Building RESTful APIs with Dart: A Complete Guide
In today’s digital landscape, creating efficient and scalable APIs is crucial for web and mobile application development. RESTful APIs have become the standard for designing web services due to their simplicity and versatility. If you’re looking to build RESTful APIs using the Dart programming language, you’re in the right place. This comprehensive guide will take you through the process step by step, from setting up your environment to implementing advanced features.
Table of Contents
1. Introduction to RESTful APIs and Dart
1.1. Understanding REST Architecture
REST (Representational State Transfer) is an architectural style that defines a set of constraints for designing networked applications. RESTful APIs use HTTP methods to perform CRUD (Create, Read, Update, Delete) operations on resources. These APIs are stateless, meaning each request from a client to the server must contain all the information necessary for the server to understand and fulfill the request. Dart, a versatile programming language developed by Google, is an excellent choice for building RESTful APIs due to its performance and asynchronous programming capabilities.
1.2. Why Choose Dart for API Development
Dart’s strong typing, modern syntax, and support for asynchronous programming make it a suitable language for API development. It provides an easy-to-use environment for creating HTTP servers, handling requests, and responding with JSON data. Dart’s single-threaded event loop model and built-in concurrency support ensure that your APIs are responsive and can handle a large number of concurrent requests efficiently.
2. Setting Up Your Development Environment
2.1. Installing Dart SDK
To get started with Dart development, you need to install the Dart SDK (Software Development Kit). The SDK includes the Dart programming language, libraries, and tools necessary for building Dart applications. You can download the SDK from the official Dart website and follow the installation instructions for your operating system.
2.2. Choosing an IDE
While Dart code can be written using a simple text editor, using an Integrated Development Environment (IDE) can significantly enhance your productivity. Some popular choices for Dart development include Visual Studio Code (VS Code) with the Dart and Flutter extensions, IntelliJ IDEA with the Dart plugin, and Android Studio with the Flutter plugin.
2.3. Creating a New Dart Project
Once you have Dart and your preferred IDE set up, you can create a new Dart project. Use the Dart command-line tool to generate a new project structure with the following command:
bash dart create my_api_project
This command will create a new directory named “my_api_project” containing the basic structure of a Dart project.
3. Building Your First Dart RESTful API
3.1. Creating Routes and Endpoints
In Dart, you can use the shelf package to create HTTP servers and handle requests. Shelf provides a modular approach to building web applications and APIs. To create a basic API endpoint, follow these steps:
- Add the shelf package to your project’s pubspec.yaml file:
yaml dependencies: shelf: ^1.0.0
- Import the necessary libraries in your Dart code:
dart import 'package:shelf/shelf.dart'; import 'package:shelf/shelf_io.dart' as shelf_io;
- Define a handler function for your API endpoint:
dart Response _handleHelloRequest(Request request) { return Response.ok('Hello, Dart API!'); }
- Create a shelf handler using the defined function:
dart var handler = Pipeline() .addMiddleware(logRequests()) .addHandler(_handleHelloRequest); Start the HTTP server: dart Copy code void main() { var server = await shelf_io.serve(handler, 'localhost', 8080); print('Server running on localhost:${server.port}'); }
With these steps, you’ve created a basic Dart API that responds with “Hello, Dart API!” when accessed.
3.2. Handling HTTP Methods (GET, POST, PUT, DELETE)
RESTful APIs support various HTTP methods to perform different actions on resources. Shelf allows you to handle these methods using the Request object’s method property. Here’s an example of handling a GET request:
dart Response _handleGetRequest(Request request) { return Response.ok('This is a GET request.'); }
Similarly, you can define handler functions for POST, PUT, and DELETE requests.
3.3. Sending and Receiving JSON Data
RESTful APIs commonly exchange data in JSON format. To send and receive JSON data in your Dart API, you can use the dart:convert library, which provides classes for working with JSON data. Here’s how you can parse JSON data from a request and send a JSON response:
dart import 'dart:convert'; Response _handleJsonRequest(Request request) { final requestBody = await request.readAsString(); final requestData = jsonDecode(requestBody); final responseData = {'message': 'Received JSON data', 'data': requestData}; return Response.ok(jsonEncode(responseData), headers: {'content-type': 'application/json'}); }
In this example, the jsonDecode function is used to parse the JSON data from the request, and the jsonEncode function is used to convert the response data to JSON format.
4. Structuring Your Dart API Project
4.1. Separation of Concerns
When building RESTful APIs, it’s important to follow the principles of separation of concerns. Divide your code into different modules or files to keep your codebase organized and maintainable. For example, you can create separate files for route handlers, data models, middleware, and utility functions.
4.2. Using Packages and Dependencies
Dart provides a package manager called pub that allows you to manage external dependencies in your projects. You can specify your project’s dependencies in the pubspec.yaml file and use the pub get command to fetch and install them. Using packages can save you time and effort by leveraging existing solutions for common tasks.
4.3. Implementing Data Models
Data models define the structure of the data you’ll be working with in your API. Dart is a statically typed language, so you can create classes to represent different types of data. For example, if you’re building an API for managing tasks, you could define a Task class like this:
dart class Task { final String id; final String title; final bool completed; Task(this.id, this.title, this.completed); }
By creating data models, you can ensure consistency in the structure of your API’s data.
5. Error Handling and Validation
5.1. Handling Errors and Exceptions
Error handling is a critical aspect of building robust APIs. Dart provides mechanisms to handle exceptions and errors gracefully. You can use try and catch blocks to handle exceptions that might occur during API operations. Additionally, you can define custom exception classes to represent specific error scenarios and provide meaningful error messages to clients.
5.2. Input Data Validation
Validating user input is essential to ensure the integrity of your data and prevent malicious actions. Dart offers libraries like validate and quiver that provide validation functions for common scenarios. You can validate data before processing it and return appropriate error responses if validation fails.
5.3. Providing Clear Error Responses
When errors occur, it’s important to provide clear and informative error responses to clients. Your API should return standardized error formats with relevant error codes and descriptions. Creating a consistent error-handling strategy improves the user experience and helps developers understand and troubleshoot issues.
6. Authentication and Security
6.1. Implementing User Authentication
Securing your API is crucial to protect sensitive data and ensure that only authorized users can access certain resources. Dart offers various authentication mechanisms, including API keys, OAuth, and JWT (JSON Web Tokens). You can choose the authentication method that best fits your project’s requirements.
6.2. Token-Based Authentication
Token-based authentication, particularly JWT, is a popular choice for securing RESTful APIs. With JWT, a token is generated when a user logs in and is included in subsequent requests to authenticate the user. The server can decode and verify the token to grant or deny access.
6.3. Securing Routes and Endpoints
Not all routes and endpoints in your API should be accessible to the public. You can use middleware to implement access control and restrict certain routes to authenticated users. By checking the authentication status of incoming requests, you can ensure that only authorized users can perform certain actions.
7. Advanced Features and Best Practices
7.1. Pagination and Data Limiting
For APIs that return large amounts of data, implementing pagination can enhance performance and user experience. Pagination involves dividing the data into smaller chunks (pages) and providing links or metadata to navigate through the pages. Additionally, you can implement query parameters to allow clients to limit the amount of data returned.
7.2. Caching Strategies
Caching is a technique used to store and reuse previously fetched data, reducing the need for repeated requests to the server. Implementing caching in your Dart API can significantly improve response times and reduce server load. You can use HTTP caching headers and libraries like shelf_cache to manage caching.
7.3. Rate Limiting for API Protection
To prevent abuse and ensure fair usage, consider implementing rate limiting for your API. Rate limiting restricts the number of requests a client can make within a certain time period. This helps maintain the overall performance of your API and prevents single clients from overwhelming the server.
8. Testing Your Dart API
8.1. Unit Testing and Integration Testing
Testing is a crucial part of software development, and APIs are no exception. Dart provides testing frameworks like test and shelf_test that allow you to write unit tests and integration tests for your API endpoints. Unit tests focus on testing individual components, while integration tests simulate the interaction between different parts of your API.
8.2. Using Testing Libraries
Testing libraries offer tools and utilities to simplify the process of writing and running tests. Dart’s testing libraries provide assertions, mock objects, and test runners to help you verify the correctness of your API’s functionality.
8.3. Automating Testing Processes
To ensure the reliability of your API, consider automating the testing process. Continuous Integration (CI) tools like Travis CI, CircleCI, or GitHub Actions can automatically run tests whenever you push changes to your code repository. This ensures that your API remains functional as you make updates.
9. Documenting Your API
9.1. Importance of API Documentation
Clear and comprehensive documentation is essential for developers who will be using your API. Documentation provides information about available endpoints, request and response formats, authentication methods, error handling, and usage examples. Well-documented APIs are easier to understand and integrate into projects.
9.2. Using Tools like Swagger/OpenAPI
Swagger (now known as the OpenAPI Specification) is a powerful tool for documenting RESTful APIs. It allows you to describe your API’s structure, endpoints, parameters, and responses in a machine-readable format. With the help of various tools and libraries, you can generate interactive API documentation that makes it easy for developers to explore and understand your API.
9.3. Keeping Documentation Up to Date
APIs evolve over time, and so should their documentation. Whenever you make changes to your API, remember to update the documentation accordingly. Outdated or inaccurate documentation can lead to confusion and errors for developers using your API.
10. Deployment and Hosting
10.1. Choosing a Deployment Platform
Once your Dart API is ready for production, you need to choose a deployment platform. Popular options include traditional web servers like Apache or Nginx, as well as specialized platforms like Google Cloud, Amazon Web Services (AWS), or Heroku. Each platform has its own benefits and considerations, so choose one that aligns with your project’s requirements.
10.2. Containerization with Docker
Docker is a containerization platform that allows you to package your application and its dependencies into a portable container. Containerization ensures consistency between development and production environments, making deployment smoother and more reliable. You can create a Dockerfile to define the environment and configuration for your Dart API and then use Docker to build and run the container.
10.3. Deploying Dart APIs to the Cloud
Cloud platforms offer scalability, high availability, and ease of management for deploying APIs. Services like Google Cloud Functions, AWS Lambda, or Firebase Cloud Functions allow you to deploy serverless Dart APIs, where the platform automatically handles scaling and resource management based on demand.
Conclusion
Building RESTful APIs with Dart opens up a world of possibilities for creating powerful and performant web services. This complete guide has covered everything from the basics of setting up your development environment to implementing advanced features like authentication, security, testing, and documentation. By following these steps and best practices, you’ll be well-equipped to develop robust and efficient APIs that can serve as the backbone of your web and mobile applications. So, harness the capabilities of Dart and start building your RESTful APIs today!
Table of Contents
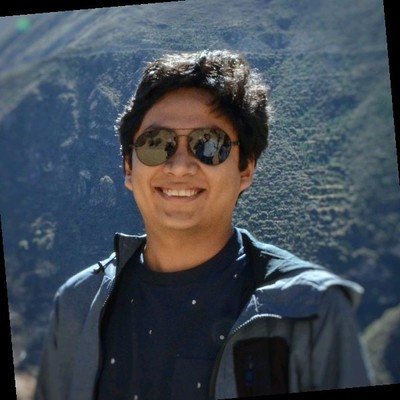
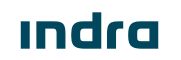