Implementing Web Scraping in CakePHP: Data Extraction
Web scraping has become an indispensable tool for gathering data from the vast expanse of the internet. Whether you’re a business owner looking to collect market data, a researcher analyzing trends, or simply a curious individual, web scraping can provide you with valuable insights. In this blog post, we’ll explore how to implement web scraping in CakePHP, a popular PHP framework, to extract data from websites effectively. We’ll cover the basics of web scraping, the tools and libraries you can use, and provide practical examples to get you started.
Table of Contents
1. What is Web Scraping?
1.1. Understanding Web Scraping
Web scraping, also known as web harvesting or web data extraction, is the process of extracting data from websites. It involves making HTTP requests to web pages, retrieving the HTML content, and then parsing and extracting specific information from that HTML. This extracted data can be used for various purposes, such as data analysis, research, or populating your own databases.
Web scraping is incredibly versatile and can be used to gather information from a wide range of sources, including news articles, product listings, social media profiles, and more. It provides access to data that might not be available through APIs or other means, making it a valuable tool for many applications.
1.2. Legality and Ethics
While web scraping offers numerous benefits, it’s essential to approach it with ethics and legality in mind. Before scraping a website, you should check if the website’s terms of service allow scraping and adhere to the rules set by the website’s robots.txt file. Additionally, be respectful of a site’s server resources by not overloading it with requests, which could lead to denial of service.
2. Getting Started with CakePHP
2.1. Setting up Your CakePHP Project
Before diving into web scraping, you need to set up a CakePHP project. If you haven’t already, follow these steps:
Install Composer, a PHP dependency manager, if you haven’t already.
Use Composer to create a new CakePHP project:
bash composer create-project --prefer-dist cakephp/app myproject
Navigate to your project directory:
bash cd myproject
2.2. Understanding Models and Controllers
In CakePHP, the Model-View-Controller (MVC) architecture is followed. Models represent your data and interact with the database, Views handle the presentation, and Controllers manage the application’s logic.
For web scraping, you’ll mainly work with Controllers. Controllers handle incoming requests, perform the scraping, and send the extracted data to the views for rendering.
3. Choosing the Right Scraping Library
3.1. Introduction to Scraping Libraries
CakePHP doesn’t come with built-in web scraping functionality, but you can easily integrate third-party PHP scraping libraries. Two popular options are:
- Goutte: Goutte is a simple web scraper built on top of Guzzle HTTP client. It provides an easy-to-use API for crawling websites and extracting data.
- Symfony Panther: Symfony Panther is another scraping library that utilizes the Symfony browser automation component. It allows you to interact with web pages as if you were using a real web browser.
3.2. Selecting the Best Library for CakePHP
The choice between Goutte and Symfony Panther depends on your specific requirements. Goutte is more suitable for simple scraping tasks, while Symfony Panther is ideal for scenarios where you need to interact with JavaScript-driven websites.
For this tutorial, we’ll use Goutte for its simplicity and ease of integration with CakePHP.
4. Implementing Web Scraping in CakePHP
4.1. Installing the Chosen Scraping Library
To install Goutte, use Composer:
bash composer require fabpot/goutte
This command will add Goutte to your project’s dependencies.
4.2. Creating a Scraping Controller
In CakePHP, controllers are responsible for handling HTTP requests and responses. To create a scraping controller, use the following CakePHP command:
bash bin/cake bake controller Scraping
This command will generate a ScrapingController.php file in your src/Controller directory.
4.3. Writing Your First Scraping Code
Open ScrapingController.php and add the following code to set up Goutte and scrape a website:
php use Goutte\Client; use Symfony\Component\DomCrawler\Crawler; class ScrapingController extends AppController { public function index() { // Create a Goutte client $client = new Client(); // Specify the URL to scrape $url = 'https://example.com'; // Send an HTTP GET request $crawler = $client->request('GET', $url); // Extract data from the page $data = $crawler->filter('h1')->text(); // Display the extracted data $this->set('data', $data); } }
In this code:
- We import the necessary classes from Goutte and Symfony.
- The index method sets up a Goutte client, specifies the URL to scrape, and sends an HTTP GET request.
- We then use the filter method to extract data from the page. In this example, we’re extracting the text of the first <h1> element on the page.
- Finally, we set the extracted data to be passed to the view for rendering.
5. Handling Data Extraction
5.1. Parsing HTML with Simple HTML DOM Parser
Web scraping often involves parsing and navigating HTML documents. To make this process easier, you can use libraries like Simple HTML DOM Parser. You can install it using Composer:
bash composer require sunra/php-simple-html-dom-parser
Once installed, you can use it to parse HTML content:
php use Sunra\PhpSimple\HtmlDomParser; // Parse an HTML string $html = HtmlDomParser::str_get_html('<div class="content">Hello, World!</div>'); // Find an element by class $element = $html->find('.content', 0); // Get the element's text $text = $element->plaintext;
5.2. Extracting Data Elements
When scraping a webpage, you’ll often need to extract specific data elements, such as links, images, or tables. Goutte and Symfony Panther provide methods for selecting and extracting these elements based on CSS selectors.
For example, to extract all the links (<a> tags) from a webpage:
php $links = $crawler->filter('a')->links(); foreach ($links as $link) { // Get the URL of the link $url = $link->getUri(); // Get the text of the link $text = $link->getText(); }
5.3. Storing Scraped Data
Once you’ve extracted data from a website, you may want to store it in a database or a file for future use. CakePHP provides built-in support for working with databases using models. You can create a model for your scraped data and use it to save the information to your database.
6. Dealing with Challenges
6.1. Handling Pagination
Many websites display data across multiple pages, requiring you to navigate through paginated content. To scrape such websites, you’ll need to implement pagination logic in your CakePHP controller. This typically involves iterating through the pages and scraping data from each page.
6.2. Handling Dynamic Websites
Some websites rely heavily on JavaScript to load content dynamically. Libraries like Goutte may not be suitable for scraping these sites, as they don’t execute JavaScript. In such cases, you may need to consider using headless browsers or tools like Puppeteer to interact with the website as a real user.
6.3. Managing Rate Limits
Websites may have rate limits or anti-scraping measures in place to prevent excessive traffic from scrapers. To avoid getting blocked, implement rate limiting and respect any delay recommendations specified in the website’s robots.txt file. You can use CakePHP’s built-in features for scheduling and throttling scraping requests.
7. Best Practices for Web Scraping in CakePHP
7.1. Respect Robots.txt
Always check a website’s robots.txt file before scraping. This file specifies which parts of the site are off-limits to web crawlers. Respecting robots.txt not only ensures you’re scraping ethically but also reduces the risk of being blocked by the website.
7.2. Use User Agents
Set a user agent for your scraping requests to identify your scraper to the website’s server. Some websites may block scrapers with generic user agents, so it’s a good practice to use a user agent that resembles a real web browser.
7.3. Error Handling and Logging
Implement robust error handling in your CakePHP scraping application. Log errors and exceptions to help troubleshoot issues and monitor the scraping process. Additionally, consider implementing retries and backup strategies for handling intermittent network errors.
Conclusion
Web scraping in CakePHP opens up a world of possibilities for collecting and utilizing data from the web. By choosing the right scraping library, understanding data extraction techniques, and following best practices, you can harness the power of web scraping to extract valuable information for your projects. Just remember to scrape responsibly, respecting the rules and guidelines set by websites, and always prioritize the ethical and legal aspects of web scraping. Happy scraping!
In this blog post, we’ve covered the fundamentals of implementing web scraping in CakePHP, from setting up your project to handling complex scraping scenarios. Armed with this knowledge, you can embark on your web scraping journey and unlock a wealth of data waiting to be discovered on the internet.
Table of Contents
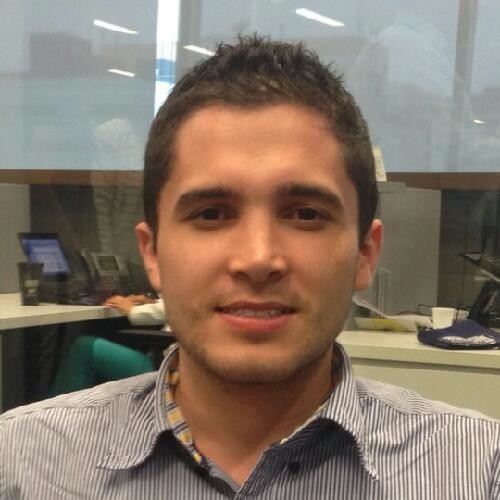
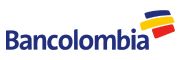