Websockets in Dart: Real-Time Communication in Web Applications
In the ever-evolving landscape of web development, real-time communication has become a crucial aspect of creating dynamic and engaging web applications. Traditional HTTP requests and responses are often not sufficient to provide the seamless, interactive experience users expect. This is where WebSockets come into play. WebSockets allow for bidirectional, low-latency communication between a client (typically a web browser) and a server. In this blog, we’ll dive into WebSockets in Dart, a programming language optimized for building web applications. We’ll explore the benefits of using WebSockets, delve into their implementation in Dart, and provide code samples to get you started on integrating real-time communication into your web projects.
Table of Contents
1. Benefits of WebSockets:
Before we delve into Dart’s implementation of WebSockets, let’s take a moment to understand the benefits they bring to web applications.
1.1. Real-Time Updates:
Traditional HTTP communication involves the client sending a request and the server responding with data. This creates a delay between each request, making it challenging to achieve real-time updates. WebSockets, on the other hand, establish a persistent connection, enabling instant communication between the client and server. This makes them ideal for applications that require live updates, such as messaging apps, collaborative tools, and live streaming platforms.
1.2. Reduced Latency:
WebSockets significantly reduce latency compared to traditional HTTP polling. With WebSockets, data can be pushed from the server to the client as soon as it’s available, eliminating the need for the client to repeatedly request updates. This results in a more responsive and efficient user experience.
1.3. Bi-directional Communication:
Unlike HTTP, which primarily supports uni-directional communication (client sends requests, server sends responses), WebSockets allow for bi-directional communication. Both the client and server can send messages to each other at any time, enabling real-time interactions that go beyond simple request-response patterns.
1.4. Efficient Data Transfer:
WebSockets use a binary protocol that is more compact and efficient than the text-based protocols used in HTTP. This leads to reduced overhead and improved performance, especially when dealing with frequent data transfers in real-time applications.
2. Implementing WebSockets in Dart:
Step 1: Setting Up a Dart Project:
To get started with WebSockets in Dart, you’ll need a Dart project. If you don’t already have one, you can create a new Dart project using the Dart SDK or popular IDEs like Visual Studio Code.
Step 2: Importing the WebSocket Package:
Dart provides a built-in dart:io library that includes classes for working with WebSockets. To use this library, import it into your Dart file:
dart import 'dart:io';
Step 3: Creating a WebSocket Server:
To set up a WebSocket server in Dart, you can use the HttpServer class from the dart:io library. Here’s an example of how to create a simple WebSocket server:
dart void main() async { final server = await HttpServer.bind('localhost', 8080); print('WebSocket server started on port 8080'); server.listen((request) { if (WebSocketTransformer.isUpgradeRequest(request)) { WebSocketTransformer.upgrade(request).then((webSocket) { webSocket.listen((message) { print('Received message: $message'); webSocket.add('Server received your message: $message'); }); }); } }); }
In this example, the server listens for WebSocket upgrade requests and establishes WebSocket connections. It then listens for incoming messages from clients and sends a response back to each client.
Step 4: Creating a WebSocket Client:
On the client side, you can create a WebSocket connection using the WebSocket class from the dart:html library. Here’s an example of how to set up a WebSocket client in Dart:
dart import 'dart:html'; void main() { final webSocket = WebSocket('ws://localhost:8080'); webSocket.onOpen.listen((event) { print('WebSocket connected'); webSocket.send('Hello, Server!'); }); webSocket.onMessage.listen((event) { print('Received message: ${event.data}'); }); webSocket.onClose.listen((event) { print('WebSocket disconnected'); }); }
In this client example, we connect to the WebSocket server and listen for events like onOpen, onMessage, and onClose. When the connection is established, we send a message to the server and handle incoming messages.
Step 5: Bidirectional Communication:
WebSockets shine in their ability to support bidirectional communication. Both the server and the client can send messages to each other at any time. To demonstrate this, let’s extend our previous examples:
Server:
dart // Inside the server's WebSocket listener webSocket.listen((message) { print('Received message: $message'); webSocket.add('Server received your message: $message'); });
Client:
dart // Inside the client's WebSocket event listeners webSocket.onOpen.listen((event) { print('WebSocket connected'); webSocket.send('Hello, Server!'); webSocket.send('How are you?'); }); webSocket.onMessage.listen((event) { print('Received message: ${event.data}'); if (event.data == 'Server received your message: How are you?') { webSocket.send('I am doing great!'); } });
In this extended example, the client sends two messages to the server after connecting. The server responds to each message, and the client handles the responses accordingly.
Conclusion
WebSockets in Dart offer a powerful solution for achieving real-time communication in web applications. With their ability to provide instant updates, reduced latency, and bi-directional communication, WebSockets enable developers to create dynamic and engaging user experiences. By understanding the benefits and implementing WebSockets using Dart’s built-in libraries, you can seamlessly integrate real-time communication into your web projects. Whether you’re building a chat application, a collaborative tool, or a live data visualization platform, WebSockets will undoubtedly play a vital role in delivering the real-time interactions your users demand.
Table of Contents
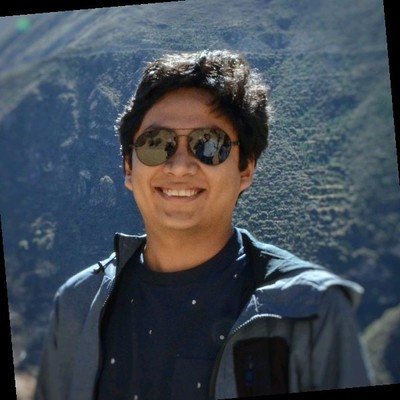
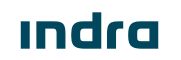