Asynchronous Programming with Dart: Handling Concurrency
In the world of modern software development, building responsive and efficient applications is crucial to providing a seamless user experience. One of the key challenges developers face is managing concurrency, where multiple tasks need to be executed concurrently without causing conflicts or slowdowns. Dart, a versatile programming language developed by Google, provides powerful tools for handling concurrency through asynchronous programming techniques. In this blog post, we’ll delve into the world of asynchronous programming with Dart and explore how to effectively handle concurrency.
Table of Contents
1. Understanding Asynchronous Programming
Before we dive into the specifics of asynchronous programming in Dart, let’s first understand what it entails. In traditional synchronous programming, code executes sequentially – one line after another. However, in scenarios where tasks might take some time to complete, such as network requests or file I/O operations, synchronous code can lead to unresponsive applications.
Asynchronous programming addresses this issue by allowing tasks to run independently of each other. This means that while one task is waiting for a resource (e.g., data from a server), another task can continue executing, preventing the entire application from freezing. Asynchronous programming greatly enhances the responsiveness and efficiency of applications.
2. Dart’s Asynchronous Programming Tools
Dart provides a range of tools and keywords for writing asynchronous code:
2.1. Future and async/await
The Future class in Dart represents a potential value or error that will be available at some point in the future. When combined with the async and await keywords, you can write asynchronous code that reads almost like synchronous code.
Here’s a simple example of using Future and async/await to fetch data from a hypothetical API:
dart Future<String> fetchData() async { // Simulate network delay await Future.delayed(Duration(seconds: 2)); return 'Fetched data'; } void main() async { print('Fetching data...'); String data = await fetchData(); print(data); }
In this example, the fetchData function simulates a network delay using Future.delayed. The await keyword ensures that the function doesn’t block the main thread while waiting for the data to be fetched.
2.2. Streams
Dart’s Stream and StreamController classes are used for handling sequences of asynchronous events. Streams are ideal for scenarios where data is continuously flowing, such as real-time updates or user interactions.
Let’s look at a basic usage of streams:
dart import 'dart:async'; Stream<int> countNumbers(int max) async* { for (int i = 1; i <= max; i++) { yield i; await Future.delayed(Duration(seconds: 1)); } } void main() { final stream = countNumbers(5); stream.listen((number) { print('Number: $number'); }); }
In this example, the countNumbers function generates a stream of numbers from 1 to the specified maximum. The async* modifier allows the function to use the yield keyword, which emits values asynchronously. The listen method is used to listen for events emitted by the stream.
3. Handling Concurrency with Asynchronous Programming
Now that we have an understanding of Dart’s asynchronous programming tools, let’s explore how to handle concurrency effectively using these tools.
3.1. Parallel Execution
Dart’s asynchronous programming tools enable parallel execution of tasks, which is particularly useful for tasks that can be performed independently. For example, if you need to fetch data from multiple APIs, you can use the Future.wait function to execute these tasks concurrently:
dart Future<void> fetchData(String url) async { // Fetch data from the given URL } void main() async { final urls = ['url1', 'url2', 'url3']; final futures = urls.map((url) => fetchData(url)); await Future.wait(futures); print('All data fetched'); }
In this example, the fetchData function fetches data from a given URL. By mapping the list of URLs to a list of futures and using Future.wait, you ensure that all the data fetching tasks run concurrently.
3.2. Throttling and Debouncing
Throttling and debouncing are techniques used to control the rate at which certain actions are executed. Throttling limits the number of times a function is called over a specific time interval, while debouncing delays the execution of a function until a certain amount of time has passed since the last invocation.
These techniques are useful in scenarios such as handling user input or controlling the frequency of network requests.
Here’s a simplified example of throttling:
dart void throttle(Function function, Duration duration) { Timer? timer; return () { if (timer == null) { function(); timer = Timer(duration, () { timer = null; }); } }; } void main() { final throttledPrint = throttle(() => print('Throttled action'), Duration(seconds: 2)); for (int i = 0; i < 5; i++) { throttledPrint(); } }
In this example, the throttle function wraps an action function and ensures that it can only be executed once within a specified time interval. This is useful for scenarios where you want to prevent excessive or rapid execution of a function.
3.3. Error Handling
When dealing with asynchronous tasks, error handling becomes essential to ensure the stability of your application. Dart provides mechanisms to catch and handle errors in asynchronous code, ensuring that the application doesn’t crash unexpectedly.
The try-catch block can be used to catch errors thrown in asynchronous functions:
dart Future<void> fetchData() async { throw Exception('An error occurred'); } void main() async { try { await fetchData(); } catch (e) { print('Error: $e'); } }
In this example, the fetchData function throws an exception, which is caught and handled in the try-catch block.
Conclusion
Asynchronous programming is a powerful paradigm that enables developers to build responsive and efficient applications. Dart’s asynchronous programming tools, such as Future, async/await, and streams, provide the means to manage concurrency effectively. By harnessing these tools, you can create applications that handle multiple tasks concurrently, ensuring a smooth and uninterrupted user experience. Whether it’s fetching data from APIs, managing user input, or dealing with real-time updates, Dart’s asynchronous programming capabilities empower you to build modern, responsive applications that excel in today’s fast-paced digital landscape.
Table of Contents
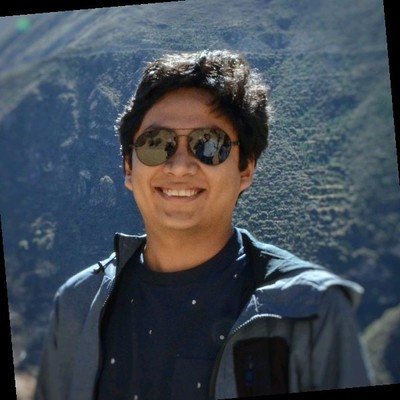
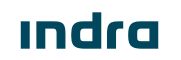