Exploring Dart’s Built-in Libraries: Saving Time and Effort
As a developer, time and effort are valuable resources. Anything that can streamline the development process and make coding more efficient is highly sought after. Dart, a powerful and flexible programming language, comes with an array of built-in libraries that can help you achieve just that. In this blog, we’ll delve into some of Dart’s essential built-in libraries, explore their functionalities, and provide code samples to showcase their power and simplicity.
1. Introduction to Dart’s Built-in Libraries
Dart is designed to be a versatile language that is easy to learn and work with. It comes with an extensive standard library, or built-in libraries, which are included by default in any Dart project. These libraries cover a wide range of functionalities, making it easier for developers to accomplish various tasks without the need for third-party dependencies.
2. Working with dart:core Library
The dart:core library is automatically imported into every Dart project and provides fundamental utilities and classes that form the foundation of the language. Let’s explore some of the essential elements in this library.
2.1 Handling Collections with List and Map
Collections are an integral part of any programming language, and Dart offers the List and Map classes within dart:core to work with ordered and key-value collections, respectively.
dart void main() { // List example List<int> numbers = [1, 2, 3, 4, 5]; print(numbers[2]); // Output: 3 // Map example Map<String, dynamic> person = { 'name': 'John Doe', 'age': 30, 'isDeveloper': true, }; print(person['name']); // Output: John Doe }
2.2 String Manipulation with String
The String class in dart:core provides various methods to manipulate strings efficiently.
dart void main() { String greeting = 'Hello, Dart!'; print(greeting.length); // Output: 12 print(greeting.toUpperCase()); // Output: HELLO, DART! print(greeting.contains('Dart')); // Output: true }
2.3 Date and Time with DateTime
The DateTime class helps with date and time manipulation, making it easier to work with time-related operations.
dart void main() { DateTime now = DateTime.now(); print(now); // Output: 2023-07-17 12:34:56.789 DateTime futureDate = now.add(Duration(days: 7)); print(futureDate); // Output: 2023-07-24 12:34:56.789 }
3. Simplify Asynchronous Programming with dart:async
Asynchronous programming is essential for building responsive applications. The dart:async library provides classes for working with asynchronous operations, including Futures and Streams.
3.1 Futures and Async/Await
Futures represent a potential value or error that will be available at some time in the future. By using async and await keywords, working with Futures becomes much more straightforward and readable.
dart Future<void> fetchData() async { try { final response = await http.get('https://api.example.com/data'); // Process the response } catch (e) { print('Error: $e'); } }
3.2 Streams for Reactive Programming
Streams allow handling sequences of events or data in a reactive manner. They are useful for scenarios like real-time updates and event-driven architectures.
dart void main() { final streamController = StreamController<int>(); final stream = streamController.stream; stream.listen((data) { print('Received data: $data'); }); streamController.add(1); // Output: Received data: 1 streamController.add(2); // Output: Received data: 2 }
4. Building User Interfaces with dart:ui
Dart’s dart:ui library provides essential tools for creating custom user interfaces and working with graphics.
4.1 Custom Drawing with Canvas
The Canvas class enables drawing shapes, paths, and text, making it ideal for custom graphics.
dart void draw(Canvas canvas, Size size) { final paint = Paint()..color = Colors.blue; canvas.drawRect(Rect.fromLTWH(0, 0, 50, 50), paint); }
4.2 Gesture Detection
The dart:ui library also supports gesture detection, allowing you to make your user interface interactive.
dart void handleTap() { // Handle tap gesture } // Inside the Widget build method GestureDetector( onTap: handleTap, child: Container( width: 100, height: 100, color: Colors.red, ), )
5. Networking and HTTP Requests using dart:io
When it comes to networking and making HTTP requests, Dart’s dart:io library comes to the rescue.
5.1 Making HTTP Requests
Performing HTTP GET or POST requests becomes effortless with the http package.
dart import 'package:http/http.dart' as http; void fetchData() async { final response = await http.get('https://api.example.com/data'); if (response.statusCode == 200) { // Handle the response data } else { print('Error: ${response.statusCode}'); } }
5.2 Server-side Development
dart:io is also handy for server-side development, allowing you to build web servers with ease.
dart import 'dart:io'; void main() async { final server = await HttpServer.bind(InternetAddress.anyIPv4, 8080); print('Server running on port 8080'); await for (HttpRequest request in server) { request.response.write('Hello, world!'); await request.response.close(); } }
6. File System Operations with dart:io
For file system-related tasks, the dart:io library offers convenient classes and methods.
6.1 Reading and Writing Files
Reading and writing files is straightforward using File class methods.
dart import 'dart:io'; void writeToFile(String data) { final file = File('data.txt'); file.writeAsString(data); } void readFromFile() { final file = File('data.txt'); if (file.existsSync()) { final contents = file.readAsStringSync(); print(contents); } }
6.2 Directory Manipulation
Working with directories is also a breeze with Directory class methods.
dart import 'dart:io'; void createDirectory() { final directory = Directory('my_directory'); directory.createSync(); } void listFilesInDirectory() { final directory = Directory('my_directory'); final files = directory.listSync(); for (var file in files) { print(file.path); } }
7. Simplified Testing with dart:test
Writing and running tests is essential for ensuring the quality of your code. Dart provides the dart:test library for easy and effective testing.
7.1 Writing Unit Tests
Let’s see an example of a simple unit test:
dart import 'package:test/test.dart'; int addNumbers(int a, int b) { return a + b; } void main() { test('Addition Test', () { expect(addNumbers(2, 3), 5); }); }
7.2 Running Tests
Running tests is a straightforward process using the test package.
bash pub run test
8. Internationalization with dart:intl
When developing applications for a global audience, supporting internationalization is crucial. The dart:intl library helps with formatting dates, numbers, and messages in various languages.
dart import 'package:intl/intl.dart'; void main() { final price = 1000; final formattedPrice = NumberFormat.currency(locale: 'en_US', symbol: '$').format(price); print(formattedPrice); // Output: $1,000.00 }
Conclusion
Dart’s built-in libraries offer a vast array of functionalities that can significantly simplify your development process. From handling collections and asynchronous programming to networking, file system operations, and internationalization, these libraries cover a wide range of use cases, saving you time and effort in your projects. As you explore these libraries further and integrate them into your applications, you’ll appreciate their power and convenience, making Dart a language of choice for efficient and effective development. Happy coding!
Table of Contents
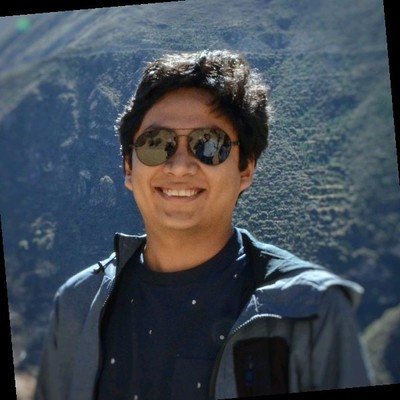
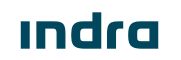