function view($id) { // ... // Set the layout for the view action $this->viewBuilder()->setLayout('view_layout'); } }
In this example, the index action uses the custom.ctp layout, while the view action uses the view_layout.ctp layout.
3.3. Passing Data to Layouts
You can also pass data to layouts, just like you do with views. This can be useful for displaying dynamic content in the header, footer, or other common elements. To pass data to a layout, you use the same set() method:
php // src/Controller/PostsController.php class PostsController extends AppController { public function index() { // ... // Pass data to the custom layout $this->set('siteTitle', 'My CakePHP Blog'); $this->viewBuilder()->setLayout('custom'); } }
Then, in the custom.ctp layout, you can access the siteTitle variable:
html <!-- src/Template/Layout/custom.ctp --> <!DOCTYPE html> <html> <head> <title><?= $this->fetch('title') ?></title> </head> <body> <header> <h1><?= $siteTitle ?></h1> </header> <!-- ... rest of the layout ... --> </body> </html>
4. View Elements
4.1. What are Elements?
View Elements are reusable and modular chunks of code that can be shared across multiple views. Elements help to keep the views clean and organized by separating common components into individual files. They are useful for things like navigation menus, sidebars, alerts, or any other part of the page that appears on multiple screens.
4.2. Creating and Using Elements
To create an element, you can simply create a new file with a .ctp extension in the src/Template/Element directory. For instance, let’s create an element for a reusable alert box:
php <!-- src/Template/Element/alert_box.ctp --> <div class="alert"> <p><?= $message ?></p> </div>
You can then use this element in any view by calling the element() method:
php <!-- src/Template/Posts/index.ctp --> <h1>Welcome to the Posts index page!</h1> <?= $this->element('alert_box', ['message' => 'This is an important message.']) ?> <!-- ... rest of the view ... -->
4.3. Passing Data to Elements
As you can see in the previous example, you can pass data to elements when including them in a view. This allows you to customize the content of the element based on the context in which it is used.
5. Working with Helpers
5.1. Introduction to Helpers
Helpers in CakePHP provide an easy way to generate HTML elements, form elements, and other common output in your views. CakePHP comes with several built-in helpers for common HTML elements, forms, pagination, and more. Additionally, you can create your custom helpers to encapsulate specific functionality that you want to reuse throughout your application.
5.2. Built-in Helpers
Some of the built-in helpers in CakePHP include:
- HtmlHelper: For generating HTML elements like links, images, and meta tags.
- FormHelper: For generating HTML forms and form elements.
- PaginatorHelper: For creating pagination links.
- TimeHelper: For formatting dates and times.
To use a helper, you need to load it in your controller’s action using the $helpers property:
php // src/Controller/PostsController.php class PostsController extends AppController { public $helpers = ['Html', 'Form', 'Paginator']; // ... }
Once loaded, you can access the helper’s methods within your view. For example, using the HtmlHelper to create a link:
php <!-- src/Template/Posts/index.ctp --> <h1>Welcome to the Posts index page!</h1> <p><?= $this->Html->link('Add New Post', ['action' => 'add']) ?></p>
5.3. Creating Custom Helpers
To create a custom helper, you create a new PHP file in the src/View/Helper directory, and it should extend the AppHelper class. Let’s create a simple custom helper that formats text in uppercase:
php // src/View/Helper/MyCustomHelper.php namespace App\View\Helper; use Cake\View\Helper; class MyCustomHelper extends Helper { public function toUppercase($text) { return strtoupper($text); } }
To use the custom helper in your views, you need to load it in your controller’s action:
php // src/Controller/PostsController.php class PostsController extends AppController { public $helpers = ['Html', 'Form', 'Paginator', 'MyCustom']; // ... }
Then, you can use the custom helper in your view:
php <!-- src/Template/Posts/index.ctp --> <h1>Welcome to the Posts index page!</h1> <p><?= $this->MyCustom->toUppercase('hello, world!') ?></p>
6. Best Practices for Organizing Templates
To maintain a clean and organized codebase, consider following these best practices for organizing your templates:
- Use Descriptive File Names: Name your view files, layout files, and element files in a way that clearly indicates their purpose and context. This makes it easier for you and other developers to understand the structure of your application.
- Keep Views Lightweight: Views should primarily focus on rendering data and should not contain complex business logic. Move any complex logic to the controller or model layer for better code separation.
- Reusable Elements: Utilize elements to keep your views modular and reusable. If a component appears on multiple pages, consider creating an element for it.
- Consistent Layouts: Stick to a consistent layout structure across your application to provide a seamless user experience. Use layouts effectively to maintain a common look and feel throughout.
- Leverage Helpers Wisely: Use built-in helpers for common tasks like generating links and forms. For more complex or application-specific functionality, create custom helpers to encapsulate the logic.
- Separation of Concerns: Remember that the View layer is responsible for presentation only. Avoid mixing business logic or database queries in your view files.
Conclusion
CakePHP’s Template System, consisting of Views, Layouts, Elements, and Helpers, offers a powerful and efficient way to create dynamic and visually appealing web applications. By following the principles of separation of concerns and code organization, you can build maintainable and scalable projects.
In this blog, we’ve explored the key components of the Template System and how they work together to provide a clean separation of the presentation layer from the business logic. Understanding Views, Layouts, Elements, and Helpers empowers you as a developer to create stunning web pages with ease and enhance the user experience of your CakePHP applications.
Start leveraging the full potential of CakePHP’s Template System and create engaging web applications that leave a lasting impression on your users! Happy coding!
Table of Contents
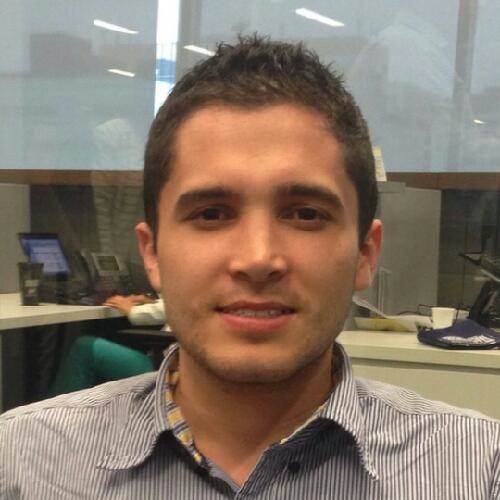
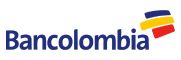