Reactive Programming with Dart: Building Responsive Applications
In the ever-evolving landscape of software development, building applications that are responsive and provide a seamless user experience is of utmost importance. Reactive programming has emerged as a powerful paradigm to achieve this goal. In this blog, we will delve into the world of reactive programming with Dart and discover how it enables us to build highly responsive applications. We’ll explore the principles, benefits, and dive into code examples that demonstrate how to harness the potential of reactive programming in Dart.
Table of Contents
1. Understanding Reactive Programming
Reactive programming is an approach to programming where applications are built by expressing the flow of data and the propagation of changes. In traditional imperative programming, you would explicitly define the sequence of steps to achieve a task. In reactive programming, you define how data changes over time, and the application automatically updates itself based on these changes.
2. The Principles of Reactive Programming
Reactive programming revolves around a few key principles:
- Data Streams: Reactive programming is centered around the concept of data streams. These streams represent sequences of events or changes in data over time. Streams can emit values, errors, and completion events.
- Observer Pattern: The observer pattern is at the heart of reactive programming. Observers (subscribers) subscribe to data streams and receive notifications whenever new data is emitted. This allows for a decoupled and efficient way of handling data changes.
- Functional Programming: Reactive programming encourages the use of functional programming concepts. Transformations, filtering, and combining of streams are often achieved using functions, making the code concise and expressive.
3. Benefits of Reactive Programming
Reactive programming offers several benefits when building responsive applications:
- Responsiveness: Reactive applications react to changes in data in real-time, providing a smooth and responsive user experience. This is crucial for interactive and dynamic applications.
- Modularity: The use of data streams and the observer pattern promotes modularity. Different parts of the application can be developed independently and seamlessly integrated using streams.
- Readability: The declarative nature of reactive programming makes the code more readable and understandable. Developers can focus on expressing the logic of the application rather than managing state and UI updates.
- Error Handling: Reactive programming provides elegant error handling mechanisms. Errors can be propagated through the stream, allowing for consistent error handling across the application.
4. Getting Started with Reactive Programming in Dart
To get started with reactive programming in Dart, you’ll need to understand the core concepts of working with streams. Dart provides a powerful Stream API that makes it easy to work with data streams. Let’s take a look at some fundamental concepts and code examples.
4.1. Creating Streams
In Dart, you can create a stream using the Stream class. Here’s an example of creating a simple stream of integers:
dart import 'dart:async'; void main() { var stream = Stream<int>.fromIterable([1, 2, 3, 4, 5]); stream.listen((value) { print(value); }); }
In this example, we create a stream of integers using the Stream.fromIterable constructor and then listen to the stream using the listen method. Whenever a new value is emitted from the stream, the provided callback is executed.
4.2. Transforming Streams
Reactive programming shines when you need to transform, filter, or combine streams. Dart’s Stream API provides methods like map, where, and combineLatest for these purposes.
dart import 'dart:async'; void main() { var stream = Stream<int>.fromIterable([1, 2, 3, 4, 5]); var doubledStream = stream.map((value) => value * 2); doubledStream.listen((value) { print(value); }); }
In this example, we use the map method to transform the original stream of integers into a new stream where each value is doubled.
4.3. Combining Streams
Combining streams allows you to synchronize multiple streams and react to events from multiple sources. The combineLatest function takes two or more streams and combines their latest values into a new stream.
dart import 'dart:async'; void main() { var stream1 = Stream<int>.fromIterable([1, 2, 3]); var stream2 = Stream<int>.fromIterable([10, 20, 30]); var combinedStream = Stream<int>.combineLatest([stream1, stream2], (values) { return values[0] + values[1]; }); combinedStream.listen((value) { print(value); }); }
In this example, we combine two streams by adding their latest values together using the combineLatest function.
5. Building a Responsive Dart Application
Now that we have a grasp of the fundamentals of reactive programming in Dart, let’s see how we can use it to build a responsive application. Imagine you’re building a simple counter application where the UI updates in real-time as the user interacts with it.
5.1. Creating a Reactive Counter
dart import 'dart:async'; void main() { var counterStreamController = StreamController<int>(); var counterStream = counterStreamController.stream; int counter = 0; counterStream.listen((value) { print('Counter: $value'); }); // Simulate user interactions for (int i = 1; i <= 5; i++) { counterStreamController.add(i); } counterStreamController.close(); }
In this example, we create a StreamController to manage our counter stream. We simulate user interactions by adding values to the stream, and the UI updates in real-time as the stream emits new values.
5.2. Integrating with UI
To create a truly responsive application, you would integrate the reactive counter with a user interface framework, such as Flutter. Let’s consider a basic Flutter example:
dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { final StreamController<int> _counterStreamController = StreamController<int>(); final Stream<int> _counterStream; MyApp() : _counterStream = _counterStreamController.stream; @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Reactive Counter'), ), body: Center( child: StreamBuilder<int>( stream: _counterStream, builder: (context, snapshot) { if (snapshot.hasData) { return Text( 'Counter: ${snapshot.data}', style: TextStyle(fontSize: 24), ); } else { return CircularProgressIndicator(); } }, ), ), floatingActionButton: FloatingActionButton( onPressed: () { _counterStreamController.add(1); }, child: Icon(Icons.add), ), ), ); } }
In this Flutter example, the UI reacts to changes in the counter stream. The StreamBuilder widget listens to the stream and updates the UI accordingly. When the user taps the floating action button, the counter value is incremented, and the UI updates in response.
Conclusion
Reactive programming with Dart offers a powerful way to build responsive applications that adapt to changing data in real-time. By embracing the principles of data streams, the observer pattern, and functional programming, developers can create applications with enhanced modularity, readability, and error handling. Dart’s Stream API provides the necessary tools to work with streams seamlessly. As demonstrated in the example, integrating reactive programming with UI frameworks like Flutter results in dynamic and interactive applications that provide an exceptional user experience. So, whether you’re building a simple counter app or a complex application, consider harnessing the power of reactive programming in Dart to create applications that truly respond to the user’s needs.
Table of Contents
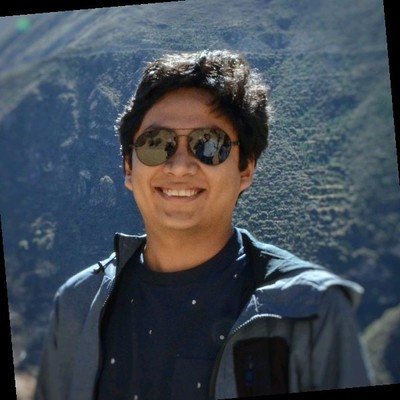
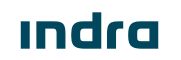