Using CakePHP’s Debugging Tools: Xdebug and Beyond
Debugging is an essential part of the development process. It’s the art of finding and fixing issues in your code, ensuring that your application runs smoothly. When working with CakePHP, you have access to a powerful set of debugging tools that can make your life as a developer much easier. In this blog post, we will explore CakePHP’s debugging capabilities, with a focus on Xdebug and other essential tools that go beyond basic debugging.
Table of Contents
1. Understanding the Importance of Debugging
Before diving into the specifics of CakePHP’s debugging tools, let’s first understand why debugging is crucial. Debugging allows developers to:
- Identify and Fix Bugs: Debugging helps locate and eliminate errors, ensuring your application functions as expected.
- Optimize Performance: It provides insights into your code’s performance bottlenecks, enabling you to optimize it for better efficiency.
- Enhance Security: Debugging can reveal vulnerabilities and security issues that might otherwise go unnoticed.
- Improve Code Quality: By addressing issues as they arise, debugging contributes to overall code quality and maintainability.
2. CakePHP’s Built-in Debugging Features
CakePHP comes with several built-in debugging features that help developers diagnose issues during development.
2.1. Debug Mode
CakePHP’s Debug Mode is a configuration setting that, when enabled, provides detailed error messages and stack traces. To activate it, open your config/app.php file and set the ‘debug’ value to true:
php // config/app.php 'debug' => true,
While Debug Mode is a handy feature, remember to disable it in production for security reasons.
2.2. Debug Kit
Debug Kit is a comprehensive debugging tool provided by CakePHP. It offers a variety of features, including:
- Request and Response panels: Inspect incoming requests and outgoing responses.
- Logging panel: View log entries generated during your application’s execution.
- Variables panel: Monitor variables and their values.
- SQL log panel: Examine executed database queries.
To install Debug Kit, use Composer:
bash composer require --dev cakephp/debug_kit After installation, enable it in your config/bootstrap.php: php Copy code // config/bootstrap.php if (Configure::read('debug')) { Plugin::load('DebugKit', ['bootstrap' => true, 'routes' => true]); }
Debug Kit adds a toolbar to your application’s pages, allowing you to access its features during development.
2.3. Logging
CakePHP has a built-in logging system that lets you record and analyze events in your application. You can use it to track errors, user actions, or any custom events you want to log.
To use CakePHP’s logging, configure it in your config/app.php file:
php // config/app.php 'Log' => [ 'debug' => [ 'className' => 'File', 'path' => LOGS, 'file' => 'debug', 'url' => env('LOG_DEBUG_URL', null), ], // Add more log configurations as needed. ],
You can then log messages like this:
php Log::info('This is an informational message.'); Log::error('An error occurred: ' . $errorMessage);
The logs are stored in the logs/ directory of your CakePHP application.
3. Supercharge Debugging with Xdebug
While CakePHP’s built-in debugging tools are useful, Xdebug takes debugging to the next level. Xdebug is a PHP extension that provides features like:
- Stack traces: Detailed information about where errors occurred.
- Variable inspection: Viewing variables and their values.
- Breakpoints: Pausing code execution at specific points for inspection.
- Remote debugging: Debugging code running on a remote server.
3.1. Installing Xdebug
To get started with Xdebug, you need to install it on your development server. The installation process may vary depending on your server environment. Here’s a general outline:
3.1.1. For PHP on Linux
Install Xdebug via PECL:
bash pecl install xdebug
Add the Xdebug extension to your php.ini file:
ini zend_extension=xdebug.so
Restart your web server or PHP-FPM:
bash sudo service apache2 restart # For Apache sudo service php7.4-fpm restart # For PHP-FPM
3.1.2. For PHP on Windows
Download the Xdebug DLL that matches your PHP version from the Xdebug website.
Add the following line to your php.ini file:
ini zend_extension=path/to/xdebug.dll
Restart your web server (e.g., Apache) or PHP service.
3.2. Configuring Xdebug for CakePHP
To configure Xdebug specifically for CakePHP, make sure your php.ini settings include the following:
ini xdebug.remote_enable = 1 xdebug.remote_autostart = 1 xdebug.remote_port = 9000
These settings enable remote debugging and specify the default port used by Xdebug.
3.3. Remote Debugging with Xdebug
One of the most powerful features of Xdebug is remote debugging, which allows you to debug code running on a remote server from your local development environment. Here’s a step-by-step guide:
- Install Xdebug on the remote server following the steps mentioned earlier.
- Configure your IDE to listen for incoming Xdebug connections. The exact steps depend on your IDE, but most modern IDEs have built-in support for Xdebug.
- Set breakpoints in your code by adding the xdebug_break() function where you want to pause execution.
- Trigger a request to the remote server, either through a web browser or a command-line tool.
- Your IDE should detect the incoming Xdebug connection and stop at the breakpoints you set, allowing you to inspect variables and step through the code.
Xdebug’s remote debugging capability is a game-changer when it comes to tracking down and fixing bugs in your CakePHP applications.
4. Beyond Xdebug: Other Essential Debugging Tools
While Xdebug is a fantastic tool for debugging PHP applications, there are other tools and plugins that can further enhance your debugging experience with CakePHP.
4.1. PHPUnit
PHPUnit is a widely-used testing framework for PHP that can be invaluable for debugging. By writing unit tests for your CakePHP code, you can catch bugs early in the development process and ensure that your code remains stable as you make changes.
To get started with PHPUnit in CakePHP, install the CakePHP testing library:
bash composer require --dev cakephp/cakephp:^4.0
You can then create and run unit tests using PHPUnit’s command-line interface. Writing tests helps you isolate issues and verify that your code behaves as expected.
4.2. Blackfire
Blackfire is a performance profiling tool that helps you identify performance bottlenecks in your CakePHP application. It provides detailed insights into how your code performs and suggests optimizations.
To use Blackfire with CakePHP, install the Blackfire agent and enable it in your server environment. Then, run performance profiles to pinpoint areas of improvement in your application.
4.3. CakePHP Debug Plugin
The CakePHP Debug Plugin is an extension of CakePHP’s built-in debugging features. It offers additional functionalities like email previews, environment variable inspection, and more.
To install the CakePHP Debug Plugin, use Composer:
bash composer require --dev dereuromark/cakephp-debug-kit:^4.0
Then, enable it in your config/bootstrap.php file:
php // config/bootstrap.php if (Configure::read('debug')) { Plugin::load('DebugKit', ['bootstrap' => true, 'routes' => true]); }
The CakePHP Debug Plugin integrates seamlessly with CakePHP’s Debug Kit, providing extra tools for your debugging arsenal.
4.4. Debugging Database Queries
Efficiently debugging database queries is crucial for CakePHP applications. CakePHP provides a Debug class that you can use to log and analyze queries. For example:
php // In your controller or model $this->loadModel('Articles'); $query = $this->Articles->find('all'); $query->enableHydration(false); // Disable hydration for debugging debug($query->sql());
This code will output the SQL query generated by CakePHP, helping you identify and optimize database-related issues.
5. Best Practices for Effective Debugging
To make the most of CakePHP’s debugging tools, consider these best practices:
5.1. Using Breakpoints
Place breakpoints strategically in your code to pause execution and inspect variables and conditions. Breakpoints are incredibly useful for tracking down the root causes of bugs.
5.2. Logging Techniques
Leverage CakePHP’s logging capabilities to record important events, errors, and custom messages. Logging provides a historical view of your application’s behavior.
5.3. Profiling
Use profiling tools like Xdebug and Blackfire to analyze code performance. Profiling helps you identify bottlenecks and optimize your application for speed and efficiency.
Conclusion
CakePHP’s debugging tools, including Xdebug and additional plugins, provide a robust foundation for identifying and resolving issues in your applications. By embracing these tools and best practices, you can streamline your development process, deliver more reliable code, and create a better user experience. Debug like a pro, and take your CakePHP development to the next level!
Table of Contents
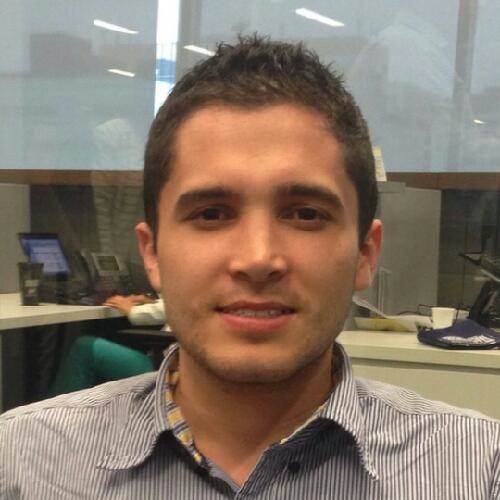
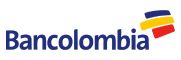