Implementing WebSockets in CakePHP: Real-Time Communication
In the world of web development, delivering a seamless and interactive user experience has become a crucial aspect of creating successful applications. Users expect real-time updates and instant interactions, which can be a challenge to achieve using traditional HTTP requests. This is where WebSockets come into play. WebSockets provide a persistent connection between the client and the server, allowing for bi-directional communication without the overhead of repeatedly opening and closing connections. In this article, we will explore how to implement WebSockets in CakePHP to achieve real-time communication and elevate your application’s capabilities.
Table of Contents
1. Understanding WebSockets: A Brief Overview
Before diving into the implementation details, let’s briefly understand what WebSockets are and why they are a game-changer for real-time communication.
1.1. What are WebSockets?
WebSockets are a communication protocol that provides full-duplex, bidirectional communication channels over a single TCP connection. Unlike traditional HTTP requests that follow the request-response pattern, WebSockets keep the connection open, allowing both the client and the server to send messages to each other at any time. This makes WebSockets ideal for scenarios where instant updates are crucial, such as real-time chats, live notifications, collaborative applications, and more.
1.2. Advantages of WebSockets:
- Real-Time Updates: WebSockets enable real-time data exchange, ensuring that users receive instant updates without the need to repeatedly poll the server.
- Reduced Latency: The persistent connection established by WebSockets eliminates the latency introduced by repeatedly opening and closing connections for each request.
- Efficient: WebSockets are more efficient than traditional HTTP polling, as they significantly reduce the overhead associated with headers and connection establishment.
- Bi-Directional: Both the client and the server can send messages to each other independently, allowing for interactive and dynamic applications.
2. Setting Up a CakePHP Project
Before we proceed, make sure you have a CakePHP project up and running. If not, you can quickly set up a CakePHP project using Composer:
bash composer create-project --prefer-dist cakephp/app my-realtime-app
3. Integrating WebSockets in CakePHP
To implement WebSockets in CakePHP, we will be using the cakephp/websocket package. This package provides the necessary tools to handle WebSocket connections and events within your CakePHP application.
Step 1: Installation
Start by installing the cakephp/websocket package using Composer:
bash composer require cakephp/websocket
Step 2: Configuration
Next, configure the WebSocket component in your CakePHP application. Open the config/bootstrap.php file and add the following lines:
php use Cake\Core\Configure; use Cake\Http\MiddlewareQueue; use Cake\Routing\RouteBuilder; // ... // WebSocket configuration Configure::write('WebSocket', [ 'my-realtime-app' => [ 'className' => 'MyRealtimeApp\WebSocket\MyRealtimeAppSocket', 'host' => 'localhost', 'port' => 3001, 'origin' => 'http://localhost:8765', ], ]);
In the above configuration, replace ‘MyRealtimeApp\WebSocket\MyRealtimeAppSocket’ with the appropriate namespace and class name for your WebSocket implementation.
Step 3: Creating WebSocket Handlers
WebSocket handlers are responsible for handling incoming WebSocket connections and events. Create a new file named MyRealtimeAppSocket.php in the src/WebSocket directory of your application. Here’s an example of what the handler might look like:
php namespace MyRealtimeApp\WebSocket; use Cake\WebSocket\WebSocketHandlerInterface; class MyRealtimeAppSocket implements WebSocketHandlerInterface { public function handleHandshake($request, $response) { // Handle WebSocket handshake } public function handleConnection($socket) { // Handle new WebSocket connection } public function handleData($socket, $data) { // Handle incoming WebSocket data } public function handleClose($socket) { // Handle WebSocket connection close } }
In this example, each method corresponds to a different stage of WebSocket communication: handshake, connection, data reception, and connection closure. Customize these methods according to your application’s needs.
Step 4: Running the WebSocket Server
To run the WebSocket server, use the following command:
bash bin/cake WebSocket
4. Implementing Real-Time Features
With the WebSocket server up and running, you can now implement real-time features in your CakePHP application. Let’s consider a simple example of a real-time notification system.
4.1. Broadcasting Notifications
Assuming you want to send real-time notifications to users, you can modify your MyRealtimeAppSocket handler’s handleConnection method:
php public function handleConnection($socket) { // Get the user's ID from the WebSocket handshake or authentication $userId = /* Retrieve user's ID */; // Store the connection in a data structure, e.g., an array or Redis $this->connections[$userId] = $socket; }
Now, whenever you need to send a notification, you can retrieve the user’s connection from the connections array and send data:
php $userId = /* User's ID */; $message = /* Notification message */; $socket = $this->connections[$userId]; $socket->send(json_encode(['type' => 'notification', 'message' => $message]));
Conclusion
Implementing WebSockets in CakePHP opens the door to a wide range of possibilities for real-time communication in your applications. Whether you’re building a live chat, collaborative editing tool, or any other interactive feature, WebSockets provide the efficiency and responsiveness that modern users expect. By following the steps outlined in this article, you can seamlessly integrate WebSockets into your CakePHP project and elevate the user experience to a whole new level. Real-time communication is no longer a luxury; it’s a necessity in today’s web development landscape.
Table of Contents
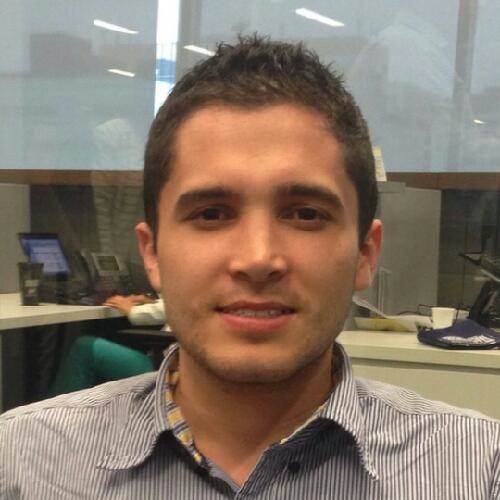
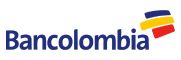