Dart for Blockchain: Developing Decentralized Applications
Blockchain technology has taken the world by storm, revolutionizing industries and reshaping our understanding of trust and transparency in digital transactions. One of the most significant aspects of blockchain is its decentralized nature, enabling the creation of decentralized applications (DApps). These applications run on a distributed network of computers, making them resistant to censorship and single points of failure.
In this blog, we will explore how to develop decentralized applications using Dart, a versatile and powerful programming language created by Google. Dart provides an excellent environment for building blockchain applications, offering high-performance, great tooling, and a strong developer community.
1. Understanding Decentralized Applications (DApps):
Before diving into Dart and blockchain, it’s crucial to grasp the fundamental concepts of decentralized applications. DApps are applications that operate on a peer-to-peer network of nodes, ensuring a consensus-driven, decentralized approach to data management and decision-making. These applications typically use smart contracts – self-executing contracts with the terms of agreement directly written into code.
2. Advantages of Developing DApps with Dart:
Dart brings several advantages to the table when it comes to developing decentralized applications for the blockchain:
2.1 High Performance:
Dart’s Just-In-Time (JIT) and Ahead-Of-Time (AOT) compilation provide high-performance execution, crucial for complex blockchain transactions and smart contract processing.
2.2 Strong Typing and Safety:
Dart’s strong typing system helps identify errors during the development phase, reducing potential bugs in smart contracts, and enhancing security.
2.3 Robust Developer Tools:
Dart comes with a powerful set of developer tools like Dart DevTools, which streamline the debugging and testing process, making it easier to identify and fix issues.
2.4 Flutter Integration:
With Flutter, Dart’s UI toolkit, developers can create seamless cross-platform DApps with rich user interfaces, increasing accessibility and usability.
3. Setting Up the Development Environment:
To start building DApps with Dart, you need to set up your development environment. Here are the steps:
3.1 Install Dart SDK:
Download and install the latest Dart SDK from the official Dart website. Ensure that you have the Dart command-line tools available in your terminal.
3.2 Choose a Blockchain Platform:
Select a blockchain platform that supports smart contracts written in Dart. Ethereum is a popular choice, and there are frameworks like Moralis and Flutterflow that provide the necessary tools for Dart-based Ethereum development.
3.3 Code Editor:
Use a code editor of your choice that supports Dart, such as Visual Studio Code or IntelliJ IDEA, and install the Dart extension.
4. Building Your First Smart Contract:
Let’s now create a simple smart contract using Dart and Solidity, the language typically used for Ethereum smart contracts. For this example, we’ll deploy it on the Ethereum blockchain using the Moralis framework.
4.1 Install Moralis:
First, install Moralis by running the following command:
bash npm install -g moralis-admin-cli
4.2 Create the Smart Contract:
Next, create a new Dart file for the smart contract, say hello_contract.dart. Here’s a basic template:
dart import 'package:moralis/moralis.dart'; class HelloContract { static const String contractAddress = "CONTRACT_ADDRESS"; static Future<String> getMessage() async { final result = await Moralis.executeFunction(contractAddress, "getMessage"); return result['result']; } static Future<void> setMessage(String message) async { await Moralis.executeFunction(contractAddress, "setMessage", params: { 'message': message, }); } }
4.3 Deploying the Smart Contract:
To deploy the contract on the Ethereum blockchain, you’ll need the contract’s bytecode and ABI. Compile the Solidity smart contract and retrieve the necessary details.
4.4 Interacting with the Smart Contract:
Now, let’s interact with the deployed contract using the Dart smart contract wrapper we created earlier:
dart void main() async { await Moralis.initialize("YOUR_APP_ID", "YOUR_SERVER_URL"); // Interact with the smart contract final message = await HelloContract.getMessage(); print("Message from the contract: $message"); await HelloContract.setMessage("Hello, blockchain!"); print("Message set successfully!"); }
5. Implementing Blockchain Transactions:
In a blockchain application, transactions are essential for interacting with smart contracts and updating the blockchain’s state. Dart provides excellent support for handling blockchain transactions.
5.1 Sending Transactions:
Using the Moralis framework, you can easily send transactions to the Ethereum blockchain:
dart import 'package:moralis/moralis.dart'; void main() async { await Moralis.initialize("YOUR_APP_ID", "YOUR_SERVER_URL"); final fromAddress = "SENDER_ADDRESS"; final toAddress = "RECEIVER_ADDRESS"; final amount = BigInt.from(1000000000000000000); // 1 Ether final transaction = Moralis.TransactionParams( to: toAddress, value: amount, ); final signedTransaction = await Moralis.signTransaction(transaction, fromAddress); final txHash = await Moralis.sendSignedTransaction(signedTransaction); print("Transaction Hash: $txHash"); }
6. Integrating Flutter for DApp UI:
To enhance the user experience, you can integrate Flutter for the DApp’s UI development. Flutter’s rich widget library and hot reload feature make it a powerful choice.
6.1 Setting Up Flutter:
Create a new Flutter project and configure the necessary dependencies in the pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter moralis_flutter: ^0.1.0
6.2 Building the DApp UI:
Design the DApp’s UI using Flutter widgets and connect it with your Dart smart contract wrapper to display data from the blockchain.
7. Testing and Deployment:
Once you have developed your DApp, perform rigorous testing to ensure its reliability and security. Consider deploying your smart contract on a testnet before going live on the mainnet.
Conclusion
Developing decentralized applications with Dart brings together the power of a robust programming language and the potential of blockchain technology. Dart’s versatility, strong typing, and Flutter integration provide a conducive environment for creating seamless and secure DApps. As blockchain technology continues to evolve, Dart’s capabilities will enable developers to build innovative and transformative applications that leverage the decentralized power of blockchain networks. So, start exploring the world of DApp development with Dart and be part of the blockchain revolution!
Table of Contents
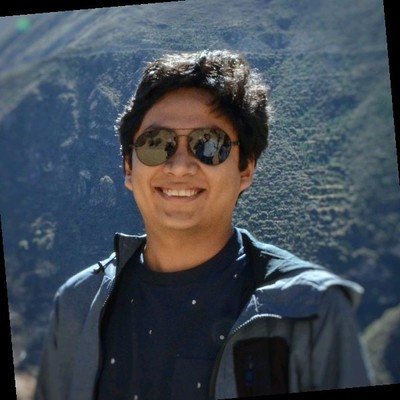
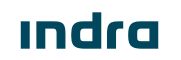