Implementing Webhooks in CakePHP: Handling External Events
In the dynamic landscape of web development, real-time data updates and seamless integrations have become the norm rather than the exception. Whether it’s updating user information, synchronizing content across platforms, or triggering specific actions, the ability to receive and process external events in a timely manner is crucial. This is where webhooks come into play. Webhooks allow your application to receive HTTP callbacks or POST requests from external sources when certain events occur, enabling you to keep your data and processes up to date without constant polling.
Table of Contents
In this tutorial, we will explore the implementation of webhooks in CakePHP, a popular PHP framework known for its flexibility and robustness. We will cover the entire process, from setting up the webhook endpoints to handling and processing external events within the CakePHP application.
1. Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites in place:
- Basic understanding of PHP and CakePHP.
- CakePHP project already set up.
- A code editor for making necessary changes to your CakePHP project files.
2. Setting Up Webhook Endpoints
The first step in implementing webhooks is to set up the endpoints that will receive the external events. These endpoints are URLs within your CakePHP application that will listen for incoming HTTP callbacks. Let’s create a controller that will handle these webhook requests.
2.1. Creating the Webhooks Controller
In your CakePHP project, navigate to the src/Controller directory and create a new file called WebhooksController.php. This controller will contain the actions for handling webhook events.
php // src/Controller/WebhooksController.php namespace App\Controller; use App\Controller\AppController; class WebhooksController extends AppController { public function initialize(): void { parent::initialize(); $this->loadModel('Webhooks'); // Load the Webhooks model } public function receive() { $this->autoRender = false; // Disable view rendering // Retrieve the incoming POST data $payload = $this->request->input('json_decode', true); // Process the payload (implement your logic here) // ... // Send a response (if required) $this->response = $this->response->withStatus(200); return $this->response; } }
In the above code, we define a receive action within the WebhooksController. This action disables view rendering ($this->autoRender = false) since we don’t need to render a view for webhook requests. We retrieve the incoming JSON payload using $this->request->input(‘json_decode’, true) and process it as needed.
2.2. Configuring Routes
Next, we need to configure the routes to map incoming webhook requests to the receive action in the WebhooksController. Open the config/routes.php file and add the following route:
php // config/routes.php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::scope('/', function (RouteBuilder $routes) { $routes->connect('/webhooks/receive', ['controller' => 'Webhooks', 'action' => 'receive']); });
In this configuration, any incoming requests to the URL /webhooks/receive will be directed to the receive action in the WebhooksController.
3. Handling and Processing Webhook Events
With the webhook endpoint set up, the next step is to handle and process the incoming webhook events. The payload received in the receive action contains information about the event, and you can implement your custom logic to respond to these events.
3.1. Processing the Payload
Let’s say you want to handle a scenario where the webhook event notifies your application about a user’s updated profile information. You can extract relevant data from the payload and update the user’s information in your database.
php // src/Controller/WebhooksController.php public function receive() { // ... (previous code) // Process the payload (example: updating user profile) $eventType = $payload['event_type']; $userId = $payload['user_id']; $updatedData = $payload['updated_data']; if ($eventType === 'user_profile_updated') { $user = $this->Users->get($userId); $user = $this->Users->patchEntity($user, $updatedData); if ($this->Users->save($user)) { // User profile updated successfully } else { // Failed to update user profile } } // ... (remaining code) }
In this example, we assume that the payload contains an event_type, user_id, and updated_data. If the event type corresponds to a “user_profile_updated” event, the controller retrieves the user’s existing record, patches it with the updated data, and saves it back to the database.
3.2. Ensuring Security
Security is paramount when dealing with webhooks, as they involve external sources making requests to your application. To enhance security, you can implement request validation and verification techniques such as verifying signatures or using API keys.
php // src/Controller/WebhooksController.php public function receive() { $this->autoRender = false; // Verify the request (implement your validation logic) $isValidRequest = $this->verifyRequest($this->request); if (!$isValidRequest) { $this->response = $this->response->withStatus(401); // Unauthorized return $this->response; } // ... (processing payload) $this->response = $this->response->withStatus(200); return $this->response; } private function verifyRequest($request) { // Implement your verification logic here // Return true if the request is valid, false otherwise }
In the verifyRequest method, you can implement your validation logic to ensure that the incoming request is legitimate. This could involve checking request headers, verifying signatures, or comparing API keys.
Conclusion
Implementing webhooks in CakePHP opens up a world of possibilities for integrating your application with external services and reacting to real-time events. By setting up webhook endpoints and handling incoming payloads effectively, you can streamline data updates, automate processes, and enhance the user experience. Remember to prioritize security by implementing validation and verification mechanisms to safeguard your application from unauthorized requests. With this knowledge in hand, you’re well-equipped to harness the power of webhooks and take your CakePHP application to the next level. Happy coding!
Table of Contents
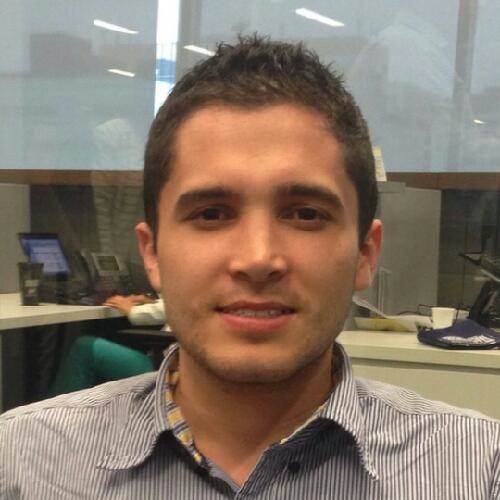
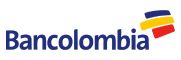