What is CodeIgniter’s query builder, and how does it simplify database queries?
CodeIgniter’s Query Builder is a powerful and user-friendly feature that simplifies the process of constructing database queries in PHP applications. It provides an object-oriented way to build SQL queries, making it easier to interact with databases without the need to write raw SQL statements. Here’s how CodeIgniter’s Query Builder simplifies database queries:
- Abstraction of SQL:** Query Builder abstracts the SQL language, allowing developers to create complex database queries using PHP methods and functions. This abstraction makes it easier to write database queries without having to learn the intricacies of SQL syntax.
- Readability and Maintainability:** By using Query Builder, queries become more readable and maintainable. Developers can chain methods and functions to build queries step by step, making it clear what each part of the query does. This enhances code comprehension and reduces the chances of errors.
- Security: CodeIgniter’s Query Builder also helps prevent SQL injection attacks by automatically escaping user inputs, ensuring that data is treated as data and not executable SQL code. This security feature is crucial for protecting your application’s database from malicious input.
- Database Independence: Query Builder is database-agnostic, which means it can be used with various database management systems (DBMS) supported by CodeIgniter, such as MySQL, PostgreSQL, SQLite, and more. Developers can write queries without worrying about the specific SQL syntax of the underlying DBMS.
- Dynamic Query Building: With Query Builder, developers can easily create dynamic queries based on user input or application logic. For example, you can conditionally add or remove clauses, change table names, or modify query parameters as needed.
- Method Chaining: One of the most convenient features of Query Builder is method chaining. You can chain multiple methods together to build complex queries in a single line of code, enhancing code efficiency and reducing verbosity.
Here’s a simple example of how Query Builder can be used to retrieve data from a hypothetical “products” table:
```php $this->db->select('product_name, price') ->from('products') ->where('category', 'Electronics') ->order_by('price', 'asc') ->limit(10); $query = $this->db->get(); // $query now holds the result set ```
In this example, we’ve used method chaining to build a query that selects product names and prices from the “products” table, filters by category, orders the results by price in ascending order, and limits the number of results to 10.
CodeIgniter’s Query Builder is a valuable tool for simplifying database interactions in PHP applications. It enhances code readability, security, and maintainability while providing a flexible and database-agnostic way to construct SQL queries. This feature is particularly useful for developers working with CodeIgniter, making database operations more efficient and less error-prone.
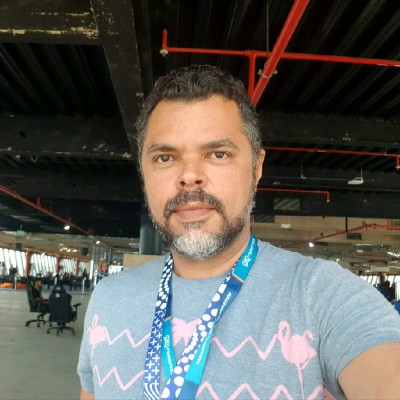
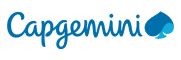