CakePHP Unit Testing: Ensuring Code Quality
In the fast-paced world of web development, delivering high-quality code is crucial to ensure the success of any project. One of the most effective ways to maintain code quality is through unit testing. Unit testing involves testing individual units or components of code to ensure they work as expected and to catch bugs early in the development process. CakePHP, a popular PHP framework, provides robust support for unit testing, making it easier for developers to write tests and maintain code integrity.
In this blog post, we will explore the importance of unit testing in CakePHP, its benefits, best practices, and code examples to illustrate the testing process. Whether you are a seasoned CakePHP developer or just starting, understanding unit testing will significantly enhance your development skills and contribute to the long-term stability of your projects.
1. Why Unit Testing Matters
Unit testing is an essential part of the development process as it offers several significant advantages:
1.1. Early Bug Detection
Writing tests for individual units allows developers to identify bugs early in the development cycle. By catching and addressing issues at this stage, you prevent the bugs from proliferating into complex problems that are difficult to track down and fix later.
1.2. Code Refactoring and Maintenance
Unit tests provide a safety net when refactoring code. When you refactor a piece of code, you can run the associated unit tests to ensure the changes do not break the existing functionality. This helps in keeping the codebase maintainable and ensures that new updates don’t introduce regressions.
1.3. Better Code Design
Writing testable code often leads to better code design. Code that is designed with testing in mind tends to be more modular, cohesive, and loosely coupled, making it easier to understand and maintain.
1.4. Continuous Integration and Delivery (CI/CD)
Automated unit tests are an integral part of any CI/CD pipeline. Running tests automatically during the build process ensures that the application is always in a deployable state and that new changes are thoroughly validated.
2. Setting Up the Environment
Before we dive into writing tests, let’s ensure our development environment is ready for unit testing in CakePHP.
2.1. Prerequisites:
1. CakePHP Project: Ensure you have a functional CakePHP project set up on your local machine.
2. PHPUnit: PHPUnit is the testing framework used for writing and running unit tests in CakePHP. Install it using Composer:
bash composer require --dev phpunit/phpunit
3. Test Suite: Create a test suite configuration file phpunit.xml in the root directory of your project:
xml <?xml version="1.0" encoding="UTF-8"?> <phpunit colors="true"> <testsuites> <testsuite name="CakePHP Test Suite"> <directory>./tests/TestCase</directory> </testsuite> </testsuites> </phpunit>
3. Writing Unit Tests in CakePHP
Now that our environment is set up, let’s proceed with writing unit tests. In CakePHP, tests are usually placed in the tests/TestCase directory. Each class being tested should have a corresponding test case file in this directory.
3.1. Creating a Simple Test
Suppose we have a straightforward Math class with a method add that we want to test. First, let’s create the Math class:
php // File: src/Math.php namespace App; class Math { public function add($a, $b) { return $a + $b; } }
Now, let’s create the test case for the Math class:
php // File: tests/TestCase/MathTest.php namespace App\Test\TestCase; use App\Math; use PHPUnit\Framework\TestCase; class MathTest extends TestCase { public function testAdd() { $math = new Math(); $result = $math->add(2, 3); $this->assertEquals(5, $result); } }
In the MathTest class, we use PHPUnit’s assertions to verify that the add method returns the expected result.
3.2. Running Tests
To execute the tests, run the following command from the root directory of your project:
bash vendor/bin/phpunit
PHPUnit will discover and run all the tests defined in your test suite. If everything is set up correctly, you should see the test output indicating whether the tests pass or fail.
4. Best Practices for CakePHP Unit Testing
To write effective unit tests in CakePHP, consider the following best practices:
4.1. Test One Thing at a Time
Each test method should focus on testing only one aspect of the code. This ensures that the tests are isolated and easier to maintain.
4.2. Use Meaningful Test Method Names
Use descriptive test method names that clearly convey what the test is validating. This makes it easier to identify the purpose of a test, especially when tests fail.
4.3. Test Edge Cases
Test your code with boundary values and edge cases. These tests help uncover unexpected behavior and corner cases that might not be apparent during regular usage.
4.4. Use Mocks and Stubs
When testing code that depends on external resources or APIs, use mocks or stubs to isolate the code from those dependencies. This keeps the tests focused on the unit under test.
4.5. Run Tests Frequently
Run your tests regularly during development to catch issues early and avoid accumulating a large number of failing tests.
Conclusion
Unit testing is a fundamental practice for ensuring code quality and maintaining the stability of CakePHP projects. In this blog post, we’ve explored the importance of unit testing, the benefits it offers, and best practices for writing effective tests in CakePHP. With the right testing approach and tools like PHPUnit, you can significantly improve your development workflow and deliver reliable applications to your users.
By incorporating unit testing into your development process, you’ll gain confidence in your code, identify and fix bugs early, and ultimately create more robust and maintainable applications with CakePHP. So, start writing those tests and enjoy the rewards of a high-quality codebase!
Table of Contents
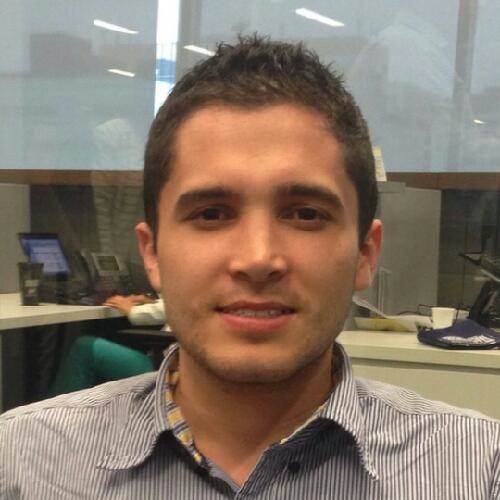
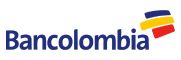