CakePHP and Third-Party APIs: Integration Made Easy
In the world of modern web development, building applications that stand alone is no longer sufficient. Today, applications often need to interact with various third-party services, such as payment gateways, social media platforms, email services, and more. Integrating with these external APIs can be a challenging task, requiring careful handling of data, authentication, and error handling. However, CakePHP, a popular PHP framework, comes to the rescue with its built-in features that make third-party API integration remarkably easy and efficient.
Table of Contents
In this blog post, we’ll explore how CakePHP simplifies the integration of third-party APIs, step by step. We’ll cover the essential aspects, including authentication, making API requests, handling responses, and error management. So, let’s dive in!
1. What is CakePHP?
CakePHP is a free, open-source, and rapid development framework for PHP. It follows the Model-View-Controller (MVC) architectural pattern, making it an ideal choice for building robust web applications. The framework’s primary focus is on simplicity, ease of use, and reducing the amount of code you need to write.
2. Why CakePHP for API Integration?
When it comes to integrating third-party APIs into your application, CakePHP provides several advantages that streamline the process:
- MVC Structure: The MVC architecture helps to keep the code organized and separates the concerns between different components, making it easier to manage API-related logic.
- CakePHP ORM: The Object-Relational Mapping (ORM) feature of CakePHP abstracts the database interactions, allowing you to interact with APIs and handle responses in a similar way you would with a database.
- Component-Based Design: CakePHP’s components offer reusable pieces of code, which can be shared across different controllers and even projects, saving you time and effort.
- Built-in Tools: The framework comes with built-in tools for data validation, security, and caching, which are essential for secure and efficient API integration.
Now, let’s dive into the key steps to integrate third-party APIs into your CakePHP application.
Step 1: Setting Up the Project
First, ensure you have a CakePHP project up and running. If you don’t have one, you can create a new project using the following Composer command:
bash composer create-project --prefer-dist cakephp/app my_api_project
Replace my_api_project with your preferred project name. After the installation is complete, navigate to the project directory:
bash cd my_api_project
Step 2: Installing the HTTP Client
To interact with third-party APIs, we need to install an HTTP client. CakePHP provides an in-built HTTP client based on the PSR-18 HTTP Client standard. This standard ensures interoperability between HTTP clients and servers.
To install the HTTP client, run the following Composer command:
bash composer require cakephp/httpclient
Step 3: Configuring Third-Party API Credentials
Before making API requests, we must configure the API credentials securely. CakePHP allows us to store these credentials in the app.php file, ensuring separation from the source code and improved security. Add the API credentials in config/app.php:
php // config/app.php return [ // Other configuration settings... 'Api' => [ 'MyThirdPartyApi' => [ 'base_url' => 'https://api.example.com', 'api_key' => 'your_api_key_here', // Other API-specific configurations... ] ] ];
Replace ‘https://api.example.com’ with the base URL of the third-party API and ‘your_api_key_here’ with the actual API key.
Step 4: Creating a Component for API Integration
Next, let’s create a custom component to encapsulate the API integration logic. Components are reusable pieces of code that can be attached to controllers. Create a new file ApiComponent.php in the src/Controller/Component directory:
php // src/Controller/Component/ApiComponent.php namespace App\Controller\Component; use Cake\Controller\Component; use Cake\Http\Client; class ApiComponent extends Component { private $httpClient; public function initialize(array $config): void { parent::initialize($config); $this->httpClient = new Client(); } public function get($endpoint, $params = []) { $config = $this->getConfig('MyThirdPartyApi'); $url = $config['base_url'] . $endpoint; try { $response = $this->httpClient->get($url, $params, [ 'headers' => ['Authorization' => 'Bearer ' . $config['api_key']] ]); return $response->getJson(); } catch (\Exception $e) { // Handle API request error... return null; } } }
In this component, we create an HTTP client instance in the initialize method. We also define a get method, which takes the API endpoint and optional parameters as input, and performs the GET request with the required headers.
Step 5: Using the API Component in a Controller
Now that our custom component is ready, we can use it in any controller to make API requests. For demonstration purposes, let’s create a simple ApiController.php and call the get method to fetch data from the third-party API:
php // src/Controller/ApiController.php namespace App\Controller; use App\Controller\AppController; class ApiController extends AppController { public function initialize(): void { parent::initialize(); $this->loadComponent('Api'); } public function fetchUserData() { $endpoint = '/users'; $params = ['limit' => 10]; // Optional parameters $userData = $this->Api->get($endpoint, $params); // Further processing of the $userData... $this->set(compact('userData')); } }
In this example, we load the Api component in the initialize method and then use the get method to fetch user data from the third-party API. You can modify the endpoint and parameters based on the API you are integrating with.
Step 6: Handling API Responses
API responses may vary, and it’s crucial to handle them appropriately. In the ApiComponent.php we created earlier, we added a try-catch block to handle exceptions. When the API request fails, it throws an exception, and we can catch it to handle errors gracefully.
php // src/Controller/Component/ApiComponent.php // ... public function get($endpoint, $params = []) { // ... try { $response = $this->httpClient->get($url, $params, [ 'headers' => ['Authorization' => 'Bearer ' . $config['api_key']] ]); // Check for non-2xx status codes and handle accordingly if ($response->getStatusCode() >= 300) { throw new \Exception('API request failed: ' . $response->getReasonPhrase()); } return $response->getJson(); } catch (\Exception $e) { // Log the error and handle it as per your application's requirements Log::error('API request error: ' . $e->getMessage()); return null; } }
In this updated version of the get method, we check the status code of the response. If it’s not in the 2xx range, we throw an exception with the error message. Additionally, we log the error to help with debugging and future improvements.
Step 7: Handling Authentication
Most third-party APIs require some form of authentication, such as API keys or OAuth tokens. With CakePHP, we can easily manage the authentication process within our custom component. Let’s assume our API requires a Bearer Token for authentication.
php // src/Controller/Component/ApiComponent.php // ... public function authenticate($token) { $this->httpClient = new Client([ 'headers' => ['Authorization' => 'Bearer ' . $token] ]); }
We add an authenticate method to the component, which takes the Bearer Token as input and sets it in the HTTP client’s headers. Now, before making any API requests, we can call the authenticate method to ensure our client is authenticated:
php // src/Controller/ApiController.php // ... public function fetchUserData() { $this->Api->authenticate('your_bearer_token_here'); // ... Rest of the code remains the same ... }
Conclusion
In this blog post, we explored how CakePHP simplifies the integration of third-party APIs by leveraging its MVC architecture, built-in HTTP client, and custom components. We walked through the key steps of setting up a CakePHP project, installing the HTTP client, configuring API credentials, creating a custom component, and handling API responses and errors. With CakePHP, integrating third-party APIs becomes a breeze, saving developers time and effort while ensuring secure and efficient communication with external services.
Now, armed with this knowledge, you can confidently extend your CakePHP applications with a wide range of third-party services, taking your web development projects to the next level.
Happy coding!
Table of Contents
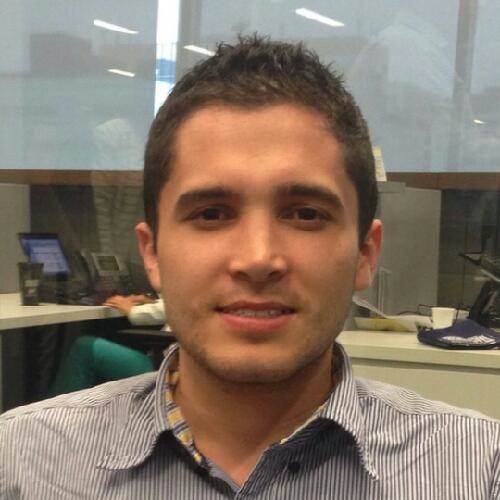
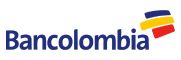