Working with CakePHP’s Utility Classes: Arrays, Strings, and more
In the world of web development, efficiency and organization are key. CakePHP, a popular PHP framework, understands this well and provides a set of utility classes that can significantly simplify your coding process. Among these utilities, Arrays and Strings stand out as fundamental tools for manipulating and managing data. In this blog post, we’ll delve into the functionalities of CakePHP’s utility classes, explore various use cases, and provide you with code samples to jumpstart your development journey.
Table of Contents
1. Introduction to CakePHP Utility Classes
CakePHP is known for its convention-over-configuration approach, which streamlines development by following certain guidelines. Alongside this philosophy, CakePHP offers a range of utility classes that handle common tasks, making code more maintainable and readable. Among these classes, Arrays and Strings are two workhorses that deserve special attention.
2. The Power of Arrays
Arrays are fundamental data structures that store collections of values. CakePHP enhances their functionality through utility classes.
2.1. Basic Array Manipulation
Manipulating arrays becomes seamless with CakePHP’s utility classes. Consider the following example, where we have an array of names and want to capitalize the first letter of each name:
php use Cake\Utility\Arrays; $names = ['john', 'jane', 'michael', 'susan']; $capitalizedNames = Arrays::map($names, function ($value) { return ucfirst($value); }); print_r($capitalizedNames);
The output will be:
csharp Array ( [0] => John [1] => Jane [2] => Michael [3] => Susan )
The Arrays::map() function iterates through each element of the array and applies the provided callback function, transforming the array’s values as needed.
2.2. Filtering and Transforming Arrays
CakePHP’s utility classes also provide methods for filtering and transforming arrays based on certain conditions. For instance, let’s say we have an array of numbers and want to retrieve only the even ones:
php use Cake\Utility\Arrays; $numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; $evenNumbers = Arrays::filter($numbers, function ($value) { return $value % 2 === 0; }); print_r($evenNumbers);
The resulting array will contain only the even numbers:
csharp Array ( [1] => 2 [3] => 4 [5] => 6 [7] => 8 [9] => 10 )
This filtering functionality helps reduce the need for manual iteration and conditional checks.
3. Strings: Beyond the Basics
Strings are at the heart of many web applications. CakePHP’s utility classes offer an array of string-related functions that simplify common operations.
3.1. String Manipulation Functions
Let’s say we need to create SEO-friendly slugs from article titles. CakePHP’s Text class comes to the rescue:
php use Cake\Utility\Text; $title = "Mastering_CakePHP's_Utility_Classes"; $slug = Text::slug($title); echo $slug;
The output will be:
mastering-cakephps-utility-classes
The Text::slug() function converts the input string into a URL-friendly format, which is a common task in web development.
3.2. Validation and Formatting
CakePHP’s utility classes also offer features for string validation and formatting. For example, let’s say we need to validate whether an email address is properly formatted:
php use Cake\Utility\Text; $email = "example.com"; $isEmailValid = Text::isEmail($email); if ($isEmailValid) { echo "Email is valid."; } else { echo "Email is not valid."; }
This function, Text::isEmail(), performs a basic email format check and returns a boolean result.
4. Working with Hashes
Hashing is crucial for security, and CakePHP’s utility classes provide hashing functions that are easy to use. For instance, consider the need to hash a user’s password before storing it in the database:
php use Cake\Utility\Security; $password = "secretpassword"; $hashedPassword = Security::hash($password); echo $hashedPassword;
The output will be a securely hashed version of the original password. Hashing is essential for protecting sensitive data and is a fundamental practice in web development.
5. Convenience Functions for File Handling
Working with files is a common task in web applications, and CakePHP offers utility classes to simplify file-related operations. For example, consider the need to get the file extension from a given filename:
php use Cake\Utility\File; $filename = "document.pdf"; $extension = File::ext($filename); echo $extension;
The output will be:
This function, File::ext(), extracts the file extension from the given filename.
6. Internationalization Made Easy
For applications catering to a global audience, internationalization (i18n) is essential. CakePHP’s utility classes support internationalization with features like translating strings based on the selected locale. Here’s a simple example:
php use Cake\I18n\I18n; I18n::setLocale('fr_FR'); $translated = __('Hello, world!'); echo $translated;
Assuming a French locale translation is available, this code will output the French equivalent of “Hello, world!”
7. Best Practices for Effective Utilization
While CakePHP’s utility classes offer powerful tools, effective utilization requires following some best practices:
- Read the Documentation: Familiarize yourself with the utility classes’ documentation to fully understand their capabilities and functions.
- Stay Consistent: When working on a CakePHP project, make consistent use of the utility classes to maintain a standardized codebase.
- Test Thoroughly: Just like any other code, thoroughly test your utilization of the utility classes to ensure they work as expected.
- Keep it Simple: Don’t overcomplicate things. Use the utility classes when they genuinely simplify your code, but avoid unnecessary abstraction.
Conclusion
CakePHP’s utility classes, especially Arrays and Strings, provide developers with a powerful toolkit to streamline common tasks and operations. By leveraging these utility classes, you can enhance your code’s efficiency, readability, and maintainability. From array manipulation to string formatting, from file handling to internationalization, these classes cover a wide range of functionalities that can significantly boost your web development process. As you embark on your CakePHP journey, don’t overlook these hidden gems that can make your coding life so much easier.
Table of Contents
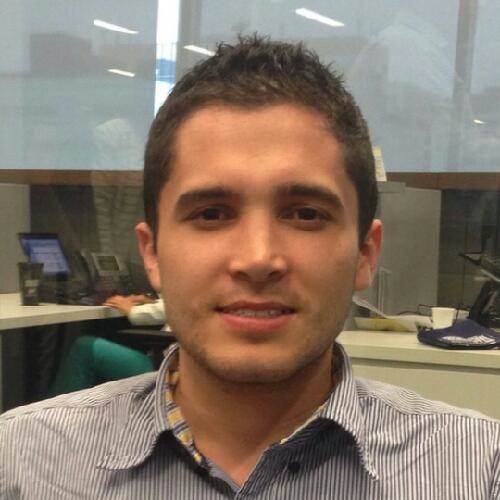
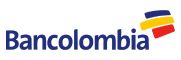